Map of the Keys: A Comprehensive Guide to Understanding and Using Key Mapping in Programming
Aug 20, 2024
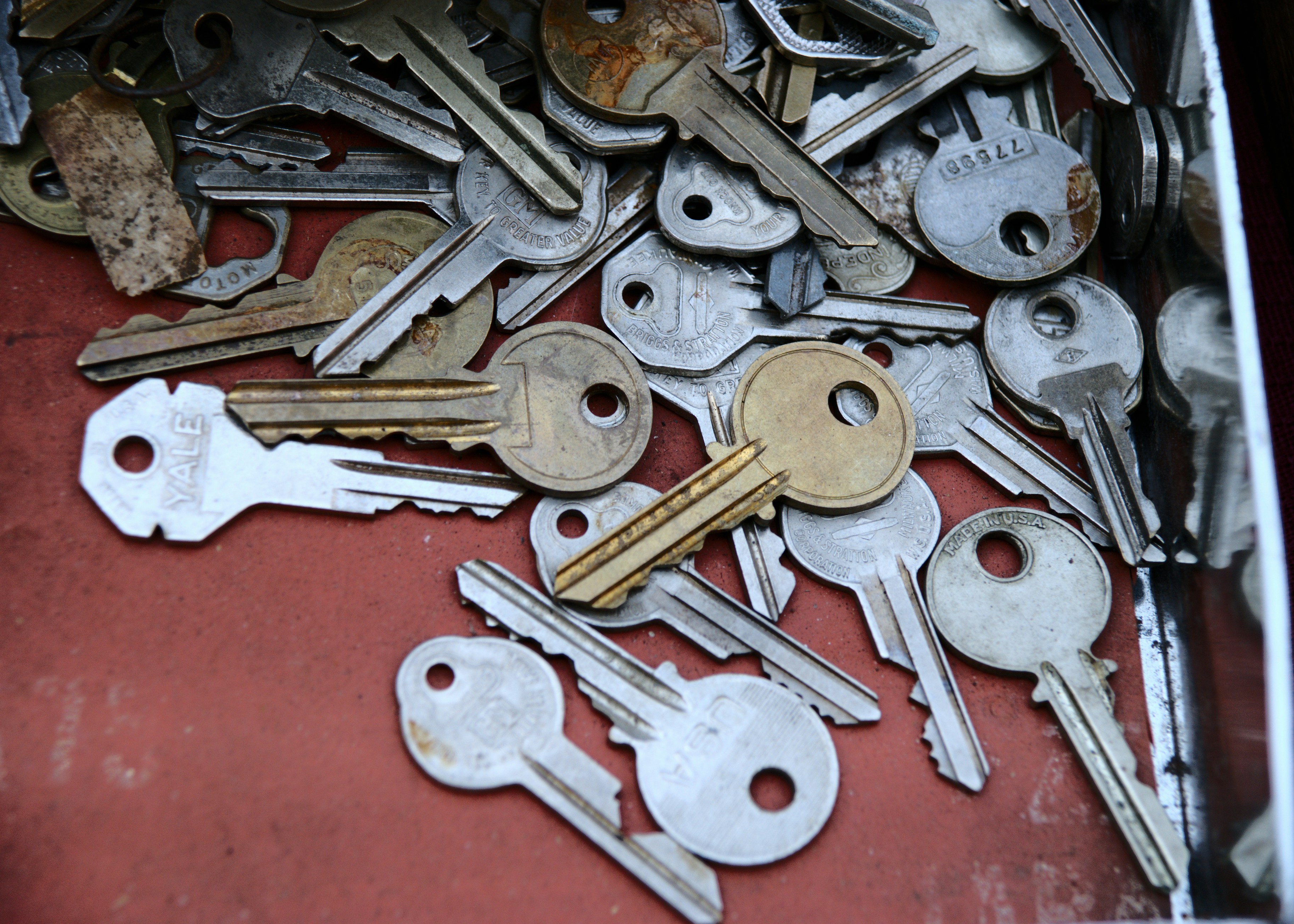
In the realm of programming, the concept of a "map of the keys" plays a crucial role, especially in user interface design and game development. This blog post aims to provide a detailed exploration of key mapping, its significance, and practical applications, along with code snippets to illustrate these concepts.
What is a Map of the Keys?
A map of the keys refers to a data structure that associates keys with specific values. In programming, this concept is often implemented using dictionaries or hash maps, allowing developers to efficiently retrieve, update, and manage the data associated with those keys.
Importance of Key Mapping
Key mapping is essential for various reasons:
User Input Handling: It allows developers to define how different keys on a keyboard or game controller interact with their application.
Customization: Users can customize their controls, enhancing their experience and making applications more accessible.
Efficiency: A well-structured map of keys can speed up data retrieval and processing, optimizing application performance.
Key Mapping in Different Programming Languages
Different programming languages provide various ways to implement a map of the keys. Below, we will explore how to create and use key maps in Python, JavaScript, and C#.
Python: Using Dictionaries
In Python, dictionaries are the primary data structure used for key mapping. Here’s a simple example:
In this example, the key_map
dictionary associates specific keys with their corresponding actions. The perform_action
function retrieves the action based on the key pressed.
JavaScript: Using Objects
In JavaScript, objects can serve as a map of the keys. Here’s how you can implement it:
In this JavaScript example, the keyMap
object maps arrow keys to their respective actions. The performAction
function is triggered on a keydown event, executing the corresponding action.
C#: Using Dictionaries
In C#, dictionaries are also used for key mapping. Here’s a simple implementation:
In this C# example, the keyMap
dictionary maps keys to actions, and the PerformAction
method checks for valid keys and executes the corresponding action.
Advanced Key Mapping Techniques
While the basic implementation of a map of the keys is straightforward, there are advanced techniques that can enhance its functionality.
Dynamic Key Mapping
Dynamic key mapping allows users to customize their key bindings at runtime. This feature is particularly useful in gaming applications where players may want to remap controls. Here’s an example in Python:
Best Practices for Key Mapping
When implementing a map of the keys, consider the following best practices:
Keep It Simple: Avoid overly complex mappings that can confuse users.
Provide Defaults: Always provide default key bindings that make sense for your application.
Allow Customization: Enable users to customize their key bindings to enhance usability.
Document Key Mappings: Provide clear documentation on key mappings to help users understand controls.
Conclusion
Understanding and implementing a map of the keys is essential for creating intuitive and user-friendly applications. By utilizing key mapping effectively, developers can enhance user experience, improve accessibility, and provide customizable controls. Whether you're working in Python, JavaScript, or C#, the principles of key mapping remain consistent.
For more information on key mapping and its applications in programming, you can explore resources such as MDN Web Docs and W3Schools.
By following the guidelines and examples provided in this blog post, you can create robust key mapping systems that cater to your users' needs. Happy coding!