Introduction to Instance Based Learning in Machine Learning
Aug 12, 2024
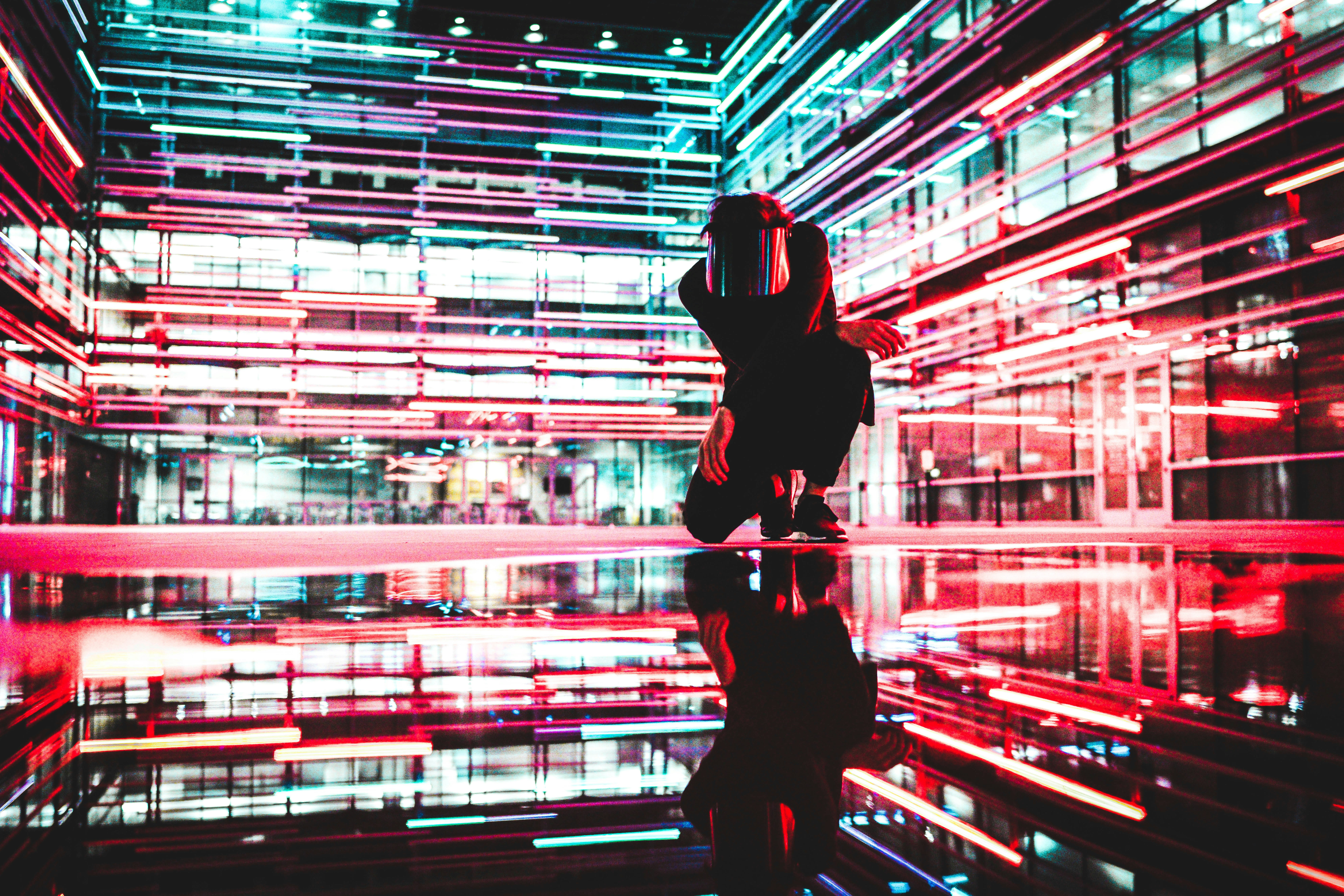
Instance-based learning, also known as memory-based learning or lazy learning, is a machine learning approach that makes predictions or classifications based on the similarity between new instances and the training examples. Unlike model-based learning algorithms that build a generalized model from the training data, instance-based learning stores the training instances and uses them directly for inference when new instances are encountered.
The core idea behind instance-based learning is that similar instances should have similar outputs or labels. When a new instance is presented, the algorithm searches for the most similar instances in the training data and uses their labels or values to make predictions for the new instance.
Key Characteristics of Instance-Based Learning
Instance-based learning has several key characteristics:
Instance storage: The training instances, comprising feature vectors and associated labels or values, are stored in memory for efficient retrieval and comparison during the prediction phase.
Similarity measure: A similarity measure or distance metric is defined to quantify the similarity between instances. Common distance metrics include Euclidean distance, Manhattan distance, or cosine similarity, depending on the type of data and the problem at hand.
Nearest neighbor search: When a new instance is presented, the algorithm searches for the nearest neighbors in the training data based on the defined similarity measure. The number of neighbors to consider, known as k, is a parameter that can be set based on the problem requirements.
