Creating a Check box Circle Image
Aug 7, 2024
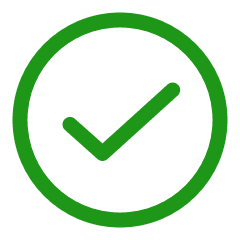
Checkboxes are an essential component of user interfaces, allowing users to make selections easily. In recent years, designers have experimented with various styles, including the checkbox circle image, which combines aesthetics with functionality. This blog post will explore the concept of checkbox circle images, their design implications, and provide code snippets to help you implement them effectively.
Understanding Checkbox Circle Images
Checkbox circle images are a modern take on traditional checkboxes, featuring a circular design that enhances visual appeal. This design choice can improve user experience by making selections more intuitive and visually distinct. Key Benefits of Checkbox Circle Images:
Aesthetic Appeal: Circular designs are often perceived as more modern and clean compared to traditional square checkboxes.
User Engagement: A visually appealing checkbox can engage users better, encouraging interaction.
Space Efficiency: Circular checkboxes can fit better in compact layouts, especially on mobile devices.
Design Considerations for Checkbox Circle Images
When designing checkbox circle images, it's crucial to consider usability and accessibility. Here are some design tips:
Contrast and Visibility: Ensure that the checkbox is easily visible against the background. Use contrasting colors for the checkbox and the checkmark.
Size Matters: The checkbox should be large enough to be easily clickable, especially on touch devices.
Feedback Mechanism: Provide clear feedback when the checkbox is checked or unchecked, such as changing colors or animations.
Implementing Checkbox Circle Images with HTML and CSS
Creating a checkbox circle image involves using HTML for structure and CSS for styling. Below is a step-by-step guide to implementing a custom checkbox circle image.
Step 1: HTML Structure
Start by creating the basic HTML structure for your checkbox:
This structure includes a label that wraps the checkbox input and a span for the custom checkmark.
Step 2: CSS Styling
Next, use CSS to style the checkbox and create the circular effect. Here’s an example:
This CSS code styles the checkbox to appear as a circle and includes hover and checked states. The border-radius
property is key to achieving the circular shape.
Enhancing Checkbox Circle Images with JavaScript
To improve user interaction, you can add JavaScript functionality. For instance, you might want to log the checkbox state or trigger other actions when the checkbox is checked or unchecked. Here’s a simple example:
This script adds an event listener to each checkbox, logging its state to the console whenever it is toggled.
Accessibility Considerations
When implementing checkbox circle images, accessibility is paramount. Ensure that your checkboxes are keyboard navigable and screen reader friendly. Here are some tips:
Use Labels: Always use
<label>
elements to associate text with checkboxes. This allows users to click the label to toggle the checkbox.ARIA Roles: Consider using ARIA roles and properties to enhance accessibility. For example, you can use
role="checkbox"
andaria-checked
attributes to indicate the state of the checkbox.
Common Use Cases for Checkbox Circle Images
Checkbox circle images can be used in various applications, including:
Forms: Enhance user experience in forms by using circular checkboxes for options like preferences or subscriptions.
To-Do Lists: Use circular checkboxes in task management applications to allow users to mark tasks as complete.
Surveys and Polls: Implement checkbox circle images in surveys to improve visual appeal and user engagement.
Conclusion
Checkbox circle images are a modern and effective way to enhance user interfaces. By combining aesthetic appeal with functionality, they can significantly improve user experience. Implementing these checkboxes requires a thoughtful approach to design, coding, and accessibility.