Loops and Threads in JavaScript: Mastering Concurrency and Parallelism
Aug 20, 2024
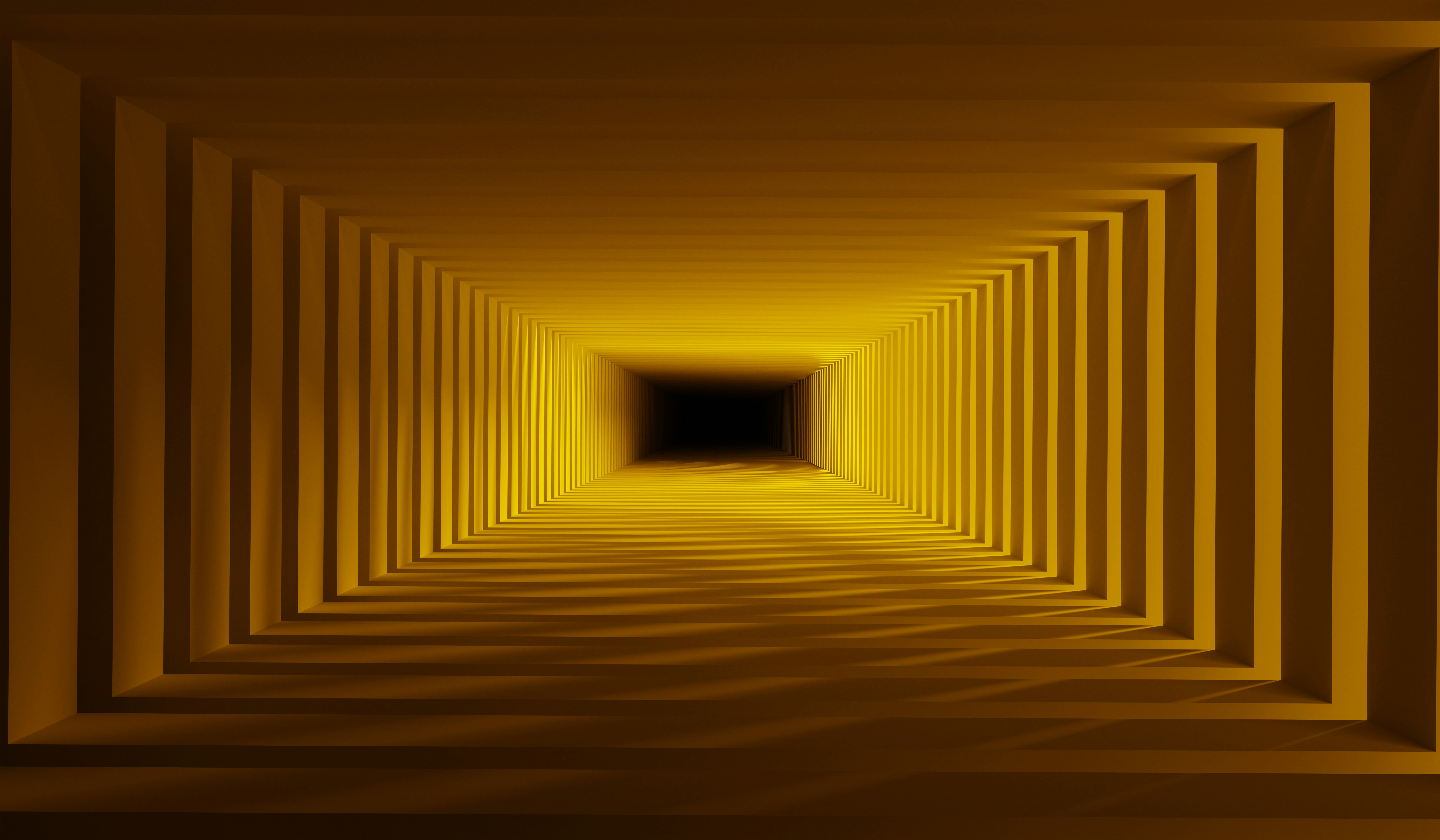
Loops and threads are fundamental concepts in JavaScript that enable developers to write efficient and scalable code. While JavaScript is primarily single-threaded, it provides mechanisms to handle asynchronous operations and achieve concurrency. In this comprehensive guide, we'll explore the intricacies of loops and threads in JavaScript, delving into their usage, best practices, and how they contribute to creating high-performance applications.
