Function Generator in JavaScript: A Comprehensive Guide
Aug 20, 2024
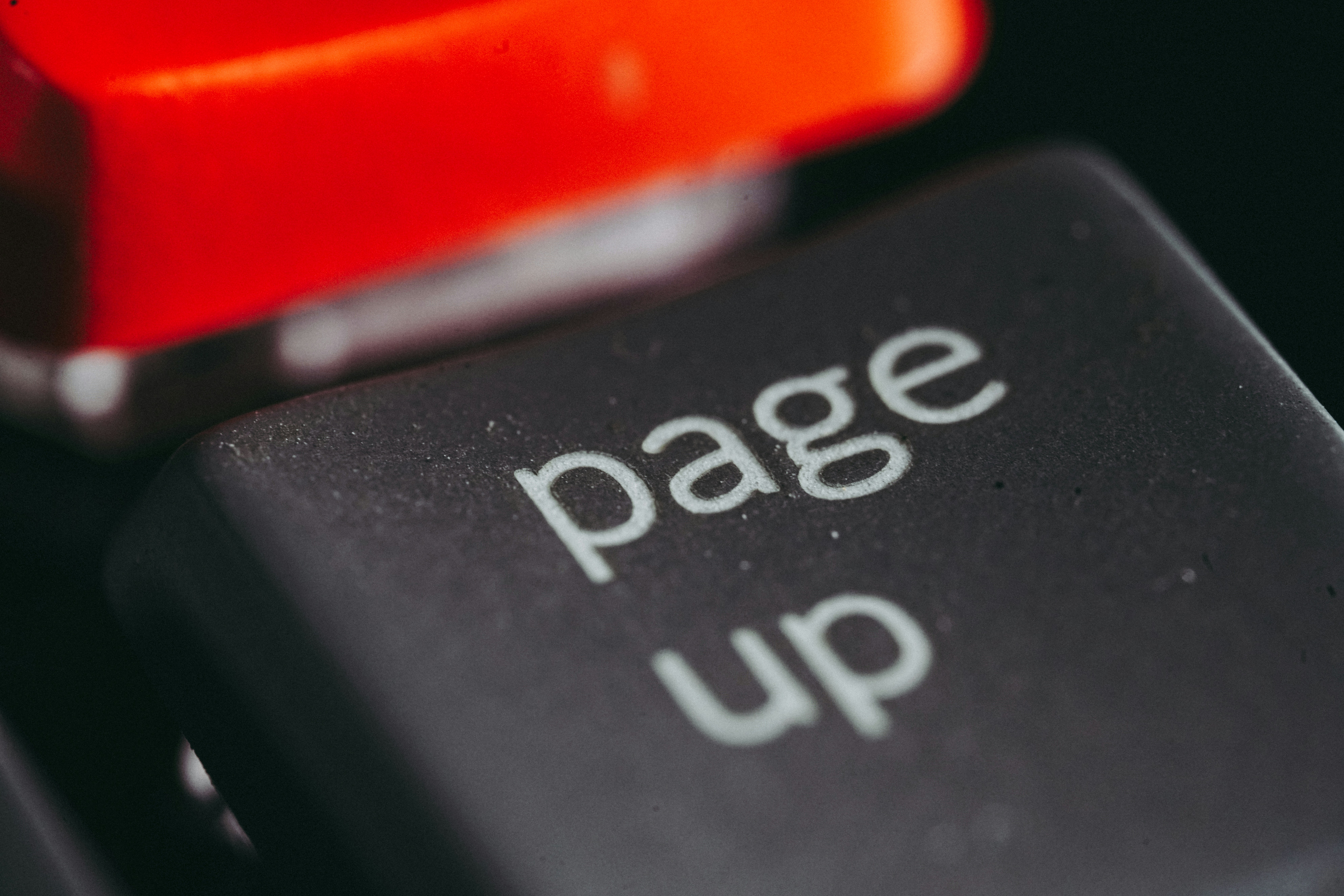
In the realm of programming, particularly in JavaScript, the concept of a function generator is both powerful and versatile. This blog post explores the intricacies of function generators in JavaScript, their syntax, use cases, and how they can enhance your coding practices.
Introduction to Function Generators
Function generators are a special type of function in JavaScript that allow you to define an iterative algorithm by using the function*
syntax. Unlike regular functions, which return a single value, generators can yield multiple values over time, pausing and resuming their execution. This makes them particularly useful for handling asynchronous programming, managing state, and implementing complex iteration patterns.
Understanding the Syntax
To define a generator function, you use the function*
keyword followed by the function name. Inside the function, you can use the yield
keyword to pause the function execution and return a value to the caller. The function can be resumed later, continuing from where it left off.
In the example above, myGenerator
is a generator function that yields three values: 1, 2, and 3.
How to Use Function Generators
To utilize a generator function, you must first create an instance of the generator by calling the function. This returns an iterator object that can be used to control the flow of execution.
The next()
method is called on the generator instance to retrieve the next value. Each call to next()
resumes the generator function until it hits the next yield
statement or completes execution.
Use Cases for Function Generators
Function generators can be employed in various scenarios, including:
Asynchronous Programming: Generators can simplify asynchronous code, allowing for a more synchronous style of programming using libraries like
co
orasync/await
.State Management: They can maintain state across multiple invocations, making them ideal for managing complex data flows.
Custom Iterators: Generators can be used to create custom iterators for collections, providing a clean and efficient way to traverse data structures.
Advanced Features of Function Generators
Yielding Multiple Values
Generators can yield multiple values, allowing for more complex data handling. You can also pass values back into the generator using the next()
method.
In this example, the generator function calculator
maintains a running total and updates it based on input values passed back into the generator.
Using for...of
with Generators
You can iterate over the values yielded by a generator using a for...of
loop, which simplifies the process of consuming generator values.
Conclusion
Function generators in JavaScript provide a robust mechanism for managing iteration and asynchronous programming. By leveraging thefunction*
syntax and theyield
keyword, developers can create efficient and maintainable code that handles complex data flows seamlessly.
For more information on JavaScript functions and advanced programming techniques, check outMDN Web DocsandJavaScript.info.