How to Check if a Variable is True or False in JavaScript
Aug 9, 2024
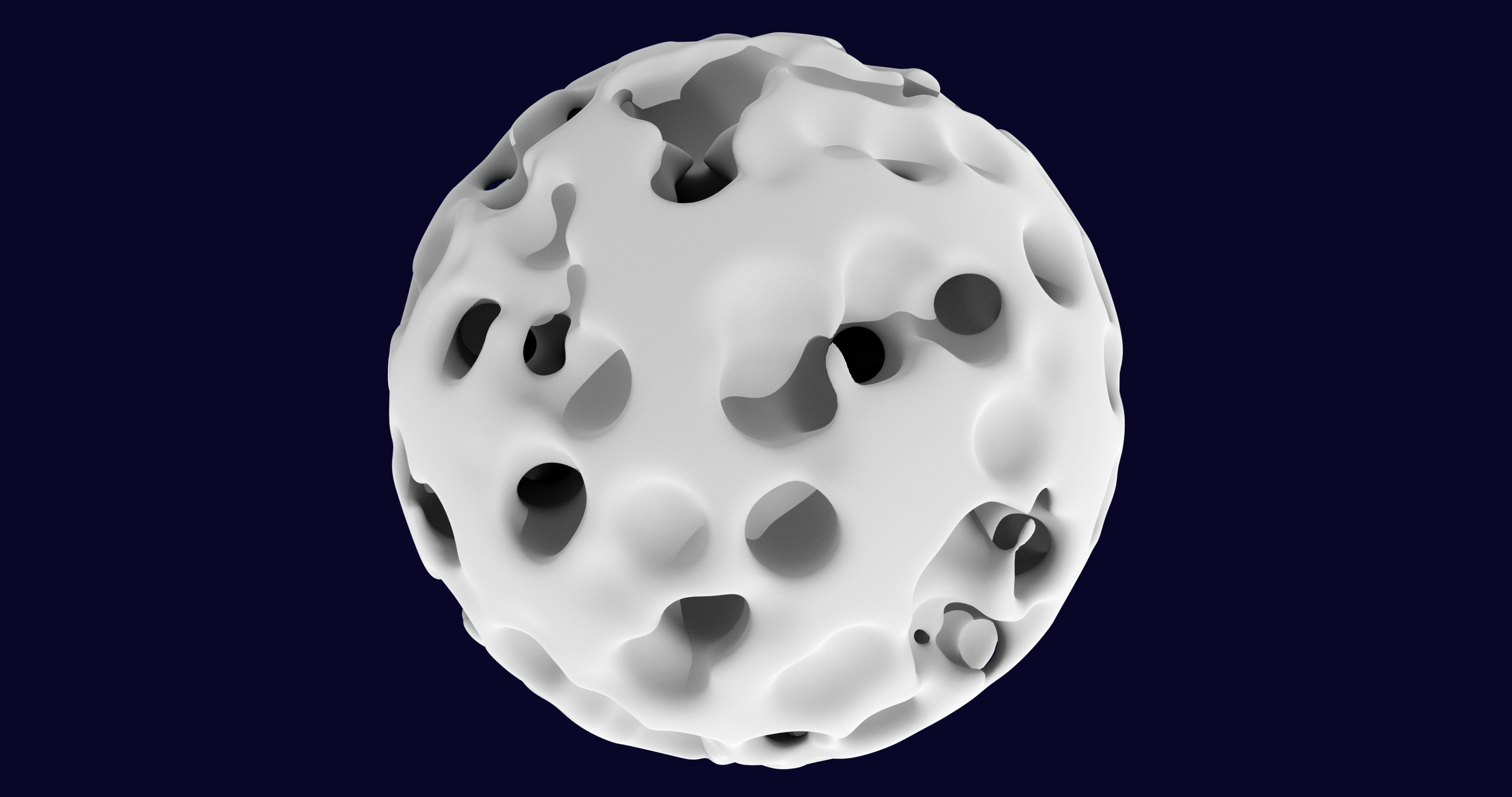
JavaScript is a dynamically typed language, which means variables can hold values of any data type, including booleans. Booleans are logical values that represent true or false. In JavaScript, you can check if a variable is true or false using various methods. In this blog post, we'll explore different techniques and best practices for checking boolean values in JavaScript.
Using the Strict Equality Operator (===)
One of the most common ways to check if a variable is true or false is by using the strict equality operator (===
). This operator compares both the value and the data type of the operands. Here's an example:
In this code snippet, we're checking if booleanValue
is strictly equal to true
. If the condition is true, the function will return the string "something". Some developers argue that using ===
is unnecessary when checking boolean values because JavaScript automatically converts the variable to a boolean value in a boolean context. They suggest using a more concise approach:
}
In this example, the conditionif (booleanValue)
is equivalent toif (booleanValue === true)
becausebooleanValue
is already a boolean value.However, it's important to note that using===
can help prevent confusion and ensure that the variable is exactly equal totrue
. This is especially useful when dealing with loosely typed languages like JavaScript, where variables can hold different data types.
Using the typeof Operator
Another way to check if a variable is a boolean is by using thetypeof
operator. This operator returns the data type of the operand as a string. Here's an example:
}
In this code snippet, we're usingtypeof
to check ifbooleanValue
is of type 'boolean'. If the condition is true, it meansbooleanValue
is a boolean, and the code inside theif
block will execute.
Using the toString.call() Method
Another method to check if a variable is a boolean is by using thetoString.call()
method. This method returns a string representation of the object type. Here's an example:
In this code snippet, we're using toString.call()
to check if the string representation of booleanValue
is '[object Boolean]'. If the condition is true, it means booleanValue
is a boolean.
Boolean Primitives and Boolean Objects
In JavaScript, there are two ways to represent boolean values: primitive and object. Primitive booleans are the most common and efficient way to work with boolean values. They are created using a literal value (true
or false
) or by converting a non-boolean value to a boolean using the Boolean()
function or the double NOT operator (!!
).
Comparing Truthy and Falsy Values
In JavaScript, values are either truthy or falsy. Falsy values are values that are considered false when converted to a boolean. The following values are falsy in JavaScript:
false
0
(zero)0n
(BigInt zero)''
(empty string)null
undefined
NaN
(Not a Number)
All other values are considered truthy, including non-zero numbers, non-empty strings, objects, and arrays.
When checking the truthiness of a value, JavaScript automatically converts the value to a boolean. This behavior is known as boolean coercion and is used extensively in conditional statements, loops, and logical operators.
Using Logical Operators for Truthiness Checks
JavaScript provides logical operators that can be used to check the truthiness of values. The logical AND (&&
) operator returns the first operand if it is falsy, otherwise it returns the second operand.
The logical OR (||
) operator returns the first operand if it is truthy, otherwise it returns the second operand.
Conclusion
Checking if a variable is true or false in JavaScript is a common task, and there are several ways to accomplish it. Using the strict equality operator (===
), thetypeof
operator, or thetoString.call()
method can help ensure that the variable is exactly equal totrue
orfalse
. It's important to understand the differences between boolean primitives and boolean objects, as well as the concept of truthy and falsy values in JavaScript.By mastering these techniques and best practices, you can write more robust and maintainable code when working with boolean values in JavaScript.