Introduction to RNNs in Deep Learning
Aug 14, 2024
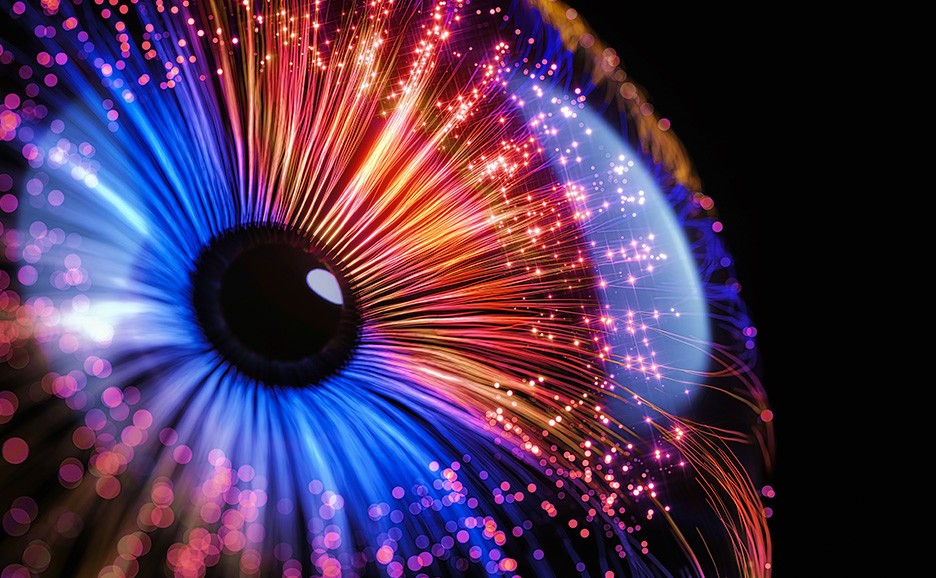
Recurrent Neural Networks (RNNs) are a powerful class of artificial neural networks that excel at processing sequential data. Unlike traditional feedforward neural networks, RNNs have the ability to learn from and incorporate information from previous inputs, making them particularly useful for tasks involving time series, natural language processing, and speech recognition.
In this comprehensive blog post, we will delve into the world of RNNs and explore their applications in deep learning. We will cover the fundamental concepts, architecture, and implementation of RNNs, as well as discuss some of the most popular variants, such as Long Short-Term Memory (LSTM) and Gated Recurrent Units (GRU).
Understanding Recurrent Neural Networks
Sequence Modeling
RNNs are designed to handle sequential data, where each element in the sequence depends on the previous ones. This makes them suitable for tasks such as language modeling, where the probability of a word occurring depends on the context provided by the preceding words in a sentence.
Neurons with Recurrence
The key feature that distinguishes RNNs from feedforward neural networks is the presence of recurrent connections. In a traditional neural network, information flows in a single direction, from the input layer to the output layer. In contrast, RNNs have connections that form a directed cycle, allowing information to flow both forward and backward.
This recurrent structure enables RNNs to maintain a hidden state, which acts as a memory that stores information from previous inputs. At each time step, the RNN processes the current input and updates its hidden state based on the previous hidden state and the current input.
Backpropagation Through Time (BPTT)
Training RNNs involves a modified version of the backpropagation algorithm called Backpropagation Through Time (BPTT). BPTT treats the unrolled RNN as a feedforward neural network and applies the standard backpropagation algorithm to compute the gradients of the loss function with respect to the weights.
However, BPTT can be computationally expensive, especially when dealing with long sequences. As the number of time steps increases, the number of derivatives required for a single weight update also increases. This can lead to the vanishing gradient problem, where gradients become smaller and smaller as they propagate backward through the network, resulting in slow learning or even preventing the network from learning altogether.
Variants of RNNs
To address the vanishing gradient problem and improve the performance of RNNs, several variants have been proposed. Two of the most popular ones are Long Short-Term Memory (LSTM) and Gated Recurrent Units (GRU).
Long Short-Term Memory (LSTM)
LSTMs are a type of RNN specifically designed to tackle the vanishing gradient problem. They introduce a memory cell, which allows the network to selectively remember or forget information over time. The memory cell is controlled by three gates: the forget gate, the input gate, and the output gate.
The forget gate determines what information from the previous hidden state and current input should be retained or discarded. The input gate controls what new information from the current input and previous hidden state should be added to the memory cell. Finally, the output gate decides what information from the memory cell and current input should be used to produce the output.
Here's a simple implementation of an LSTM layer in PyTorch:
Gated Recurrent Units (GRU)
GRUs are another variant of RNNs that aim to solve the vanishing gradient problem. They combine the forget and input gates of an LSTM into a single update gate, and they also have a reset gate that controls how much information from the previous hidden state is combined with the current input.
GRUs have a simpler structure compared to LSTMs, making them computationally more efficient. However, LSTMs generally perform better on tasks that require precise control over the memory.Here's a simple implementation of a GRU layer in PyTorch:
Applications of RNNs in Deep Learning
RNNs have a wide range of applications in deep learning, particularly in domains involving sequential data. Here are some examples:
Natural Language Processing
RNNs are widely used in natural language processing tasks such as language modeling, machine translation, and text generation. They can learn the patterns and dependencies in natural language and generate coherent and contextually relevant text.
Speech Recognition
RNNs, combined with techniques like connectionist temporal classification (CTC), have shown impressive results in speech recognition tasks. They can map audio sequences to text transcriptions, handling the temporal dependencies in speech.
Image Captioning
By combining convolutional neural networks (CNNs) for image understanding with RNNs for sequence generation, researchers have developed models that can generate descriptive captions for images.
Time Series Forecasting
RNNs are well-suited for time series forecasting tasks, where they can learn from past data and make predictions about future values. They have been applied to problems such as stock price prediction, weather forecasting, and traffic flow estimation.
Conclusion
Recurrent Neural Networks are a powerful tool in the deep learning arsenal, particularly for tasks involving sequential data. By incorporating information from previous inputs and maintaining a hidden state, RNNs can learn complex patterns and dependencies in data.
In this blog post, we have explored the fundamental concepts of RNNs, their architecture, and the challenges involved in training them. We have also discussed some of the most popular variants, such as LSTMs and GRUs, and their applications in various domains.