Best Way to Structure a Div
Aug 6, 2024
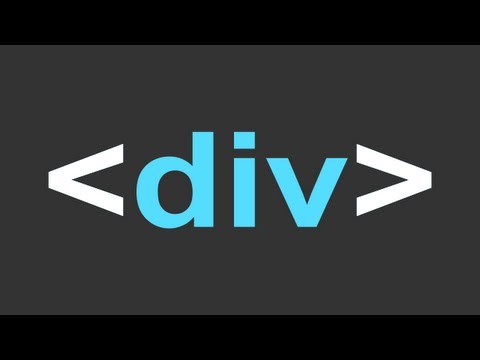
When it comes to web development, the <div>
tag is one of the most essential elements in HTML. It serves as a versatile container that allows developers to group content and apply styles through CSS. In this blog post, we will explore the best practices for structuring a <div>
, focusing on positioning using CSS and the various CSS position properties that can be utilized to achieve desired layouts.
Understanding the <div>
Tag
The <div>
tag is a block-level element
that does not inherently convey any meaning about its content. It is primarily used for styling and layout purposes. By grouping elements within a <div>
, developers can apply CSS styles uniformly, making it easier to manage and manipulate web page layouts.
When to Use the <div>
Tag
Grouping Related Content: Use
<div>
to group similar content, such as text, images, and other elements that share a common purpose.Styling with CSS: Apply CSS styles to a
<div>
to control the appearance of the grouped content.Layout Control: Utilize
<div>
tags to create complex layouts by nesting them and applying different styles.
Best Practices for Structuring a <div>
To effectively structure a <div>
, consider the following best practices:
Use Semantic HTML: While
<div>
tags are useful, prefer semantic HTML elements (like<header>
,<footer>
,<section>
, etc.) when appropriate for better accessibility and SEO.Assign Classes and IDs: Use classes and IDs to target specific
<div>
elements with CSS. This practice enhances maintainability and readability.Limit Nesting: Avoid excessive nesting of
<div>
tags, which can lead to complicated structures that are hard to manage.Keep It Clean: Ensure your HTML is well-organized and readable. Use indentation and spacing to improve clarity.
Positioning Using CSS
The CSS position
property is crucial for controlling the layout of <div>
elements. It defines how an element is positioned in the document. There are several values for the position
property:
static: This is the default positioning. The element is positioned according to the normal flow of the document.
relative: The element is positioned relative to its normal position. Use this to adjust the position without affecting surrounding elements.
absolute: The element is positioned relative to the nearest positioned ancestor (not static). It is removed from the normal document flow.
fixed: The element is positioned relative to the viewport, meaning it stays in the same place even when the page is scrolled.
sticky: This is a hybrid between relative and fixed positioning, toggling based on the user's scroll position.
Example of Positioning Using CSS
Here’s how you can use different positioning methods with <div>
elements:
Practical Examples of Using <div>
Tags
Example 1: Basic Layout
This example demonstrates a simple layout using<div>
tags for the header, content area, and footer.
Example 2: Flexbox Layout
Using Flexbox is another effective way to structure<div>
elements. Here’s how to create a responsive layout:
Conclusion
Structuring a<div>
effectively is essential for creating well-organized and visually appealing web pages. By understanding the variousCSS positionproperties and employing best practices, developers can create flexible layouts that enhance user experience. Remember to use semantic HTML where possible, and leverage the power of CSS to style and position your<div>
elements appropriately.By mastering these techniques, you can elevate your web development skills and create layouts that are not only functional but also aesthetically pleasing.
Happy coding!