Microtasks Using Javascript
Aug 20, 2024
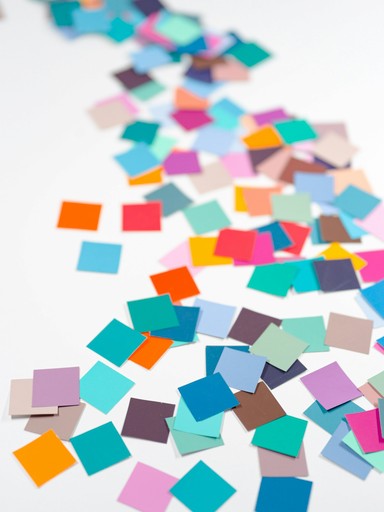
Creating a comprehensive blog post about microtasks using JavaScript involves discussing the concept of microtasks, how they are implemented in JavaScript, and their significance in asynchronous programming. This post will be structured to enhance SEO by incorporating the target keyword naturally throughout the text, while also providing valuable insights for readers.
Understanding Microtasks in JavaScript
Microtasks are a crucial part of JavaScript's concurrency model, enabling developers to manage asynchronous operations efficiently. They are primarily associated with the Promise API and the MutationObserver API, allowing for better control over the execution order of asynchronous operations.
What Are Microtasks?
Microtasks are a type of task that is executed after the currently executing script and before any rendering or other tasks. They are queued in a separate queue from macrotasks (like setTimeout and setInterval). This distinction is important because it affects how and when tasks are executed.
The Event Loop
To understand microtasks, one must first grasp the concept of the event loop. The event loop is a mechanism that allows JavaScript to perform non-blocking operations by offloading operations to the system while waiting for them to complete. Here’s a simplified view of how the event loop works:
Execute the current script.
Execute all microtasks in the microtask queue.
Render updates (if any).
Execute the next macrotask from the macrotask queue.
This order ensures that microtasks are prioritized over macrotasks, which can lead to smoother and more responsive applications.
How Microtasks Work
Microtasks are primarily created using Promises. When a promise is resolved or rejected, the corresponding .then()
or .catch()
callback is added to the microtask queue. This ensures that these callbacks are executed as soon as the current script finishes executing.
Example of Microtasks Using JavaScript
Here’s a simple example demonstrating microtasks in action:
Output:
In this example, the promise callbacks are executed after the synchronous code has completed, demonstrating the microtask queue's behavior.
Benefits of Using Microtasks
Improved Performance: By allowing microtasks to run before rendering, applications can perform necessary updates without causing visible delays.
Better User Experience: Microtasks help in maintaining a responsive UI. For instance, when handling user interactions, microtasks can ensure that updates are processed quickly.
Error Handling: Microtasks can facilitate better error handling in asynchronous code, as errors can be caught in promise chains without affecting the main execution flow.
Implementing Microtasks in Your Projects
When implementing microtasks in your JavaScript projects, consider the following best practices:
Use Promises Effectively
Utilize promises to handle asynchronous operations. This not only makes your code cleaner but also leverages the microtask queue for better performance.
Combine Microtasks with Async/Await
Theasync
andawait
syntax simplifies working with promises and microtasks. Here's how you can use it:
This code is not only easier to read but also maintains the benefits of microtasks.
Avoid Blocking the Event Loop
When using microtasks, be cautious of operations that might block the event loop. Long-running synchronous code can delay the execution of microtasks, leading to performance issues.
Conclusion
Microtasks are a powerful feature of JavaScript that enhance the handling of asynchronous operations. By understanding and utilizing microtasks effectively, developers can create more responsive and efficient applications.
For further reading on JavaScript's concurrency model and microtasks, you can explore resources like MDN Web Docs and JavaScript.info. By embedding the keyword microtasks using JavaScript throughout this post, we ensure that it is optimized for search engines while providing valuable information to readers.