Introduction to Fibonacci Series in JavaScript
Jul 27, 2024
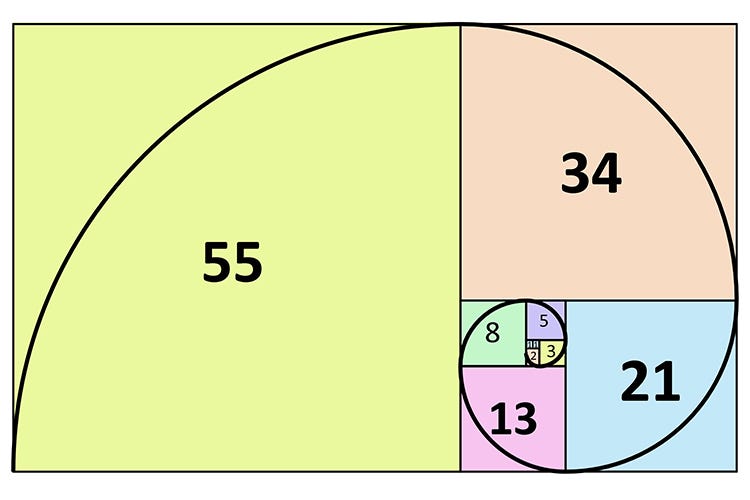
The Fibonacci series is a well-known mathematical sequence named after the Italian mathematician Leonardo of Pisa, also known as Fibonacci. This series starts with 0 and 1, and each subsequent number is the sum of the two preceding ones. The Fibonacci sequence is widely used in various fields, including mathematics, computer science, and nature.In this blog post, we will explore how to implement the Fibonacci series in JavaScript using different approaches, including loops and recursion. We will also discuss the time and space complexities of each method and provide examples to help you understand the concepts better.
What is the Fibonacci Series?
The Fibonacci series is a sequence of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. The sequence is defined as follows:
where:
$F_0 = 0$
$F_1 = 1$
$n \geq 2$
The first few terms of the Fibonacci series are: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ...
Fibonacci Series in JavaScript Using for Loop
One of the most common ways to generate the Fibonacci series in JavaScript is by using a for loop. Here's an example:
Explanation:
The
fibonacciSeries(n)
function is defined to calculate the Fibonacci series up ton
numbers.We start with an array
fib
initialized with the first two numbers of the Fibonacci series:[0, 1]
.The
for
loop starts from index 2 and runs until it reaches the lengthn
specified by the user. This is because the first two numbers of the series are already defined.Inside the loop, each number is calculated by adding the two preceding numbers in the series using
fib[i - 1] + fib[i - 2]
, and the result is stored infib[i]
.The function returns the Fibonacci series up to
n
numbers usingfib.slice(0, n)
. Theslice()
method is used to ensure that the length of the returned array matches the numbern
specified by the user.Finally, we call
fibonacciSeries(10)
to print the first 10 numbers of the Fibonacci series to the console.
This code is a simple and efficient way to use JavaScript for generating the Fibonacci series and is particularly useful for beginners to understand how arrays and loops work in the language.
Fibonacci Series in JavaScript Using while Loop
Another approach to generate the Fibonacci series in JavaScript is by using awhile
loop. Here's an example:
Explanation:
The
fibonacciSeriesUpTo(maxValue)
function is defined to generate the Fibonacci series up to a specified maximum value.If the
maxValue
is 0 or 1, the function returns an empty array or an array with[0, 1]
, respectively.The
fib
array is initialized with the first two numbers of the Fibonacci series:[0, 1]
.The
while
loop continues until the next Fibonacci number exceeds themaxValue
.Inside the loop, the next Fibonacci number is calculated by adding the last two numbers in the
fib
array usingfib[fib.length - 1] + fib[fib.length - 2]
.If the calculated next Fibonacci number is greater than the
maxValue
, the loop is broken using thebreak
statement.The calculated next Fibonacci number is added to the
fib
array usingfib.push(nextFib)
.Finally, the function returns the
fib
array containing the Fibonacci series up to the specifiedmaxValue
.The user is prompted to enter a number, which is converted to an integer using
parseInt()
.If the user input is a valid number, the
fibonacciSeriesUpTo()
function is called with the user input, and the result is logged to the console.If the user input is not a valid number, an error message is displayed.
This approach allows you to generate the Fibonacci series up to a certain number based on user input.