Prediction or classification: Once the nearest neighbors are identified, the algorithm assigns a prediction or label to the new instance based on the labels or values of the nearest neighbors. This can involve various techniques, such as majority voting for classification tasks or weighted averaging for regression tasks.
Adaptation to local data: Instance-based learning allows for adaptation to local patterns in the data. As the training instances are stored, the algorithm can adjust predictions based on the distribution and characteristics of the nearest neighbors.
Popular Instance-Based Learning Algorithms
Several algorithms fall under the category of instance-based learning:
k-Nearest Neighbors (k-NN): The k-NN algorithm is the most widely recognized instance-based learning algorithm. It calculates the distance between the query instance and all stored instances, selects the k closest instances, and uses a majority vote or average to make predictions.
Self-Organizing Map (SOM): SOM is an unsupervised neural network algorithm that learns to produce a low-dimensional representation of the input space, called a map. It is often used for dimensionality reduction, clustering, and visualization of high-dimensional data.
Learning Vector Quantization (LVQ): LVQ is a supervised version of SOM, where the map is trained to represent different classes in the input space. It is used for classification tasks and can adapt to new data easily.
Locally Weighted Learning (LWL): LWL is a lazy learning algorithm that makes predictions based on a weighted average of the nearest neighbors, with weights determined by a kernel function. It is particularly useful for regression tasks with noisy data.
Case-Based Reasoning (CBR): CBR is a problem-solving paradigm that uses specific knowledge of past situations to solve new problems. It involves retrieving similar past cases, reusing their solutions, revising the solutions, and retaining the new solution.
Advantages of Instance-Based Learning
Instance-based learning offers several advantages:
Handling complex relationships: Instance-based learning can handle complex and non-linear relationships in the data without explicitly modeling them.
Adaptation to changing data: The algorithm can adapt to new data easily, as it can incorporate new instances as they become available.
Interpretability: Since the predictions are made based on known instances, it is easier to interpret why a decision was made, which is valuable in fields like medicine and finance.
Local approximations: Instead of estimating for the entire instance set, local approximations can be made to the target function.
Disadvantages of Instance-Based Learning
While instance-based learning has its advantages, it also has some limitations:
High computational cost: Classification costs can be high, as each query involves starting the identification of a local model from scratch.
Memory requirements: A large amount of memory is required to store the data, which can be a concern for large datasets.
Sensitivity to irrelevant features: Performance can degrade if the feature set includes irrelevant or redundant data, as this can skew the distance measurements.
Handling high-dimensional data: Instance-based learning may struggle with high-dimensional data due to the curse of dimensionality, where the distance between instances becomes less meaningful as the number of dimensions increases.
Implementing Instance-Based Learning with k-NN
Let's dive into the implementation of the k-Nearest Neighbors (k-NN) algorithm, which is a popular instance-based learning algorithm.
Preparing the Data
Suppose we have a dataset of iris flowers, where each instance is described by four features (sepal length, sepal width, petal length, and petal width) and belongs to one of three classes (setosa, versicolor, or virginica). We can load the dataset using scikit-learn:
Implementing k-NN
Here's a simple implementation of the k-NN algorithm in Python:
In this implementation:
We define a
euclidean_distance
function to calculate the Euclidean distance between two instances.The
knn_predict
function takes the training data (X_train
,y_train
), the test data (X_test
), and the number of neighborsk
as input.For each test instance
x_test
, we calculate the Euclidean distance betweenx_test
and each training instancex_train
.We sort the distances and retrieve the indices of the
k
nearest neighbors.We use these indices to get the labels of the
k
nearest neighbors fromy_train
.We determine the most common label among the
k
nearest neighbors usingmax(set(nearest_labels), key=nearest_labels.count)
and append it to they_pred
list.Finally, we return the predicted labels
y_pred
.
Evaluating the Model
To evaluate the performance of the k-NN model, we can use the accuracy score:
This will output the accuracy of the k-NN model on the test set.
Applications of Instance-Based Learning
Instance-based learning has been applied in various domains, including:
Medical diagnosis: Matching patient symptoms and histories to diagnose conditions based on records of similar past patients.
Recommendation systems: Suggesting products, movies, or music based on preferences demonstrated by similar users.
Financial forecasting: Predicting stock prices or market movements based on the patterns of similar historical data points.
Image recognition: Classifying images based on their similarity to labeled examples in the training set.
Text classification: Categorizing documents or emails based on their resemblance to instances in the training data.
Robotics: Enabling robots to learn from and adapt to specific situations encountered during operation.
Conclusion
Instance-based learning is a powerful machine learning approach that offers several advantages, such as handling complex relationships, adapting to changing data, and providing interpretable predictions. While it has limitations, such as high computational cost and sensitivity to irrelevant features, instance-based learning remains a valuable tool in the machine learning toolkit, particularly in applications that benefit from nuanced and personalized responses.