Introduction to Bayesian Machine Learning
Aug 13, 2024
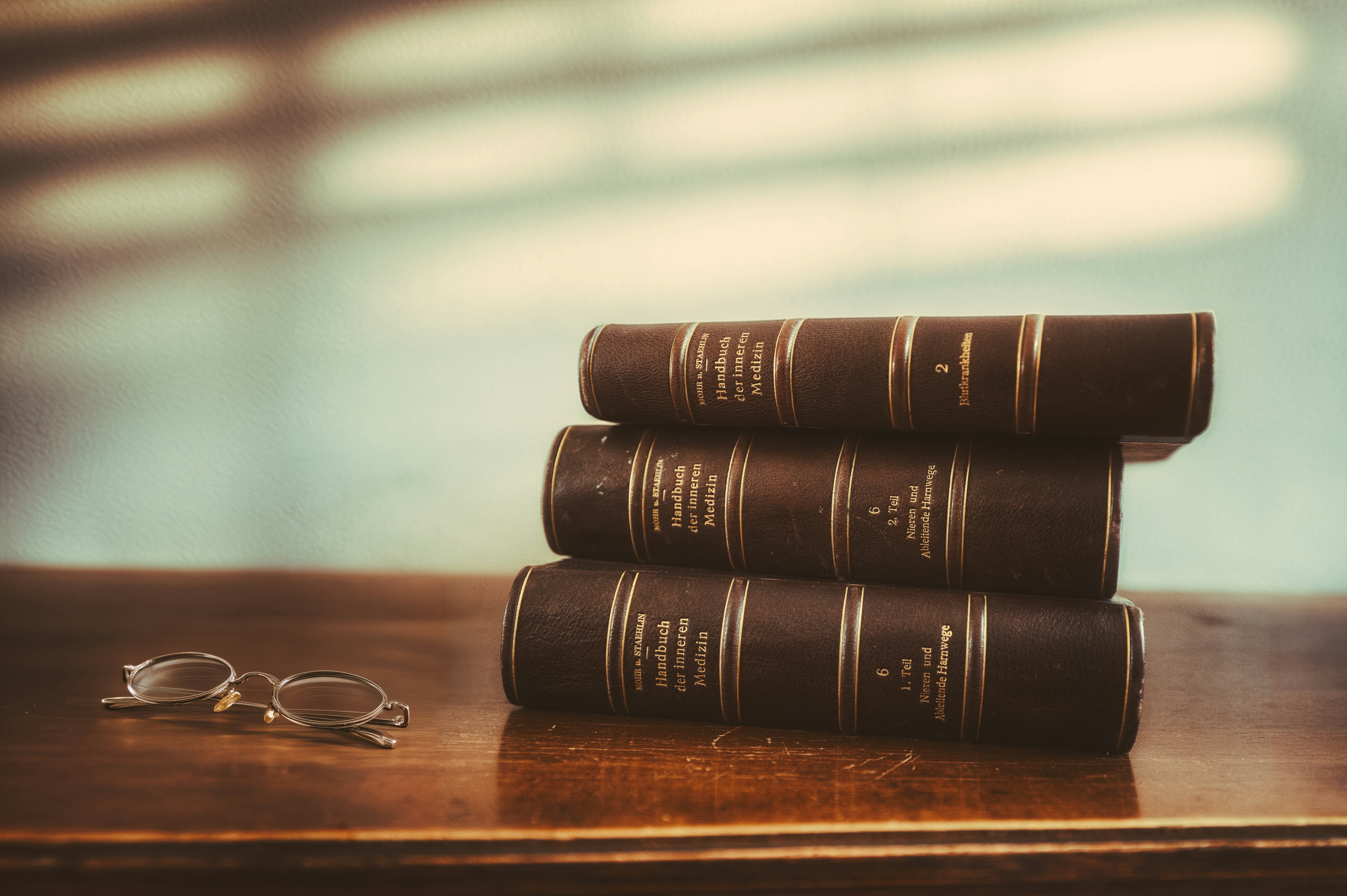
Bayesian machine learning is a powerful paradigm that combines Bayesian statistics with machine learning techniques to make predictions and inferences while accounting for uncertainty. This approach leverages Bayes’ theorem, which allows us to update our beliefs about a hypothesis based on new evidence, leading to more informed decision-making. As the field of machine learning continues to evolve, Bayesian methods are gaining traction due to their ability to provide not only predictions but also insights into the uncertainty of those predictions.
