Introduction to Linear Regression in Machine Learning
Aug 17, 2024
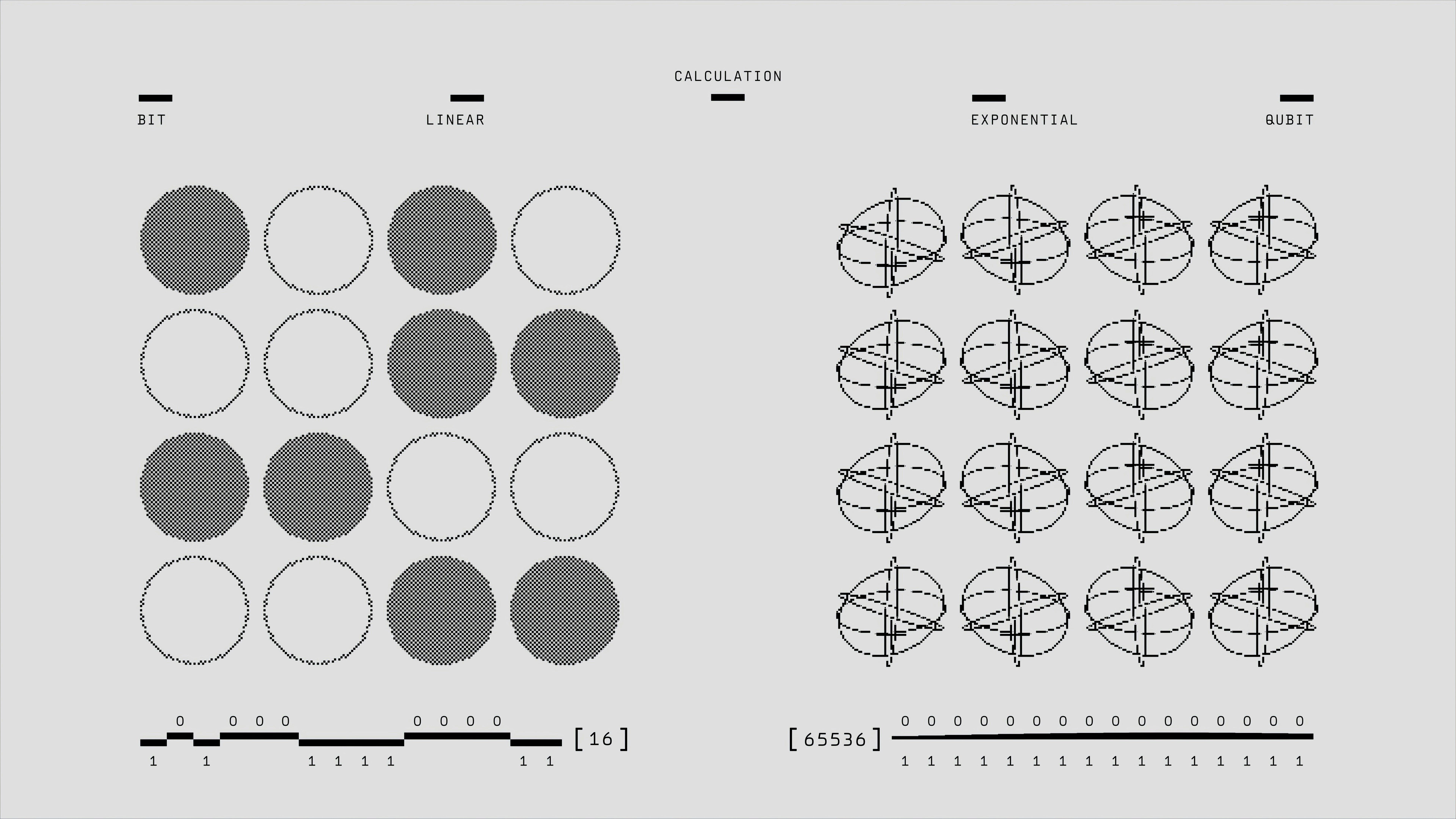
Linear regression is a fundamental machine learning algorithm that has been widely used for many years due to its simplicity, interpretability, and efficiency. It is a type of supervised learning algorithm that predicts a continuous target variable based on one or more independent variables. Linear regression assumes a linear relationship between the dependent and independent variables and uses a linear equation to model this relationship.
In this blog post, we will dive deep into the world of linear regression in machine learning, exploring its key concepts, types, assumptions, evaluation metrics, and implementation in Python. We will also discuss the advantages and disadvantages of linear regression and its applications in various domains.
What is Linear Regression?
Linear regression is a statistical method used in data science and machine learning for predictive analysis. It provides a linear relationship between an independent variable (also known as the predictor or feature) and a dependent variable (also known as the response or target) to predict the outcome of future events.
