Mastering 3D Cube CSS
Jul 28, 2024
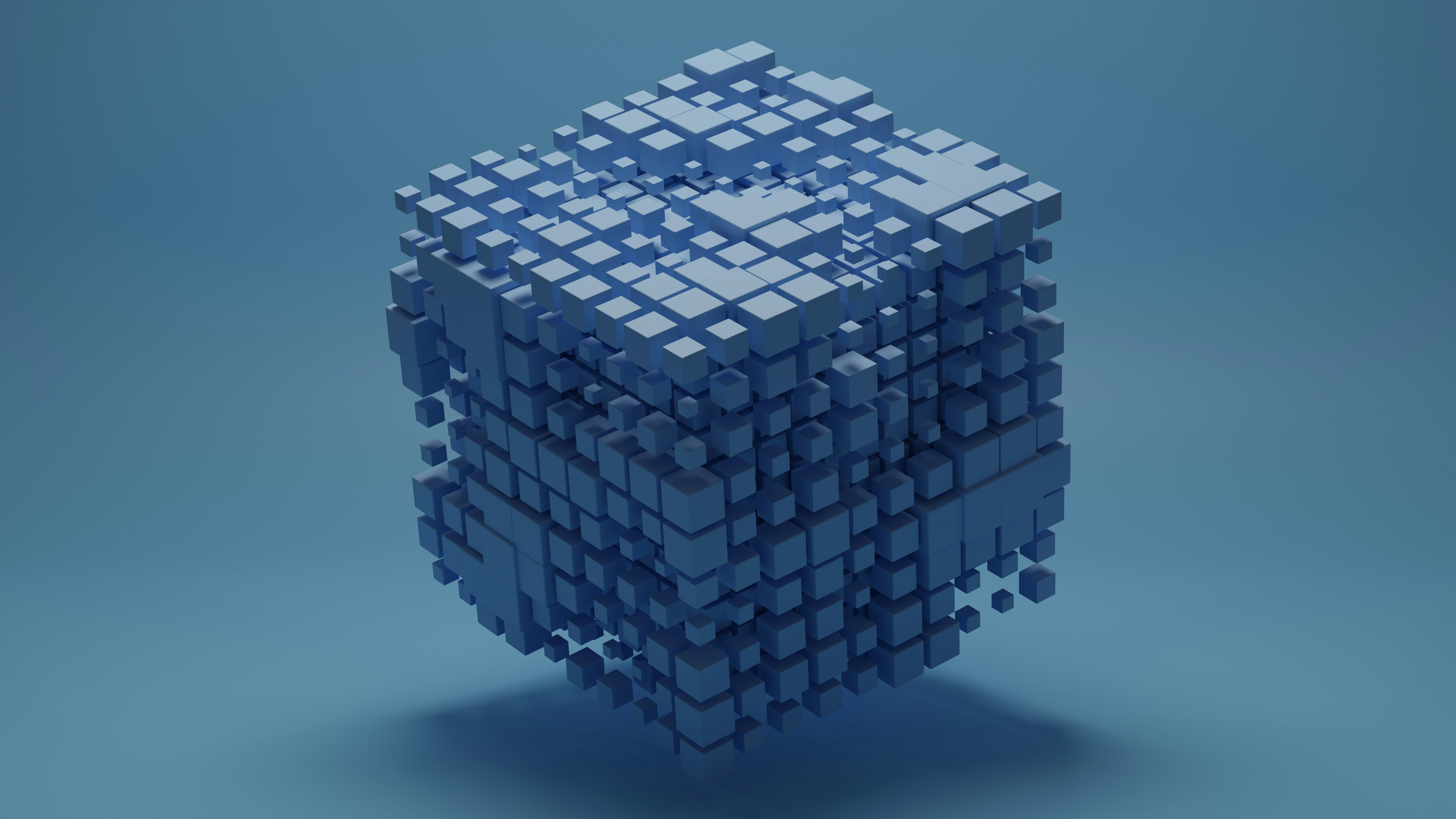
Creating visually appealing web designs is crucial in today's digital landscape, and one of the most captivating elements you can incorporate is a 3D cube using CSS. This blog post will delve into the intricacies of designing and implementing a 3D cube with CSS, complete with practical code snippets and examples. By the end, you'll have a solid understanding of how to create stunning 3D cube effects for your web projects.
What is CSS 3D Cube?
A CSS 3D cube is a three-dimensional representation of a cube created using HTML and CSS. It utilizes CSS properties such as transform
, perspective
, and animation
to create a realistic 3D effect. This technique can enhance user experience by adding depth and interactivity to web applications.
Why Use 3D Cubes in Web Design?
Incorporating 3D cubes in your web design can offer several benefits:
Visual Appeal: 3D cubes can make your website more engaging and visually interesting.
Interactivity: They can respond to user actions, such as mouse movements or clicks, providing an interactive experience.
Showcase Content: 3D cubes can be used to display images, videos, or other content in a dynamic way.
Getting Started: Basic Structure
To create a 3D cube, you need a basic HTML structure. Here’s a simple example:
Styling the Cube with CSS
Next, we will style the cube using CSS. The key properties to focus on aretransform
,perspective
, andtransition
. Here’s how you can achieve this:
Explanation of the CSS Code
Perspective: The
perspective
property on the body element gives depth to the 3D scene. The lower the value, the more pronounced the 3D effect.Transform Style:
preserve-3d
allows child elements to maintain their 3D position.Face Positioning: Each face of the cube is positioned using
translateZ
to move them away from the center of the cube.Animation: The cube is animated to rotate continuously, which can be adjusted by changing the duration in the
animation
property.
Enhancing the Cube: Adding Colors and Shadows
To make the cube more visually appealing, you can add colors and shadows to each face. Here's an updated CSS snippet:
Interactive 3D Cube: User Interaction
To create an interactive experience, you can use JavaScript to allow users to control the cube's rotation with mouse movements. Here’s a simple implementation:
Explanation of the JavaScript Code
Event Listener: The
mousemove
event listener captures the mouse position on the screen.Rotation Calculation: The mouse position is normalized to create a rotation effect that feels natural as the user moves the mouse.
Dynamic Transform: The cube's rotation is updated in real-time based on the mouse position.
Resources for Further Learning
To enhance your knowledge and skills in CSS 3D transformations, consider exploring the following resources:
CSS Tricks
: Comprehensive guides and tutorials on CSS techniques.CodePen
: A platform to experiment with and share your CSS cube designs.MDN Web Docs
: Official documentation for CSS properties and their usage.