Exploring the Concept of Currying in JavaScript
Jul 26, 2024
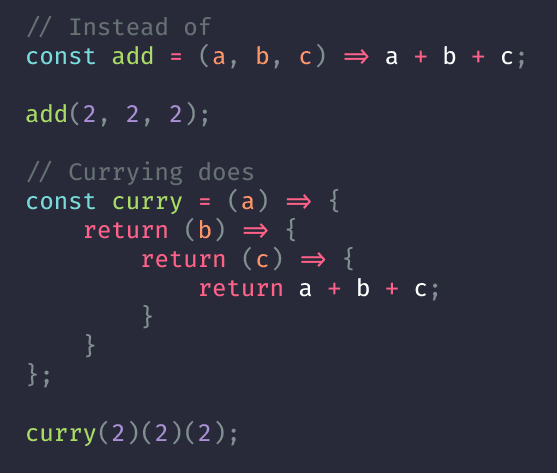
Currying is a powerful programming technique that transforms a function with multiple arguments into a sequence of functions with a single argument each. This transformation allows for more modular and composable code, making it easier to manage complex functions and avoid passing the same variable multiple times. In this blog post, we will delve into the concept of currying in JavaScript, providing examples and code snippets to illustrate its application.
What is Currying in JavaScript?
Currying is a functional programming technique that involves breaking down a function that takes multiple arguments into a series of functions that take one argument each. This process is named after Haskell Curry, a mathematician and logician who contributed to the development of functional programming.In JavaScript, currying is achieved by returning a new function that expects the next argument each time a function is called. This transformation allows for a function like f(a, b, c)
to be rewritten as f(a)(b)(c)
, where each function call returns a new function that expects the next argument.
Benefits of Currying in JavaScript
Currying offers several advantages in JavaScript:
Modular Code: By breaking down a function into smaller functions, currying helps in creating more modular code. This makes it easier to manage complex functions and reuse parts of the code.
Composability: Currying allows for the composition of functions, enabling the creation of higher-order functions. This is particularly useful in functional programming.
Avoiding Variable Passing: Currying avoids the need to pass the same variable multiple times, which can simplify code and reduce errors.
Improved Readability: The use of nested functions can improve the readability of code, as each function is focused on a specific task.
Implementing Currying in JavaScript
Currying can be implemented in JavaScript using various techniques. Here are a few examples:
Using the bind
Method
Using Closures
Using a Helper Function
Accessing Object Elements
Infinite Currying
Infinite currying is a concept that allows for the creation of an infinite chain of functions. This can be useful in scenarios where the number of arguments is not fixed.
Conclusion
Currying is a powerful technique in JavaScript that allows for the transformation of functions with multiple arguments into a sequence of functions with a single argument each. This technique enhances code modularity, composability, and readability. By understanding and implementing currying, developers can create more efficient and maintainable code, making it easier to manage complex functions and avoid passing the same variable multiple times.