What is Bayesian Machine Learning?
At its core, Bayesian machine learning is about understanding and quantifying uncertainty. The fundamental formula behind Bayesian machine learning
is given by Bayes' theorem:
P(H∣D)=P(D∣H)⋅P(H)P(D)
Where:
P(H∣D)P(H∣D) is the posterior probability of hypothesis HH given the observed data DD.
P(D∣H)P(D∣H) is the likelihood of the data DD given the hypothesis HH.
P(H)P(H) is the prior probability of hypothesis HH.
P(D)P(D) is the probability of the observed data DD.
This formula allows us to update our prior beliefs (P(H)P(H)) based on new evidence (P(D∣H)P(D∣H)) to calculate the posterior probabilities (P(H∣D)P(H∣D)).
The Importance of Bayesian Machine Learning
Bayesian machine learning is particularly useful in scenarios where data is scarce or noisy, as it allows for the incorporation of prior knowledge into the learning process. This is crucial in fields such as medical diagnostics, finance, and robotics, where decisions must be made under uncertainty. The ability to quantify uncertainty helps practitioners make better-informed decisions and manage risks more effectively.
Applications of Bayesian Machine Learning
Bayesian machine learning has a wide range of applications across various domains:
Spam Filtering: By analyzing the likelihood of certain words appearing in emails, Bayesian classifiers can effectively distinguish between spam and non-spam emails.
Credit Card Fraud Detection: Bayesian methods can identify patterns in transaction data to infer the probability of fraud, allowing for real-time alerts.
Weather Prediction: Bayesian models can be used to predict weather patterns by updating forecasts based on new data.
Medical Diagnosis: Bayesian techniques can help in diagnosing diseases by combining patient symptoms with prior probabilities of diseases.
Natural Language Processing (NLP): Bayesian models are employed in various NLP tasks, including sentiment analysis and topic modeling.
Bayesian Inference Techniques
Bayesian inference is the process of updating the probability estimate for a hypothesis as more evidence or information becomes available. There are several techniques used in Bayesian inference, including:
Maximum Likelihood Estimation (MLE): This method estimates the parameters of a statistical model by maximizing the likelihood function, which measures how well the model explains the observed data.
Maximum A Posteriori (MAP): This approach seeks to maximize the posterior distribution, incorporating both the likelihood of the data and prior beliefs about the parameters.
Markov Chain Monte Carlo (MCMC): MCMC methods are used for sampling from probability distributions when direct sampling is difficult. These methods are particularly useful in complex models where the posterior distribution cannot be computed analytically.
Coding Bayesian Machine Learning
Implementing Bayesian machine learning models can be achieved using various programming languages and libraries. Below is a simple example using Python and the PyMC3 library
, which is widely used for probabilistic programming.
Example: Bayesian Linear Regression
In this example, we will create a Bayesian linear regression model using PyMC3
.
Explanation of the Code
Data Generation: We create synthetic data for our regression model, where
y
is a linear function ofX
with some added noise.Model Definition: We define a Bayesian model using
PyMC3
. We specify priors for the intercept (alpha
), slope (beta
), and noise (sigma
).Likelihood: We define the likelihood of the observed data given the model parameters.
Sampling: We use MCMC to sample from the posterior distribution of the model parameters.
Visualization: Finally, we visualize the trace of the sampled parameters.
Conclusion
Bayesian machine learning is a robust framework that allows for the incorporation of prior knowledge and the quantification of uncertainty in predictions. Its applications span various fields, making it a valuable tool for data scientists and machine learning practitioners. As computational power increases and more sophisticated algorithms are developed, the use of Bayesian methods is likely to expand further, providing deeper insights and more reliable predictions in complex real-world scenarios.