Drag and Drop a Textbox Field Example in Angular
Aug 17, 2024
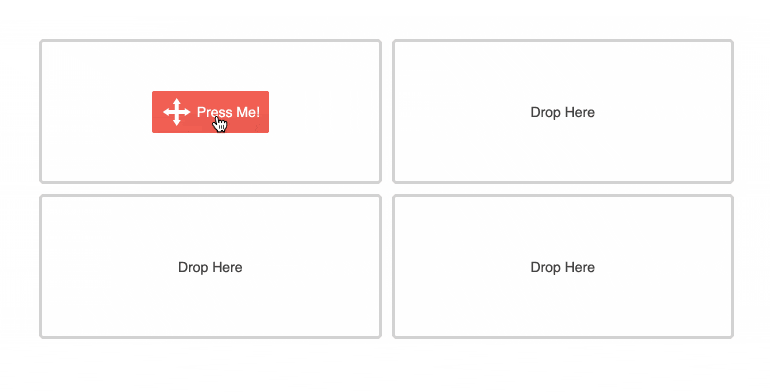
1. Introduction to Drag and Drop in Angular
Angular provides a powerful way to create dynamic web applications. One of its features is the ability to implement drag-and-drop functionality, which can be particularly useful in applications that require user input, such as forms. In this example, we will create a simple drag-and-drop textbox field that allows users to drag a textbox to a specific area on the screen.
2. Setting Up Your Angular Project
To get started, you need to have Angular CLI installed. If you haven't installed it yet, you can do so using the following command:
Next, create a new Angular project:
Once your project is set up, you can add Angular Material, which provides a set of reusable UI components, including drag-and-drop functionality:
3. Implementing Drag and Drop Functionality
3.1. HTML Structure
In yourapp.component.html
, set up the basic structure for the drag-and-drop textbox. You will use Angular Material's drag-and-drop module.
3.2. CSS Styles
Now, add some basic styles to yourstyles.css
orapp.component.css
to make the drag-and-drop area visually appealing.
3.3. TypeScript Logic
In yourapp.component.ts
, import the necessary modules and implement the logic for handling the drop event.
4. Testing the Drag and Drop Feature
Now that you have implemented the drag-and-drop functionality, it's time to test it. Run your Angular application using the following command:
Open your browser and navigate to http://localhost:4200
. You should see the drag-and-drop textbox. Try dragging it around the drop zone to see how it behaves.
Conclusion
In this blog post, you learned how to create a drag-and-drop textbox field example in Angular. This feature can significantly improve user interaction within your applications. By following the steps outlined above, you can easily implement this functionality in your own projects.