Exploring the Features of JavaScript
Jul 25, 2024
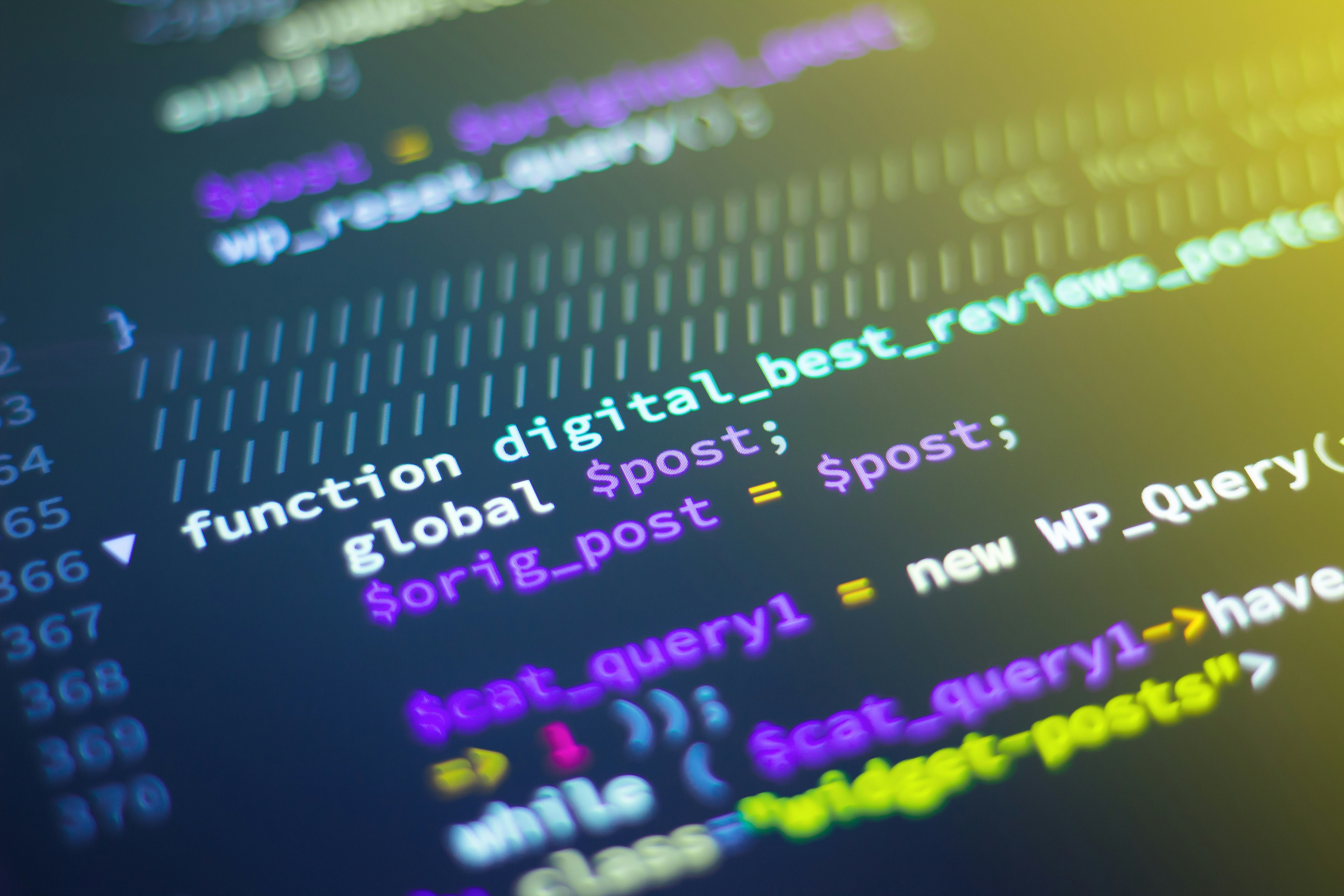
JavaScript is one of the most popular programming languages in the world, primarily known for its role in web development. It enables interactive web pages and is an essential part of web applications. In this blog post, we will explore the key features of JavaScript
, providing examples and code snippets to illustrate each point. Whether you are a beginner or an experienced developer, understanding these features will enhance your programming skills and improve your web development projects.
1. Dynamic Typing
One of the most notable features of JavaScript is its dynamic typing. This means that variables in JavaScript can hold any type of data, and their type can change at runtime. This flexibility allows developers to write more versatile code.
Example:
2. First-Class Functions
JavaScript treats functions as first-class citizens, meaning that functions can be assigned to variables, passed as arguments to other functions, and returned from functions. This feature is fundamental to functional programming in JavaScript.
Example:
3. Object-Oriented Programming (OOP)
JavaScript supports object-oriented programming
through the use of objects and prototypes. You can create objects using constructors or object literals, and JavaScript allows for inheritance through prototypes.
Example:
4. Asynchronous Programming
JavaScript is known for its non-blocking nature, allowing for asynchronous programming
. This is crucial for web applications that need to perform tasks like fetching data from a server without freezing the user interface.
Example: Using Promises
5. Event Handling
JavaScript provides a robust event handling system
, allowing developers to respond to user interactions such as clicks, form submissions, and keyboard inputs. This feature is essential for creating interactive web applications.
Example:
6. Template Literals
Template literals are a powerful feature introduced in ES6 that allows for easier string interpolation and multi-line strings. They use backticks (`) instead of quotes.
Example:
7. Arrow Functions
Arrow functions provide a more concise syntax for writing function expressions. They also lexically bind thethis
value, which can help avoid common pitfalls with traditional function expressions.
Example:
8. Destructuring Assignment
Destructuring assignment is a feature that allows unpacking values from arrays or properties from objects into distinct variables. This makes code cleaner and easier to read.
Example:
Conclusion
JavaScript is a powerful and versatile programming language with a wide array of features that make it indispensable for modern web development. From dynamic typing and first-class functions to asynchronous programming and modular design, understanding these features will enable developers to create more efficient and effective web applications.