Master JavaScript: All You Need
Aug 22, 2024
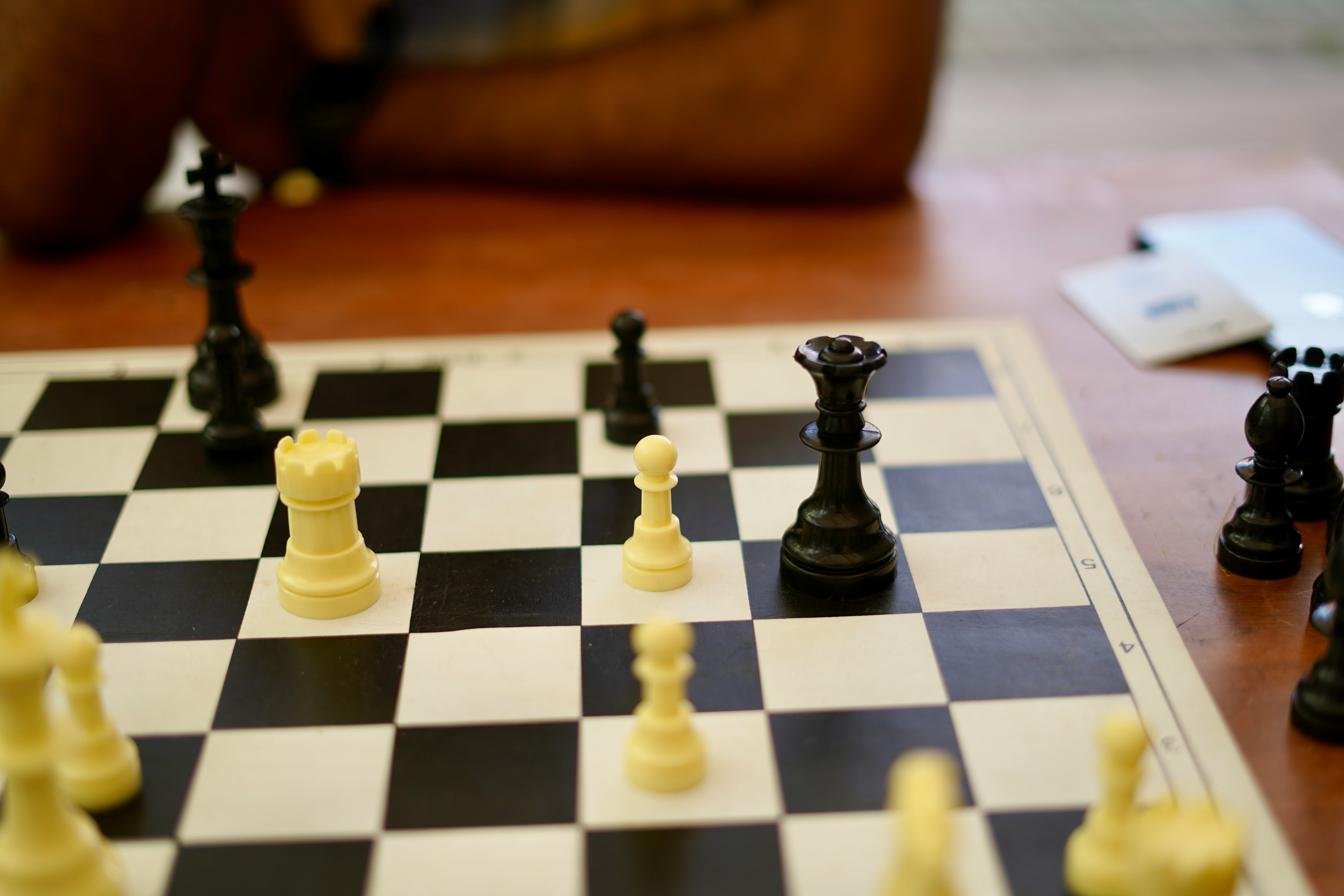
JavaScript is one of the most popular programming languages in the world, powering everything from simple websites to complex web applications. Whether you're a beginner looking to learn the basics or an experienced developer aiming to master JavaScript, this guide will provide you with the insights, techniques, and resources you need to elevate your skills.
