JavaScript Essentials: Removing Duplicates from Arrays and Finding Prime Numbers
Jul 27, 2024
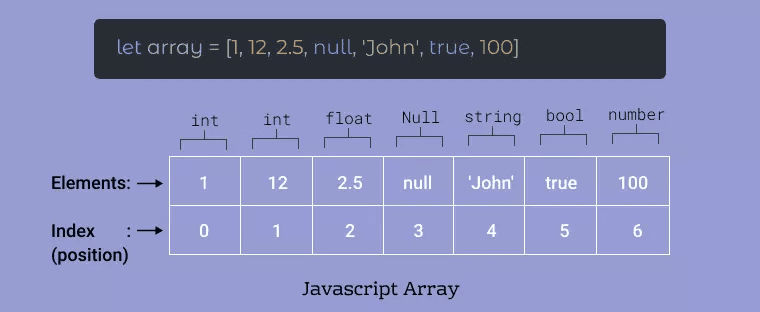
In the world of JavaScript programming, two common tasks arise frequently: removing duplicates from arrays and checking for prime numbers. This blog post will delve into both topics, providing clear explanations, code snippets, and best practices for implementation. By the end, you will have a solid understanding of how to tackle these challenges effectively.
Introduction
JavaScript is a versatile language that powers many applications across the web. Understanding how to manipulate arrays and perform mathematical checks is fundamental for any developer. This post focuses on two specific tasks: removing duplicates from arrays and determining if a number is prime.
Removing Duplicates from Arrays
Why Remove Duplicates?
Duplicates in arrays
can lead to inefficient data handling and can complicate algorithms that rely on unique values. By ensuring that your arrays contain only unique elements, you improve performance and maintain data integrity.
Methods to Remove Duplicates
Here are several effective methods to remove duplicates from an array in JavaScript:
Using Set
The simplest and most efficient way to remove duplicates is by using the Set
object, which only allows unique values.
Using filter() and indexOf()
Another approach involves using thefilter()
method combined withindexOf()
to keep only the first occurrence of each element.
Using reduce()
Thereduce()
method can also be employed to accumulate unique values into a new array.
Using forEach()
You can also use theforEach()
method to iterate through the array and build a new array of unique values.
Removing Duplicates from Objects
When dealing with arrays of objects, you might want to remove duplicates based on a specific property.
Finding Prime Numbers in JavaScript
What is a Prime Number?
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. In other words, a prime number has exactly two distinct positive divisors: 1 and itself.
Methods to Check for Prime Numbers
There are several methods to determine if a number is prime in JavaScript:
Basic Loop Method
The simplest way to check if a number is prime is to iterate through all numbers less than the number itself.
Sieve of Eratosthenes
The Sieve of Eratosthenes is an efficient algorithm to find all prime numbers up to a specified integer.
Conclusion
Understanding how to remove duplicates from arrays and check for prime numbers in JavaScript is crucial for any developer. The methods discussed in this blog post offer a variety of approaches to tackle these common tasks.By using these techniques, you can ensure your data is clean and your mathematical operations are efficient. As you continue to work with JavaScript, these skills will serve as foundational tools in your programming toolkit.