The equation for simple linear regression is : y=β0+β1x+ where:
$y$ is the dependent variable
$x$ is the independent variable
$\beta_0$ is the y-intercept (the value of $y$ when $x = 0$)
$\beta_1$ is the slope (the change in $y$ for a one-unit change in $x$)
$\epsilon$ is the error term (the difference between the actual and predicted values)
The goal of linear regression is to find the values of $\beta_0$ and $\beta_1$ that minimize the sum of squared differences between the actual and predicted values.
Types of Linear Regression
There are two main types of linear regression:
Simple Linear Regression: This type of regression involves a single independent variable and a single dependent variable. The equation for simple linear regression is : y=β0+β1x
Multiple Linear Regression: This type of regression involves multiple independent variables and a single dependent variable. The equation for multiple linear regression is : y=β0+β1x1+β2x2+...+βnxn where $x_1, x_2, ..., x_n$ are the independent variables and $\beta_1, \beta_2, ..., \beta_n$ are their corresponding coefficients.
Assumptions of Linear Regression
Linear regression makes several assumptions about the data:
Linearity: The relationship between the independent variable(s) and the dependent variable should be linear.
Independence: The residuals (the differences between the actual and predicted values) should be independent of each other.
Homoscedasticity: The variance of the residuals should be constant across all levels of the independent variable(s).
Normality: The residuals should be normally distributed.
Multicollinearity (for multiple linear regression): The independent variables should not be highly correlated with each other.
If these assumptions are violated, the results of the linear regression analysis may not be reliable or accurate.
Evaluation Metrics for Linear Regression
There are several metrics used to evaluate the performance of a linear regression model:
Mean Squared Error (MSE): The average squared difference between the actual and predicted values.
Root Mean Squared Error (RMSE): The square root of the MSE, which has the same units as the dependent variable.
R-squared ($R^2$): The proportion of the variance in the dependent variable that is predictable from the independent variable(s). It ranges from 0 to 1, with higher values indicating better model fit.
Adjusted R-squared: A modified version of $R^2$ that adjusts for the number of predictors in the model.
Mean Absolute Error (MAE): The average absolute difference between the actual and predicted values.
These metrics can be used to compare different linear regression models or to assess the overall fit of a single model.
Python Implementation of Linear Regression
Let's implement a simple linear regression model in Python using the scikit-learn library:
In this example, we first generate some sample data for a simple linear regression problem. We then split the data into training and testing sets using the train_test_split
function from scikit-learn. Next, we create a LinearRegression
object and train the model on the training data using the fit
method. We then make predictions on the testing data using the predict
method.
Finally, we evaluate the model by calculating the mean squared error and R-squared using NumPy functions. The results show that the model has a low MSE and a high R-squared value, indicating a good fit to the data.
Advantages and Disadvantages of Linear Regression
Advantages:
Easy to implement and interpret: Linear regression models are computationally simple and provide an easy-to-interpret mathematical formula to generate predictions.
Scalable: Linear regression models are not computationally heavy and can scale well with increased data volume.
Suitable for online settings: The ease of computation of linear regression models allows them to be used in online settings for real-time predictions.
Disadvantages:
Assumes linearity: Linear regression assumes a linear relationship between the independent and dependent variables, which may not always be true.
Sensitive to outliers: Linear regression is sensitive to outliers in the data, which can significantly affect the model's performance.
Limited to continuous variables: Linear regression is primarily used for predicting continuous target variables and may not be suitable for categorical or discrete variables.
Applications of Linear Regression
Linear regression has a wide range of applications in various domains:
Predicting house prices: Linear regression can be used to predict house prices based on features such as square footage, number of bedrooms, location, and age of the house.
Forecasting sales: Linear regression can be used to forecast future sales based on historical data and other factors such as advertising spending, economic indicators, and seasonal trends.
Estimating exam scores: Linear regression can be used to estimate exam scores based on factors such as study hours, attendance, and previous performance.
Predicting employee attrition: Linear regression can be used to predict employee attrition based on factors such as job satisfaction, salary, and tenure.
Analyzing gene expression data: Linear regression can be used in bioinformatics to analyze gene expression data and identify genes that are differentially expressed under different conditions.
These are just a few examples of the many applications of linear regression in machine learning and data science.
Conclusion
Linear regression is a fundamental machine learning algorithm that has been widely used for many years due to its simplicity, interpretability, and efficiency. It is a valuable tool for understanding relationships between variables and making predictions in a variety of applications. In this blog post, we have explored the key concepts of linear regression, including its types, assumptions, evaluation metrics, and implementation in Python. We have also discussed the advantages and disadvantages of linear regression and its applications in various domains.