Linear Regression in Python: A Comprehensive Guide
Aug 16, 2024
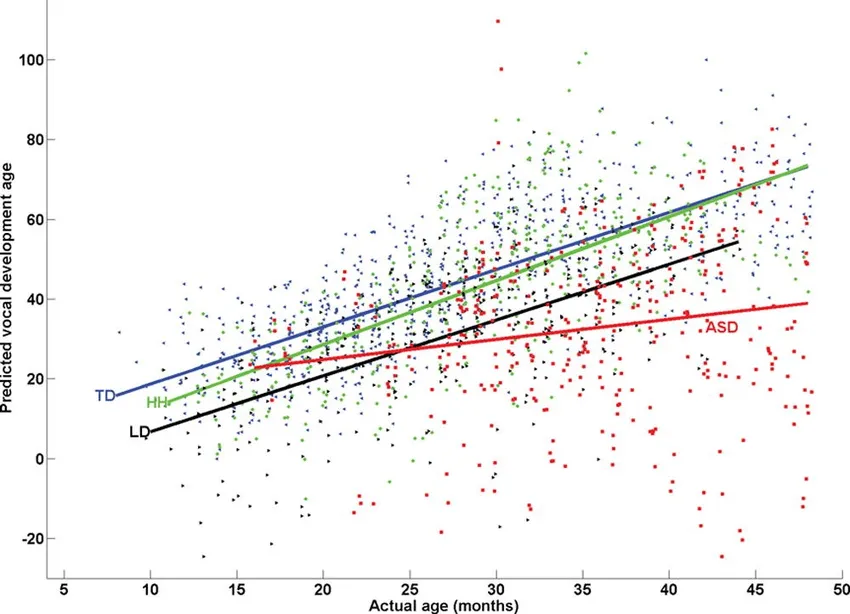
Linear regression is a fundamental statistical technique used extensively in data analysis and machine learning. This blog post will explore the concept of linear regression, its applications, and how to implement it using Python. We will cover both simple and multiple linear regression, utilizing popular libraries such as scikit-learn
and statsmodels
. By the end of this guide, you will have a solid understanding of linear regression in Python and be able to apply it to your own datasets.
What is Linear Regression?
Linear regression is a predictive modeling technique that establishes a relationship between a dependent variable (also known as the target variable) and one or more independent variables (predictors). The goal is to find the best-fitting line through the data points that can be used to make predictions.
The linear regression model can be expressed mathematically as:
y=a0+a1x1+a2x2+...+anxn+ϵ
Where:
yy is the dependent variable.
x1,x2,...,xnx1,x2,...,xn are the independent variables.
a0a0 is the intercept of the regression line.
a1,a2,...,ana1,a2,...,an are the coefficients of the independent variables.
ϵϵ is the random error term.
Applications of Linear Regression
Linear regression is widely used across various fields, including:
Economics: Predicting economic growth based on various indicators.
Healthcare: Estimating patient outcomes based on treatment variables.
Marketing: Forecasting sales based on advertising spend.
Real Estate: Predicting house prices based on features like size and location.
Assumptions of Linear Regression
Before applying linear regression, it's crucial to understand its underlying assumptions:
Linearity: The relationship between the independent and dependent variables should be linear.
Independence: Observations should be independent of each other.
Homoscedasticity: The residuals (errors) should have constant variance at every level of the independent variable.
Normality: The residuals should be approximately normally distributed.
Implementing Linear Regression in Python
In this section, we will walk through the steps to implement linear regression in Python using two popular libraries: scikit-learn
and statsmodels
.
Step 1: Importing Libraries
To get started, we need to import the necessary libraries. Here’s how to do it:
Step 2: Preparing the Data
For demonstration purposes, let's create a simple dataset. In a real-world scenario, you would load your data from a file or a database.
Step 3: Visualizing the Data
Before applying linear regression, it's helpful to visualize the data to understand the relationship between the variables.
Step 4: Splitting the Data
Next, we will split the data into training and testing sets. This allows us to train our model on one subset of the data and evaluate it on another.
Step 5: Implementing Linear Regression with scikit-learn
Now we can create and train our linear regression model usingscikit-learn
.
Step 6: Visualizing Predictions
To visualize how well our model performed, we can plot the predicted values against the actual values.
Conclusion
Linear regression is a powerful tool for predictive modeling and data analysis. In this blog post, we explored the fundamentals of linear regression, its assumptions, and how to implement it in Python using bothscikit-learn
andstatsmodels
. By understanding these concepts, you can leverage linear regression to uncover insights from your data and make informed predictions.