How to Capitalize the First Letter of a String in JavaScript
Jul 25, 2024
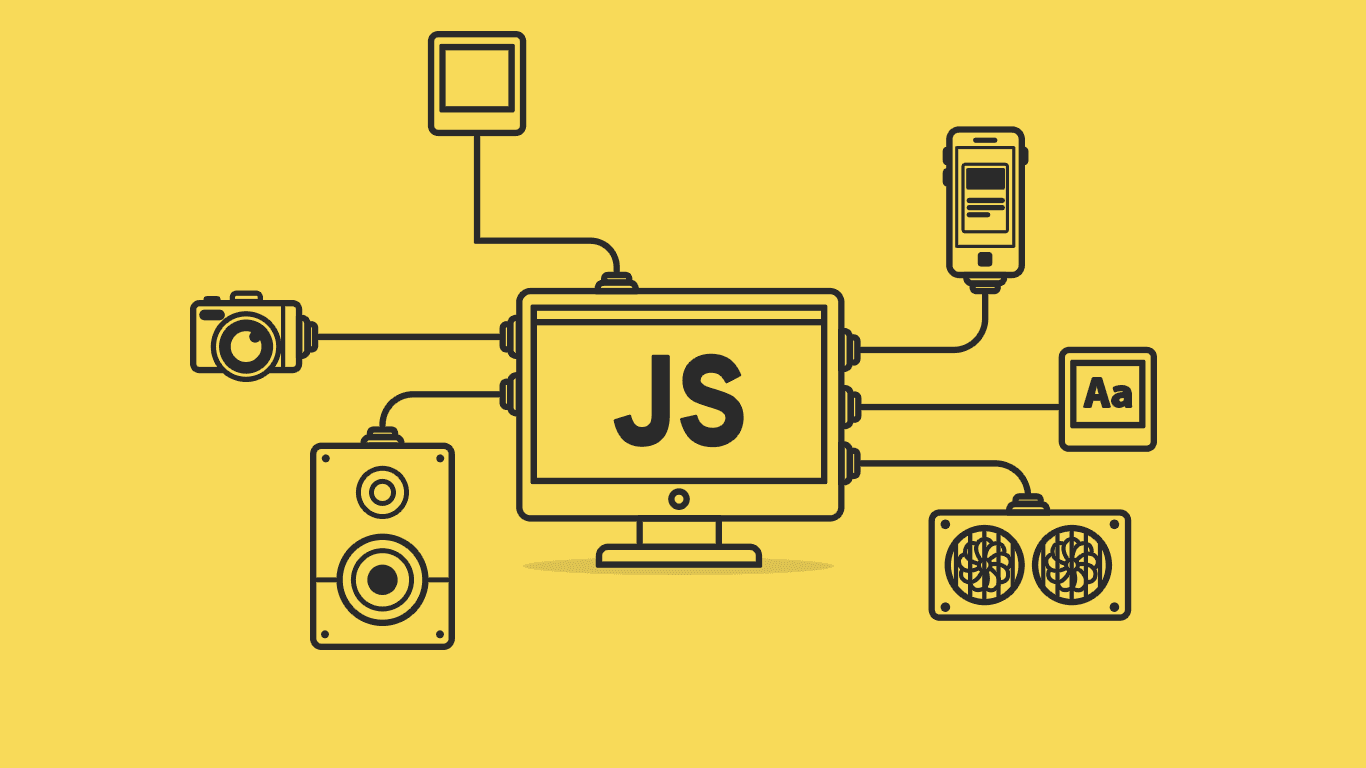
Capitalizing the first letter of a string is a common task in JavaScript. Here are a few ways to achieve this:
Using the toUpperCase()
method and charAt()
function
The charAt(0)
function returns the first character of the string. We then chain the toUpperCase()
method to capitalize this character. Finally, we use slice(1)
to get the rest of the string (from index 1 to the end) and concatenate it back to the capitalized first letter.
Using regular expressions
The regular expression /^\w/
matches the first word character (letter, digit, or underscore) at the beginning of the string (^
). We then pass a callback function to replace()
that capitalizes the matched character using toUpperCase()
.
Using template literals
Template literals allow us to embed expressions using ${}
. We use the same approach as the first method, but with template literals for a more concise syntax.These are just a few examples of how to capitalize the first letter of a string in JavaScript. The choice depends on your personal preference and the specific requirements of your project.
Optimizing Blog Posts for Search Engines
Writing SEO-friendly blog posts is crucial for attracting organic traffic to your website. Here are some tips to optimize your blog content:
1. Choose blog topics with keyword research
Use keyword research tools to find topics that your target audience is searching for. Focus on long-tail keywords that are more specific and have lower competition.
2. Write a compelling blog post title
The title should be attention-grabbing, informative, and include your target keyword. Keep it under 60 characters for optimal display in search results.
3. Outline your blog post with SEO in mind
Plan the structure of your post, including an introduction, body paragraphs, and a conclusion. Use relevant subheadings (H2, H3) to break up the content and make it more scannable.
4. Use keywords strategically throughout the blog post
Incorporate your target keyword naturally in the introduction, body paragraphs, and conclusion. Avoid keyword stuffing, as it can negatively impact your SEO.
5. Make sure your blog post covers your topic completely
Provide comprehensive, in-depth information to satisfy the search intent of your readers. Longer, more detailed posts tend to rank better in search results.
6. Add SEO-optimized images and videos
Break up your text with relevant images and videos. Include descriptive alt text for images to help search engines understand their content.
7. Link to related blog posts
Improve user experience and reduce bounce rates by linking to other relevant content on your website. This also helps search engines understand the structure and hierarchy of your site.
8. Optimize the meta description
The meta description is the short summary that appears in search results. Keep it under 160 characters, include your target keyword, and make it compelling to encourage clicks.
9. Review metrics regularly
Monitor your blog post's performance using analytics tools. Track metrics like traffic, bounce rate, and conversion rate to identify areas for improvement and optimize your content over time.By following these tips and continuously refining your SEO strategy, you can create blog posts that rank well in search engines and attract qualified traffic to your website.