Constant Speed Carousel
Aug 19, 2024
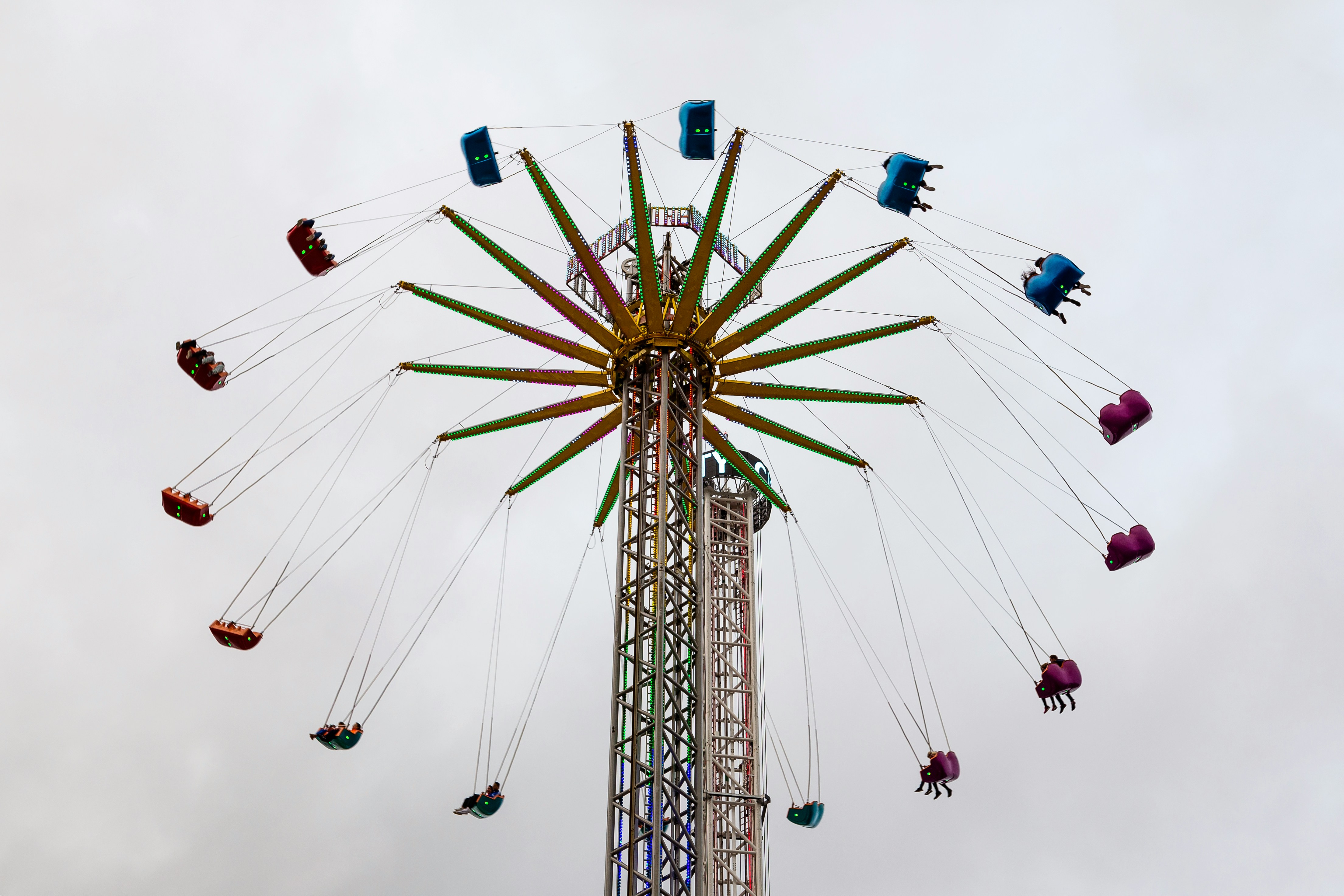
Understanding Constant Speed Carousels
A constant speed carousel is a mechanical system that rotates around a central axis at a consistent speed. These systems are widely used in various applications, from amusement park rides to industrial machinery. Understanding the principles behind constant speed carousels can help in designing and programming these systems efficiently.
The Mechanics of a Constant Speed Carousel
At its core, a constant speed carousel operates based on principles of rotational motion. The key components include:
Motor: Provides the necessary torque to maintain a constant speed.
Gear System: Transmits power from the motor to the carousel platform.
Platform: The surface on which objects or passengers are placed.
Control System: Manages the speed and direction of the carousel.
Key Equations
The motion of a constant speed carousel can be described using the following equations:
Angular Velocity (ωω):
ω=2πT
where TT is the period of rotation.
Centripetal Acceleration (acac):
ac=v2r
where vv is the linear speed and rr is the radius of the carousel.
Torque (ττ):
τ=I⋅α
where II is the moment of inertia and αα is the angular acceleration.
Implementing a Constant Speed Carousel in Code
To simulate a constant speed carousel, you can use programming languages such as Python. Below is a simple code snippet that demonstrates how to create a basic simulation of a constant speed carousel.
This code uses NumPy for numerical calculations and Matplotlib for plotting the carousel's circular motion over time. The speed
variable controls how fast the carousel rotates.
Applications of Constant Speed Carousels
Amusement Parks
In amusement parks, constant speed carousels provide a fun and safe experience for riders. The design ensures that the speed remains constant, providing a predictable and enjoyable ride.
Industrial Applications
In industrial settings, constant speed carousels are used for material handling and storage. They can efficiently transport goods in warehouses, reducing the need for manual labor.
Robotics
In robotics, constant speed carousels can be used in assembly lines where parts need to be placed at a consistent rate. Programming these systems requires an understanding of both mechanical and software engineering principles.
Programming Considerations
When programming a constant speed carousel, consider the following:
Motor Control: Implement PID (Proportional-Integral-Derivative) controllers to maintain constant speed despite load variations.
Safety Protocols: Ensure that emergency stops and safety sensors are integrated into the control system.
User Interface: Develop an intuitive interface for operators to control speed and monitor performance.
Example of Motor Control in Python
Here’s a simple example of how you might implement a basic motor control system for a carousel:
This code snippet creates a simple class for controlling the carousel motor, allowing you to set and run the motor at a constant speed.
Conclusion
A constant speed carousel is a fascinating topic that combines mechanical engineering, programming, and practical applications. By understanding the underlying principles and implementing effective control systems, you can design and program efficient carousels for various uses.
Incorporating the keyword "constant speed carousel" throughout the content not only helps in SEO optimization but also ensures that readers find the information relevant and engaging. By following best practices in coding and mechanical design, you can create a carousel that operates smoothly and reliably.