Understanding JavaScript: The Basics
JavaScript is a high-level, dynamic, untyped, and interpreted programming language. It is a core technology of the World Wide Web, alongside HTML and CSS, and enables interactive web pages. Here are some fundamental concepts to grasp:
Variables: Used to store data values. In JavaScript, you can declare variables using
var
,let
, orconst
.Data Types: JavaScript has several data types, including:
String: Represents text.
Number: Represents numeric values.
Boolean: Represents true or false.
Object: A complex data structure that can hold multiple values.
Functions: Blocks of code designed to perform a particular task. Functions can be declared using the
function
keyword or as arrow functions.Control Structures: These include loops (
for
,while
) and conditional statements (if
,else
) that control the flow of execution.
Setting Up Your Development Environment
To start mastering JavaScript, you need a proper development environment. Here’s how to set it up:
Text Editor: Choose a text editor like Visual Studio Code, Sublime Text, or Atom. These editors provide syntax highlighting, code completion, and debugging tools.
Browser: Modern browsers like Chrome, Firefox, or Edge come with built-in developer tools that allow you to inspect and debug your JavaScript code.
Node.js: Install Node.js to run JavaScript on your server. It also allows you to use npm (Node Package Manager) to manage libraries and packages.
Core JavaScript Concepts to Master
To truly master JavaScript, you need to delve into its core concepts:
Scope: Understand the difference between global and local scope, and how closures work.
Asynchronous Programming: Learn about callbacks, promises, and async/await to handle asynchronous operations.
Event Handling: Master how to handle events in the browser, such as clicks, form submissions, and keyboard events.
DOM Manipulation: Learn how to interact with and manipulate the Document Object Model (DOM) to create dynamic web pages.
Error Handling: Understand how to handle errors gracefully using
try
,catch
, andfinally
.
Advanced JavaScript Techniques
Once you have a solid grasp of the basics, it's time to explore advanced techniques:
Prototypes and Inheritance: Understand how JavaScript uses prototypes for inheritance and how to create your own objects.
Modules: Learn how to use ES6 modules to organize your code and manage dependencies.
Functional Programming: Explore functional programming concepts such as higher-order functions, map, filter, and reduce.
Design Patterns: Familiarize yourself with common design patterns in JavaScript, such as the Module Pattern, Revealing Module Pattern, and Singleton Pattern.
****JavaScript Frameworks and Libraries
To enhance your JavaScript skills, consider learning popular frameworks and libraries:
React: A JavaScript library for building user interfaces, particularly single-page applications.
Vue.js: A progressive framework for building user interfaces that can also function as a web application framework.
Angular: A platform for building mobile and desktop web applications using TypeScript.
Best Practices for JavaScript Development
To write clean, efficient, and maintainable code, adhere to these best practices:
Code Organization: Structure your code logically and use comments to explain complex sections.
Consistent Naming Conventions: Use meaningful variable and function names to improve readability.
Version Control: Use Git for version control to track changes and collaborate with others.
Testing: Implement unit tests and integration tests to ensure your code works as expected.
Resources for Further Learning
To continue your journey in mastering JavaScript, explore the following resources:
MDN Web Docs: Comprehensive documentation on JavaScript and web technologies.
JavaScript.info: A modern tutorial that covers all aspects of JavaScript.
FreeCodeCamp: Offers a free coding curriculum that includes JavaScript and web development.
Codecademy: An interactive platform that teaches coding through hands-on projects.
****Conclusion
Mastering JavaScript is a journey that requires dedication, practice, and a willingness to learn. By understanding the fundamentals, exploring advanced concepts, and utilizing frameworks, you can become a proficient JavaScript developer. Remember to keep practicing and stay updated with the latest trends and best practices in the JavaScript community.
For more information on specific JavaScript topics, check out resources like MDN Web Docs and JavaScript.info. Happy coding!