How to Create a URL in JavaScript
Aug 22, 2024
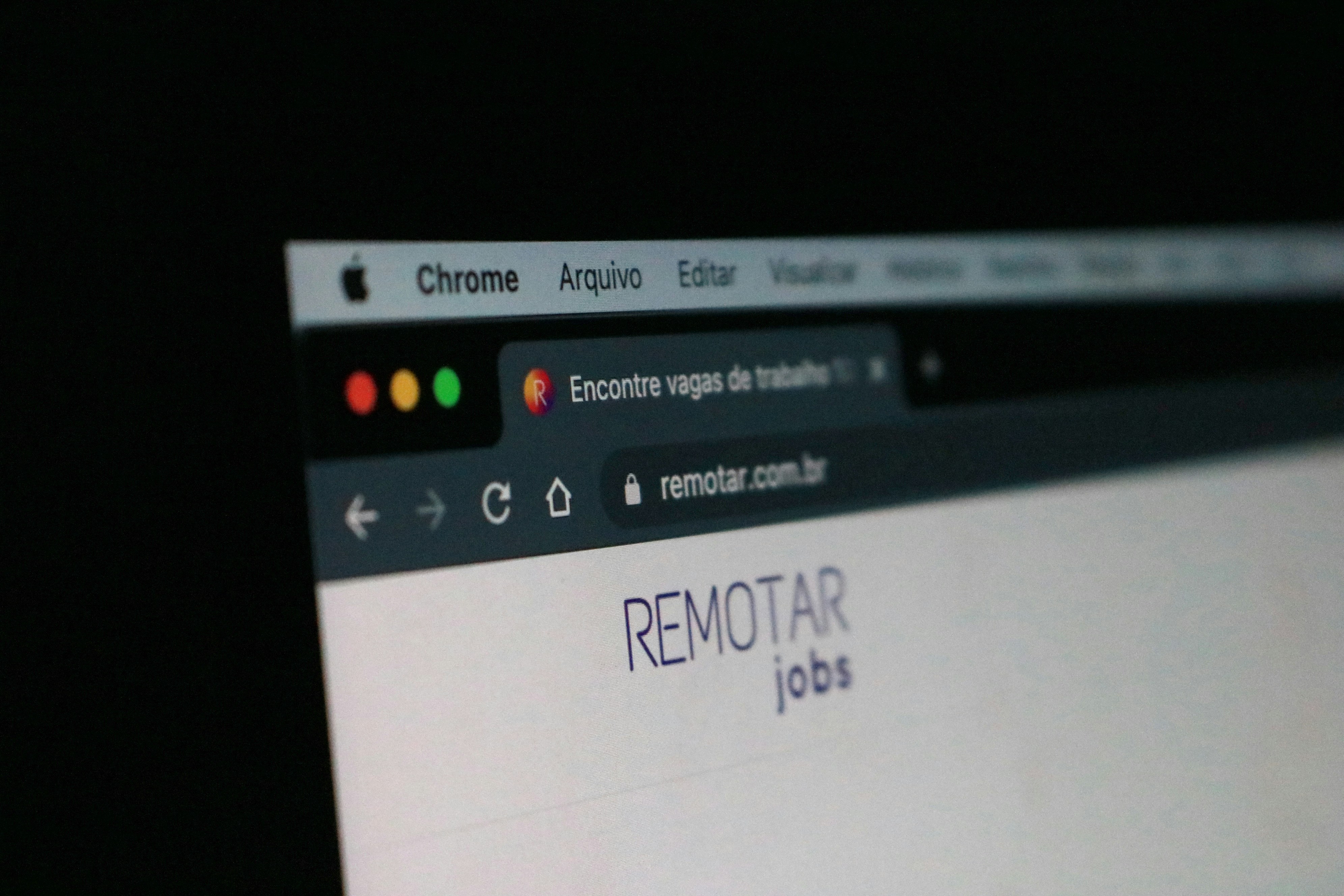
Creating URLs dynamically in JavaScript is an essential skill for web developers. Whether you're building a single-page application (SPA), handling routing, or simply manipulating links, understanding how to create and manage URLs is crucial. This comprehensive guide will cover various methods and techniques for creating URLs in JavaScript, including code snippets and practical examples.
Understanding URLs
Before diving into the code, let's clarify what a URL (Uniform Resource Locator) is. A URL is a reference to a web resource that specifies its location on a computer network and a mechanism for retrieving it. A typical URL consists of several components:
Protocol: The method used to access the resource (e.g.,
http
,https
,ftp
).Host: The domain name or IP address of the server (e.g.,
www.example.com
).Path: The specific location of the resource on the server (e.g.,
/path/to/resource
).Query String: A set of parameters that provide additional information to the server (e.g.,
?key1=value1&key2=value2
).Fragment: A reference to a specific part of the resource (e.g.,
#section1
).
Creating a URL Using the URL Constructor
JavaScript provides a built-in URL
constructor that makes it easy to create and manipulate URLs. This constructor can be used to create a new URL object from a string.
Basic Syntax
Example: Constructing a URL
Here's how to create a URL using theURL
constructor:
Manipulating URL Components
Once you've created a URL object, you can easily manipulate its components. TheURL
object provides properties for each part of the URL.
Accessing URL Components
Modifying URL Components
You can modify these properties to change the URL dynamically.
Creating URLs with Query Parameters
When working with URLs, you often need to add query parameters. TheURLSearchParams
interface provides a convenient way to work with the query string of a URL.
Adding Query Parameters
Removing Query Parameters
You can also remove parameters easily:
Encoding URLs
When creating URLs, it's essential to ensure that special characters are properly encoded. JavaScript provides functions likeencodeURIComponent
andencodeURI
for this purpose.
Encoding Components
Using the History API for Navigation
In single-page applications, you often need to manipulate the URL without reloading the page. The History API allows you to change the URL and manage browser history.
Pushing a New URL
Handling URL Changes
When using the History API, you should also handle thepopstate
event to respond to back and forward navigation.
Best Practices for URL Creation
Use the URL Constructor: Always prefer the
URL
constructor for creating and manipulating URLs to avoid common pitfalls with string concatenation.Encode Parameters: Always encode query parameters to ensure that special characters do not break your URLs.
Use Meaningful Paths: Design your URL paths to be meaningful and descriptive, which can improve SEO.
Maintain Consistency: Ensure that your URL structure is consistent across your application for better user experience and SEO.
Test Your URLs: Always test your generated URLs to ensure they behave as expected.
Conclusion
Creating and managing URLs in JavaScript is a fundamental skill for modern web development. By leveraging theURL
constructor,URLSearchParams
, and the History API, you can create dynamic, user-friendly URLs that enhance the functionality of your web applications.
For more information on web development best practices, check out resources from MDN Web Docs and W3Schools.