How to Create a CSS Button Half Out of a Div
Aug 17, 2024
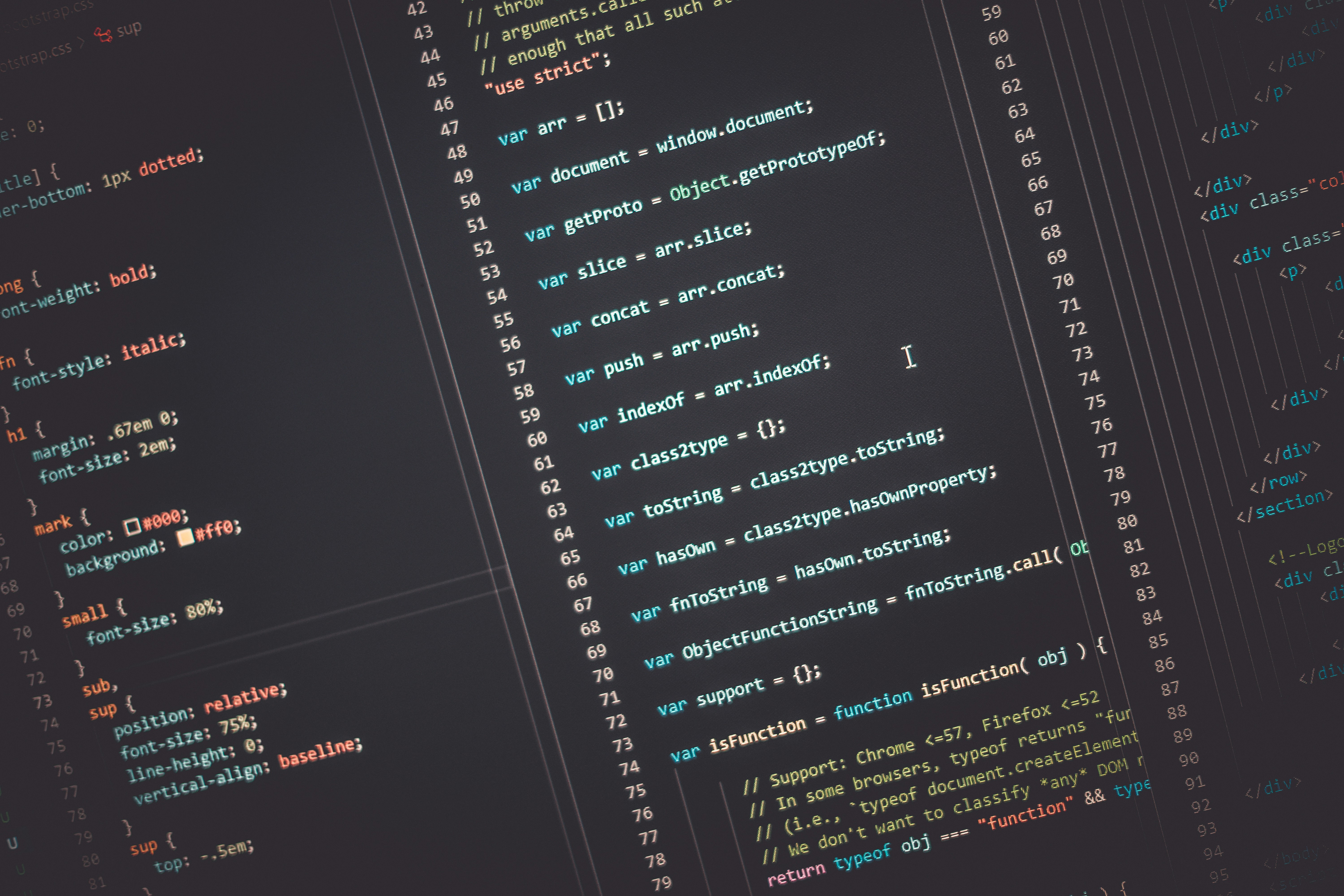
Are you looking to create a unique and eye-catching button design for your website? One popular technique is to have a button that appears to be half out of its containing div. This creates an interesting visual effect that can help your buttons stand out. In this blog post, we'll walk you through the step-by-step process of creating a CSS button that is half out of its parent div.
Understanding the Concept
Before we dive into the code, let's first understand the concept behind this technique. The key idea is to position the button element partially outside its parent container using CSS positioning properties. By setting the button's top or bottom position to a negative value, we can make it overlap with the container's border, creating the illusion of the button being half out of the div.
Step 1: Set up the HTML Structure
Let's start by creating the basic HTML structure for our button and its parent container:
In this example, we have a <div>
element with the class "container" that serves as the parent container for our button. Inside it, we have a <button>
element with the class "btn" representing our button.
Step 2: Style the Container
Next, let's style the container div using CSS:
We set a background color for the container using background-color
, add some padding using padding
, and set the position
property to relative
. This will allow us to position the button relative to its parent container.Additionally, we set overflow
to hidden
. This is important because it will hide any part of the button that extends beyond the container's boundaries.
Step 3: Style the Button
Now, let's style the button:
Here's what each CSS property does:
background-color
: Sets the background color of the button.border
: Removes the default button border.color
: Sets the text color of the button.padding
: Adds some padding around the button text.text-align
: Centers the button text.text-decoration
: Removes the default underline from the button text.display
: Sets the button to be an inline-block element.font-size
: Sets the font size of the button text.position
: Sets the button's position toabsolute
, allowing us to position it relative to its parent container.bottom
: Positions the button 20 pixels above its original position, making it appear half out of the container.left
: Centers the button horizontally within the container.transform
: Horizontally centers the button by translating it 50% of its own width to the left.
Step 4: Adjust the Button Position
To fine-tune the button's position, you can adjust the bottom
value in the .btn
class. A negative value will make the button appear more out of the container, while a positive value will make it appear more inside the container.
You can also adjust the padding
and font-size
properties to change the button's size and appearance.
Step 5: Add Hover Effect (Optional)
To make the button more interactive, you can add a hover effect:
This CSS rule changes the button's background color when the user hovers over it, providing visual feedback.
Complete CSS Code
Here's the complete CSS code for creating a CSS button half out of a div:
Conclusion
Creating a CSS button that appears half out of its parent div is a simple yet effective way to add visual interest to your website. By using CSS positioning and theoverflow
property, you can achieve this unique design. Remember to adjust the button's position and size to fit your specific needs. Happy coding!