Understanding Loops in JavaScript
Loops are a crucial part of any programming language, allowing developers to execute a block of code repeatedly based on a specific condition. JavaScript offers several loop constructs, each with its own characteristics and use cases. Let's dive into the most commonly used loops:
1. for Loop
The for
loop is the most basic and widely used loop in JavaScript. It consists of three parts: initialization, condition, and increment/decrement. Here's an example:
Output :
2. while Loop
Thewhile
loop executes a block of code as long as a specified condition is true. It's useful when you don't know the number of iterations beforehand. Here's an example:
Output
3. do-while Loop
Thedo-while
loop is similar to thewhile
loop, but it executes the block of code at least once, even if the condition is false. Here's an example:
Output
4. for-in Loop
Thefor-in
loop iterates over the enumerable properties of an object. It's commonly used to iterate over the keys of an object. Here's an example:
5. for-of Loop
Thefor-of
loop iterates over the values of an iterable object, such as an array. It provides a more concise way to iterate over arrays compared to traditional loops. Here's an example:
Asynchronous Loops and Promises
JavaScript's asynchronous nature introduces some challenges when using loops with asynchronous operations. To handle this, you can use Promises and theasync/await
syntax. Here's an example of how to iterate over an array of Promises usingPromise.all()
:
In this example, we create an array of Promises that resolve with a delay. By using Promise.all()
, we wait for all Promises to resolve before logging the results.
Threads and Concurrency in JavaScript
JavaScript is primarily single-threaded, meaning it can only execute one task at a time. However, it provides mechanisms to handle asynchronous operations and achieve concurrency. Let's explore some of these concepts:
1. Event Loop
The event loop is the core of JavaScript's asynchronous behavior. It continuously checks the call stack and the task queue, executing tasks when the call stack is empty. The event loop enables JavaScript to handle asynchronous operations without blocking the main thread. Here's a simplified illustration of the event loop:
2. Asynchronous Functions
JavaScript provides several ways to handle asynchronous operations, such as callbacks, Promises, andasync/await
. These constructs allow you to execute tasks asynchronously without blocking the main thread. Here's an example of an asynchronous function usingasync/await
:
In this example, the fetchData()
function uses the async
keyword, allowing it to use the await
keyword inside to wait for the asynchronous fetch()
operation to complete without blocking the main thread.
3. Web Workers
Web Workers provide a way to run scripts in the background without interfering with the main thread. They allow for true parallelism by running code in separate threads. Web Workers communicate with the main thread using messages. Here's a simple example of using a Web Worker:
In this example, we create a new Web Worker and communicate with it using postMessage()
and onmessage
events. The Web Worker runs in a separate thread, allowing for parallel execution.
Best Practices for Loops and Threads in JavaScript
To optimize the performance and maintainability of your code, consider the following best practices when working with loops and threads in JavaScript:
Use the most appropriate loop construct for your use case. Choose between
for
,while
,do-while
,for-in
, andfor-of
based on your specific requirements.Avoid blocking the main thread. When dealing with asynchronous operations, use constructs like Promises,
async/await
, and Web Workers to ensure smooth execution without blocking the main thread.Optimize loop performance. Minimize the number of iterations, avoid unnecessary operations inside the loop, and use
const
instead oflet
if the variable value doesn't change.Handle errors properly. Use
try/catch
blocks to catch and handle errors that may occur during asynchronous operations.Communicate effectively between threads. When using Web Workers, be mindful of the data you send between the main thread and the worker thread to minimize overhead and optimize performance.
Monitor and debug asynchronous code. Use browser developer tools and logging mechanisms to monitor the execution of asynchronous code and debug any issues that may arise.
By following these best practices and understanding the concepts of loops and threads in JavaScript, you can write efficient, scalable, and maintainable code that takes advantage of JavaScript's asynchronous capabilities.
Conclusion
Loops and threads are essential concepts in JavaScript that enable developers to write efficient and scalable code. By mastering the various loop constructs and understanding how to handle asynchronous operations using Promises, async/await
, and Web Workers, you can create high-performance applications that leverage JavaScript's concurrency and parallelism capabilities.
Remember to choose the appropriate loop construct for your use case, optimize loop performance, handle errors properly, communicate effectively between threads, and monitor and debug your asynchronous code. With these practices in mind, you'll be well on your way to writing optimized, efficient, and maintainable JavaScript code.For more information on loops and threads in JavaScript, check out these resources: