Multi-Line String in JSX: A Comprehensive Guide
Aug 9, 2024
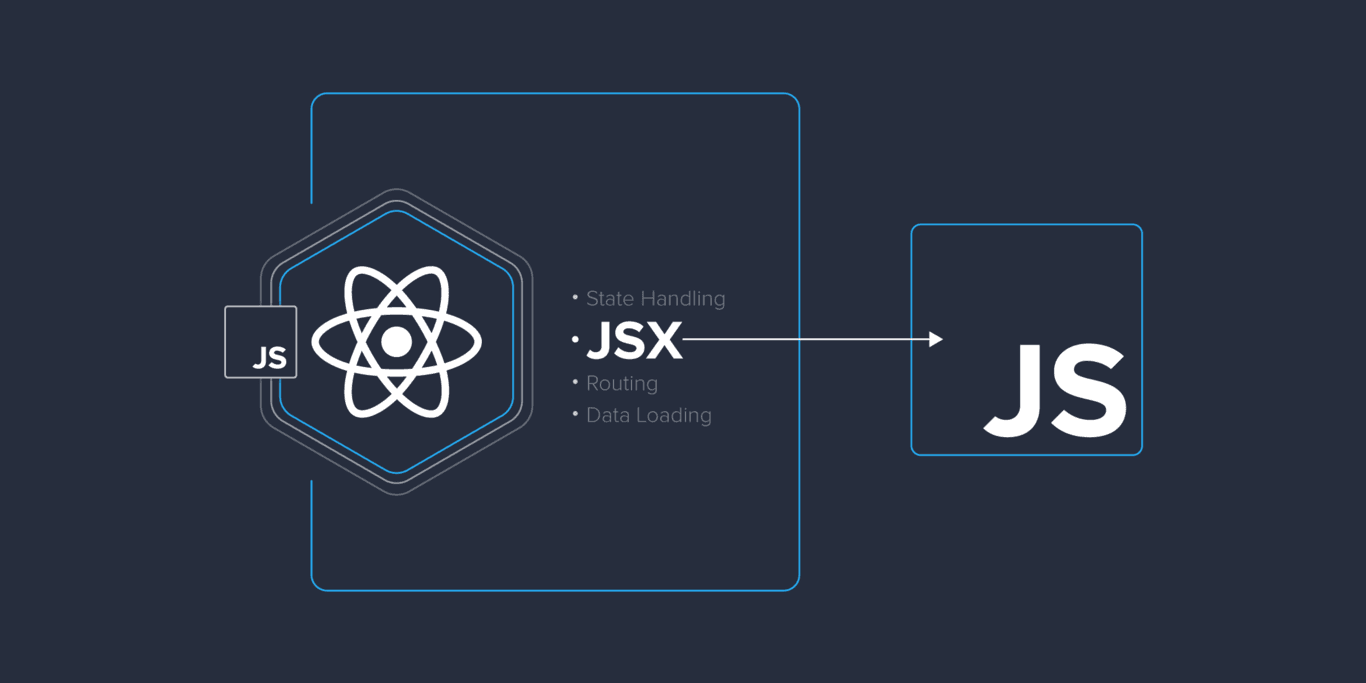
When working with JSX in React, managing strings effectively is crucial for creating dynamic and user-friendly applications. One common requirement is the ability to handle multi-line strings. This guide will explore how to implement multi-line strings in JSX using template literals, string interpolation, and JavaScript strings. We will also provide code snippets and examples to illustrate these concepts.
Understanding Multi-Line Strings in JavaScript
JavaScript provides several ways to create strings, but multi-line strings can be particularly tricky. Traditionally, multi-line strings
were created using escape characters, which could lead to less readable code. However, with the introduction of template literals in ES6 (ES2015), developers can now use backticks to create strings that span multiple lines without the need for escape characters.
Template Literals
Template literals are enclosed by backticks (`
) and allow for easy multi-line string creation and string interpolation. Here’s how you can create a multi-line string using template literals:
This will output:
How to Show Value in String JS
In many cases, you may want to include dynamic values within your strings. This is where string interpolation comes in handy. By using template literals, you can easily embed expressions within your strings.
Example of String Interpolation
Using Multi-Line Strings in JSX
When working with JSX, you can leverage template literals to create multi-line strings that can be rendered in your components. Here’s how to do it effectively.
Basic Example
In a React component, you can use a template literal to create a multi-line string and render it directly:
However, rendering multi-line text directly like this will not preserve the line breaks in HTML. To achieve this, you can use the white-space
CSS property or convert the line breaks to <br />
tags.
Preserving Line Breaks with CSS
You can use CSS to ensure that line breaks are preserved when rendering multi-line strings:
In this example, the whiteSpace: 'pre-line'
style will preserve the line breaks when the text is rendered.
Converting Line Breaks to <br />
Tags
If you prefer to convert line breaks to HTML <br />
tags, you can use the following approach:
In this case, we split the string at each newline character and map over the resulting array to render each line followed by a <br />
tag.
Conclusion
Handling multi-line strings in JSX can significantly enhance the readability and maintainability of your code. By utilizing template literals, you can easily create multi-line strings and incorporate dynamic values using string interpolation. Whether you choose to preserve line breaks with CSS or convert them to <br />
tags, the techniques outlined in this guide will help you effectively manage strings in your React applications.