How To Make An Inputs Appear Below Another In HTML
Aug 17, 2024
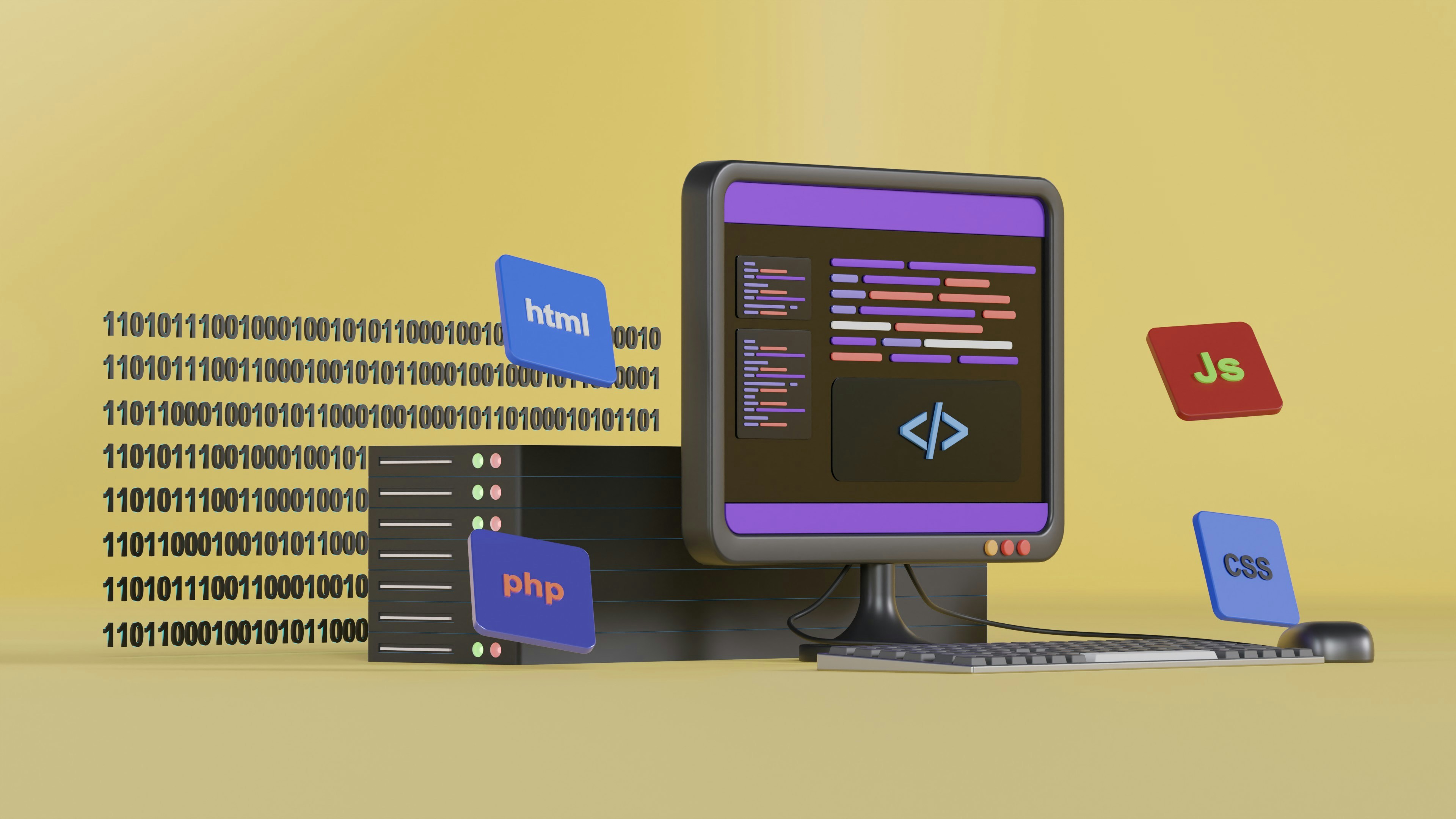
To create a blog post on "how to make inputs appear below another in HTML," we will cover the necessary HTML structure, CSS styling, and practical examples. This guide will help you understand how to effectively position input fields in your web forms.
Understanding HTML Structure
HTML (HyperText Markup Language) is the standard markup language for creating web pages. To make inputs appear below one another, you can use various HTML elements and CSS properties. The most common approach is to use the <form>
element to contain your inputs, along with block-level elements like <div>
or <p>
to ensure that each input starts on a new line.
Basic HTML Form Structure
Here’s a simple example of an HTML form with inputs stacked vertically:
Explanation of the Code
<form>
: This tag defines the form and contains all the input elements.<div>
: Each input field is wrapped in a<div>
to ensure they are block-level elements, which means they will stack vertically.<label>
: Labels are associated with their respective input fields, improving accessibility.<input>
: Different types of inputs (text, email, password) are used to gather user information.
CSS Styling for Vertical Alignment
To enhance the appearance of your form and ensure the inputs are visually appealing, you can apply some CSS. Here’s an example of a simple CSS file (styles.css
) that styles the form:
Explanation of the CSS
body
: Sets the font and background color for the entire page.form
: Styles the form with padding, background color, and shadow for a clean look.div
: Adds space between each input field.label
: Ensures labels are displayed as block elements, so they appear above the inputs.input
: Styles the input fields to be full-width with padding for better usability.button
: Styles the submit button and adds hover effects for interactivity.
Using Flexbox for Advanced Layouts
If you want to explore more advanced layouts, CSS Flexbox can be a powerful tool. Here’s how you can use Flexbox to stack inputs vertically:
HTML Structure with Flexbox
You can modify the previous HTML slightly to utilize Flexbox:
CSS for Flexbox
Here’s the CSS to style the Flexbox layout:
Explanation of Flexbox CSS
display: flex;
: This sets the form to use Flexbox.flex-direction: column;
: This arranges the items in a column, ensuring they stack vertically.
Conclusion
In this guide, we explored how to make inputs appear below one another in HTML using various methods, including basic HTML structure, CSS styling, and Flexbox layouts. By understanding these techniques, you can create user-friendly forms that enhance the user experience on your website.