Button Click on Autofill Triggers Submit: A Comprehensive Guide
Aug 17, 2024
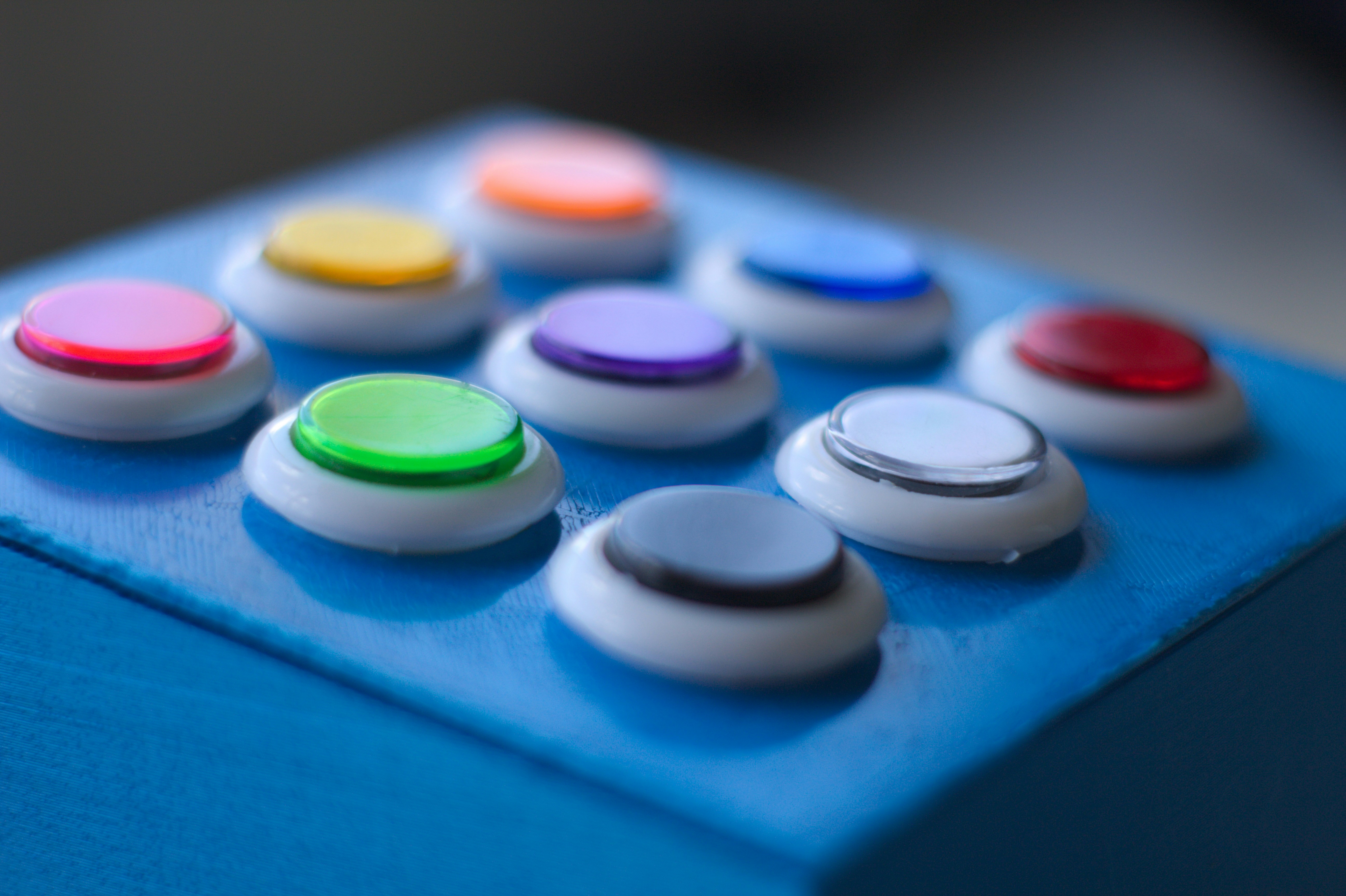
In modern web development, user experience is paramount. One common interaction that can enhance usability is when a button click on an autofill form triggers a submit action. This functionality is particularly useful in scenarios where users expect seamless interactions, such as in registration forms, payment gateways, or any data entry forms. In this blog post, we will explore the mechanics behind this interaction, provide code snippets, and discuss best practices for implementation.
Understanding Autofill and Form Submission
Autofill is a feature that automatically fills in form fields based on previously entered data, making it easier for users to complete forms without having to type everything out. When combined with JavaScript, we can enhance this feature by ensuring that a button click triggers the form submission, even if the user does not explicitly click the submit button.
Why Use Autofill?
Improved User Experience: Autofill saves time and reduces the chance of user error.
Increased Conversion Rates: Simplifying the form-filling process can lead to higher submission rates.
Accessibility: Helps users with disabilities who may find typing challenging.
Implementing Button Click on Autofill Triggers Submit
To achieve the desired functionality, we will use a combination of HTML, CSS, and JavaScript. Below is a step-by-step guide with code snippets.
Step 1: Create the HTML Form
Here’s a simple HTML form that includes autofill functionality.
In this form, we have three fields: username, email, and password. The autocomplete
attribute is set to suggest autofill options based on the user's previous entries.
Step 2: Add JavaScript for Button Click Functionality
Next, we need to add JavaScript to handle the button click event. When the button is clicked, we will check if the form fields are filled and then submit the form.
This script listens for a click event on the button. It checks if the form is valid (i.e., all required fields are filled) and submits the form if it is. If not, it alerts the user to complete the required fields.
Best Practices for Implementing Autofill and Submit Functionality
Use Semantic HTML: Ensure that your form elements are properly labeled. This improves accessibility and ensures that screen readers can correctly interpret the form.
Validate Input: Always validate user input both on the client-side and server-side to prevent errors and security vulnerabilities.
Provide Feedback: If a user attempts to submit a form with missing fields, provide clear feedback on what needs to be corrected.
Test Across Browsers: Autofill behavior can vary between browsers. Test your implementation in different environments to ensure consistency.
Consider Security: Be cautious with sensitive information, especially when using autofill for passwords or personal data. Always use HTTPS to secure data transmission.
Enhancing User Experience with CSS
To make your form visually appealing, you can add some CSS styles. Here’s a simple example:
This CSS will style the form, making it more user-friendly and visually appealing.
Conclusion
Implementing a button click on autofill triggers submit functionality can significantly enhance the user experience on your website. By following the steps outlined in this guide, you can create a seamless interaction that encourages users to complete forms quickly and efficiently.