Creating a Body Animated HTML Background Image GIF
Aug 7, 2024
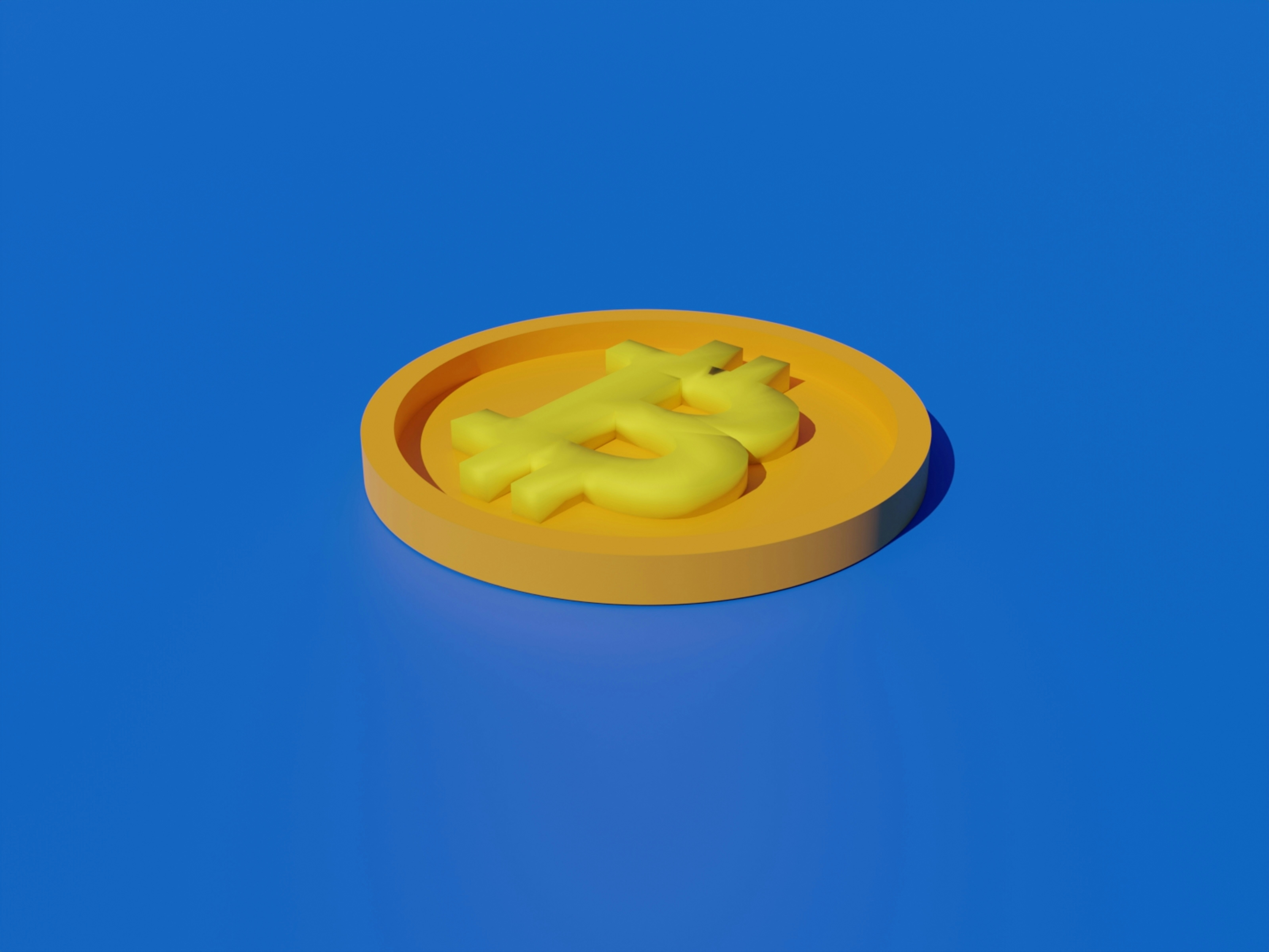
In the world of web design, animated backgrounds can significantly enhance the user experience by adding visual interest and engagement. This blog post will explore how to implement a body animated HTML background image GIF effectively, along with practical code snippets and techniques such as the Next.js mouse ripple effect.
Understanding GIF Backgrounds
GIFs (Graphics Interchange Format) are popular for their ability to display animations
. Using a GIF as a background can create a dynamic and lively atmosphere for your website. However, it’s essential to use them wisely to avoid overwhelming users or causing performance issues.
Advantages of Using GIFs as Backgrounds
Visual Appeal: Animated GIFs can capture attention and convey emotions or themes effectively.
Lightweight: Compared to video backgrounds, GIFs are often smaller in size, making them easier to load.
Compatibility: GIFs are widely supported across all modern browsers, ensuring a consistent experience for users.
Setting Up Your HTML Structure
To start, let’s set up a basic HTML structure. This will include a div
that will serve as our animated background.
CSS for Animated Background
Next, we’ll add CSS to style our background. The key properties to focus on arebackground-image
,background-size
, andbackground-position
.
Implementing the Next.js Mouse Ripple Effect
To enhance user interaction, we can add a mouse ripple effect using JavaScript and CSS. This effect will create a ripple animation wherever the user clicks on the page.
JavaScript for Mouse Ripple Effect
Here’s a simple JavaScript function to implement the ripple effect:
CSS for Ripple Effect
Now, let’s style the ripple effect with CSS:
Best Practices for Using GIFs as Backgrounds
Optimize GIF Size: Ensure your GIF is optimized for web use to prevent slow loading times. Tools like Tiny GIF can help reduce file size without significant quality loss.
Limit Animation Length: Long or complex animations can distract users. Keep animations short and loop them seamlessly.
Consider Accessibility: Ensure that text remains readable over the animated background. Use contrasting colors and consider adding a semi-transparent overlay if necessary.
Test Across Devices: Always test your animated backgrounds on various devices and screen sizes to ensure a consistent experience.
Conclusion
Using a body animated HTML background image GIF can significantly enhance the aesthetic appeal of your website. By following the guidelines and code snippets provided, you can create an engaging user experience. Additionally, incorporating features like theNext.js mouse ripple effect adds an interactive layer that can delight users.