How to Add Line Break Between Checkbox Items
Aug 6, 2024
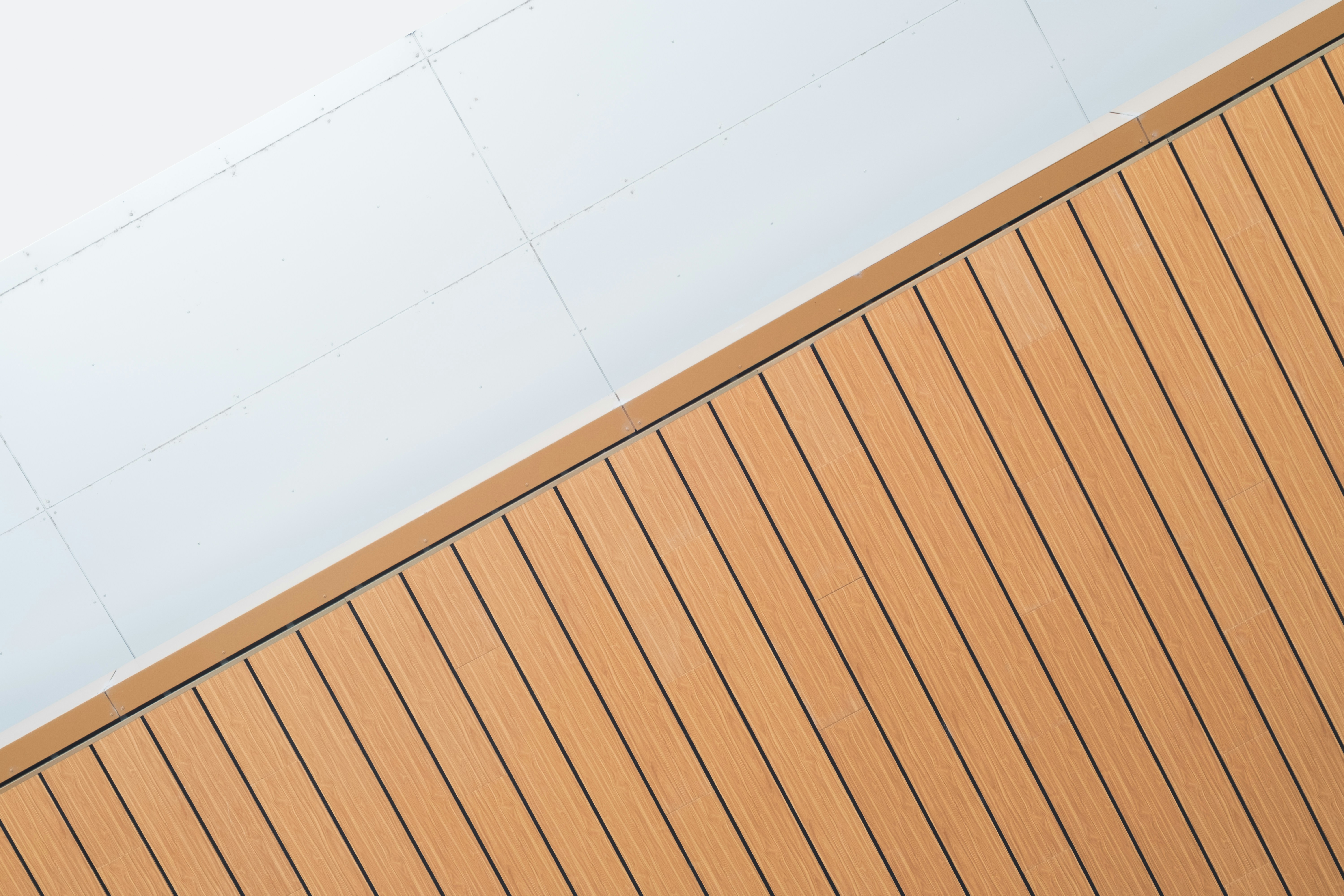
When creating a list of checkboxes in HTML, you may want to add line breaks between the checkbox and its corresponding label text. This can make the list more visually appealing and easier to read. Here are a few methods to achieve this:
Using the <br>
Tag
The simplest way to add a line break between a checkbox and its label is to use the <br>
tag after the checkbox input element:
This will create a checkbox list with a line break after each checkbox, resulting in the following output:
[] Item 1
[] Item 2
[] Item 3
Using CSS display
Property
Alternatively, you can use CSS to control the display of the checkbox and label elements. By setting the display
property of the checkbox to block
, it will create a line break before the label:
This will result in the same output as the previous method:
[] Item 1
[] Item 2
[] Item 3
Using CSS margin
or padding
Another way to add spacing between the checkbox and label is by using CSS margin
or padding
. You can apply a bottom margin or padding to the checkbox or a top margin/padding to the label:
This will add 10 pixels of space between each checkbox and label:
[] Item 1
[] Item 2
[] Item 3
Using CSS float
and clear
You can also use the float
property to position the checkbox and label side by side, and then use clear
to create a line break:
This will create a checkbox list with each item on a new line:
[] Item 1
[] Item 2
[] Item 3
Using CSS display: flex
If you want more control over the layout and spacing, you can use the display: flex
property along with flex-direction: column
to create a vertical layout and margin
to add spacing:
This will create a checkbox list with each item on a new line and a 10-pixel margin between each item:
[] Item 1
[] Item 2
[] Item 3
Conclusion
There are several ways to add line breaks between checkbox items in HTML. The most suitable method depends on your specific requirements and preferences. Consider factors such as simplicity, flexibility, and control over the layout when choosing the best approach for your project.