HTML Concatenate Two Spans
Aug 9, 2024
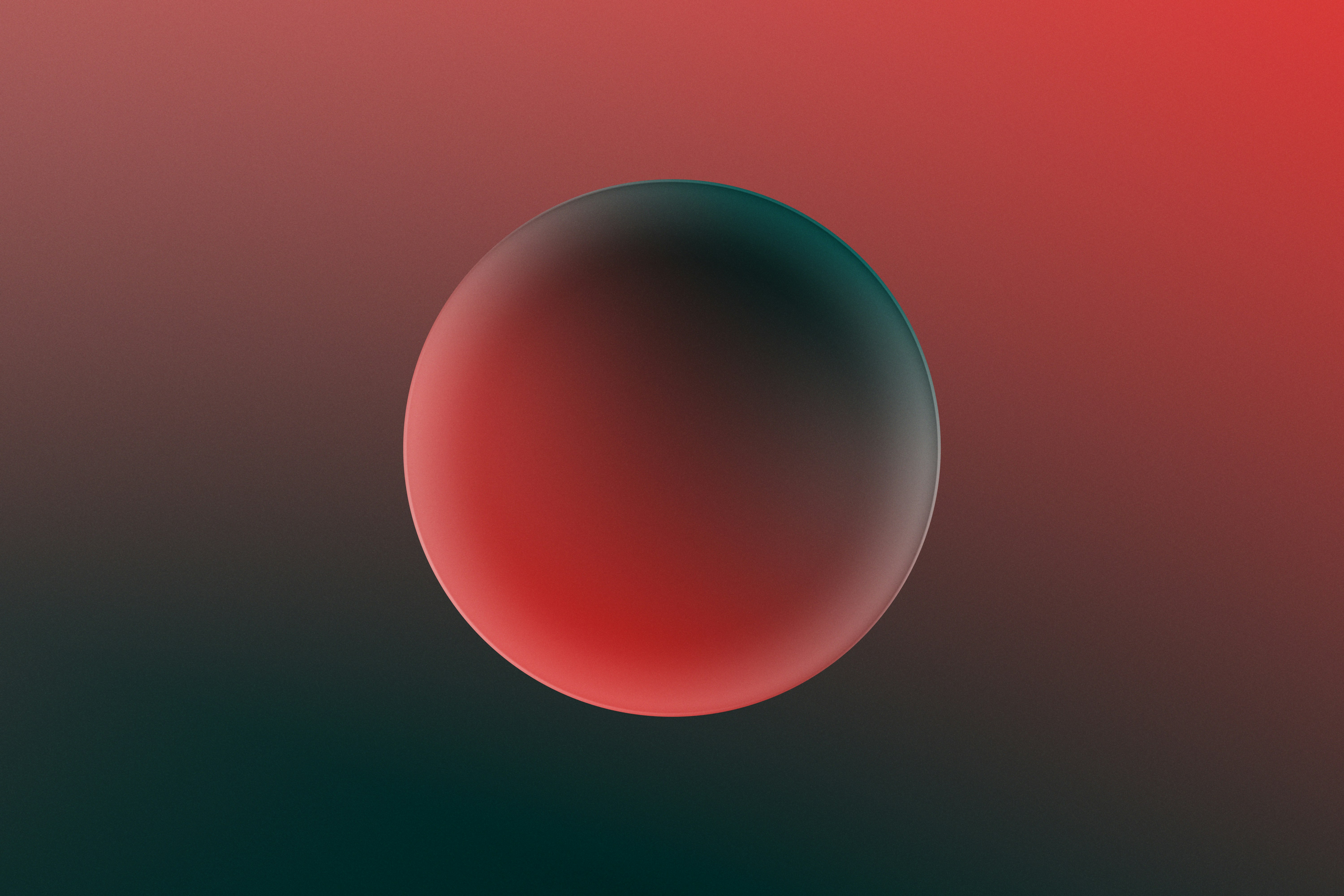
In web development, the ability to manipulate HTML elements such as <span>
tags is crucial for creating dynamic and interactive user experiences. This blog post will explore how to concatenate two <span>
elements in HTML, focusing on the JavaScript methods that allow you to join multiple arrays into one variable, as well as the use of the concat
method in strings.
Understanding the HTML <span>
Tag
The <span>
tag is an inline container used to group and style text or elements within a document. It does not inherently provide any visual changes but allows for CSS and JavaScript manipulation, making it useful for applying styles and scripts to specific portions of text.
Example of Using <span>
Tags
In this example, the words "how" and "day" are wrapped in <span>
tags, which can be styled or manipulated using JavaScript.
Joining Multiple Arrays into One Variable
When working with JavaScript, you often need to combine multiple arrays into a single array. This can be achieved using various methods, including the concat
method, the spread operator, and the push
method.
1. Using the concat
Method
The concat
method is a straightforward way to join two or more arrays. It does not alter the original arrays but returns a new array containing the combined elements.
Example:
2. Using the Spread Operator
Introduced in ES6, the spread operator (...
) allows for a more concise syntax when merging arrays.
Example:
3. Using the push
Method
Thepush
method can also be used to combine arrays, but it modifies the original array.
Example:
Concatenating Two <span>
Elements
To concatenate two<span>
elements into one, you can use JavaScript to manipulate the DOM. This is particularly useful when you want to merge adjacent<span>
elements that share the same class or style.
Example Scenario
Suppose you have the following HTML:
You want to merge the adjacent <span>
elements with the class "matched" into a single <span>
.
JavaScript Code to Merge <span>
Elements
In this code snippet, we select all <span>
elements with the class "matched", concatenate their text content, and create a new <span>
that contains the merged text.
Advantages of Concatenating <span>
Elements
Improved Readability: Merging
<span>
elements can lead to cleaner HTML, making it easier to read and maintain.Enhanced Styling: By combining multiple spans, you can apply styles more effectively, as fewer elements can lead to simpler CSS rules.
Dynamic Content Management: In applications where content changes frequently, dynamically merging spans can improve performance and user experience.
Conclusion
In this post, we explored how to concatenate two <span>
elements in HTML using JavaScript, alongside methods for joining multiple arrays into one variable. Understanding these concepts is essential for efficient web development, allowing you to create more dynamic and interactive web pages.