Next.js Interview Questions
Jul 24, 2024
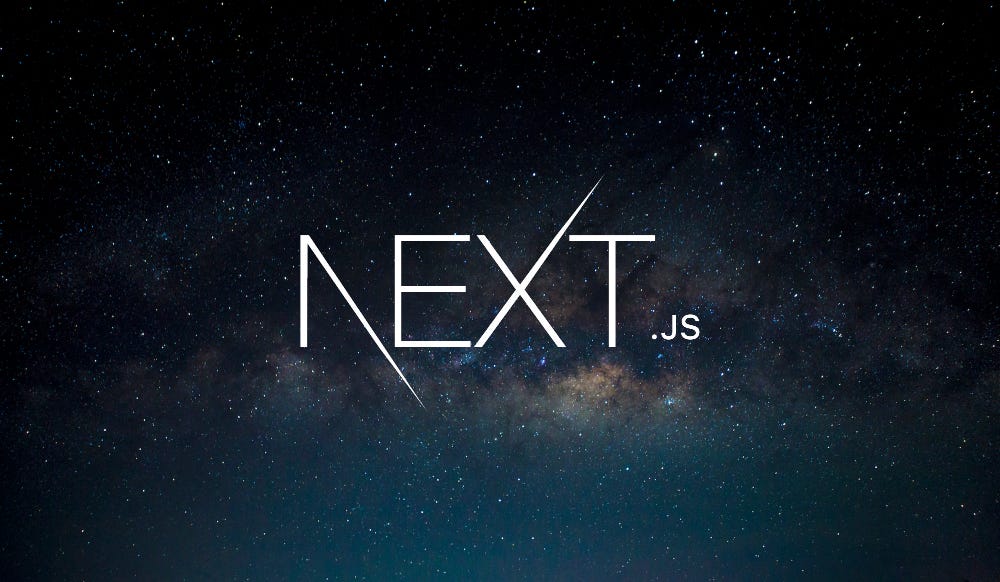
Next.js has emerged as one of the most popular frameworks for building server-side rendered (SSR) React applications. As the demand for Next.js developers continues to grow, so does the need for candidates to prepare for interviews effectively. This blog post provides a comprehensive list of 50 Next.js interview questions, along with detailed answers and code examples to help you ace your next interview.
Key Features of Next.js
Server-Side Rendering (SSR): Renders pages on the server for improved SEO and performance.
Static Site Generation (SSG): Pre-renders pages at build time for faster load times.
API Routes: Allows you to create serverless functions directly in your Next.js application.
Automatic Code Splitting: Loads only the necessary code for each page, improving performance.
Built-in CSS and Sass Support: Enables easy styling of components.
Basic Next.js Interview Questions
1. What is Next.js?
Answer: Next.js is a Reactframework thatenables server-side renderingand static sitegeneration forbuilding webapplications. It is designedto optimize performanceand improve SEO.
2. How do you install Next.js?
Answer: Youcan install Next.js by runningthe followingcommand in yourterminal:
3. What are the advantages of using Next.js?
Answer: Advantagesof Next.js include:
Improved SEO through server-side rendering.
Faster page loads with static site generation.
Automatic code splitting for optimized performance.
Built-in support for API routes.
4. Explain the difference between server-side rendering and static site generation.
Answer:Server-side rendering(SSR) generatesHTML on eachrequest, whilestatic site generation(SSG) pre-renders HTMLat build time. SSR is idealfor dynamic content, while SSG isbest for staticcontent.
5. What is the purpose of getStaticProps
?
Answer:getStaticProps
is a Next.js function usedto fetch dataat build timefor static generation. It allows youto pass dataas props to yourcomponent.
6. What is the purpose of getServerSideProps
?
Answer:getServerSideProps
isused for server-side rendering, fetching dataon each requestand passing itas props to thecomponent.
7. How do you create API routes in Next.js?
Answer:You can createAPI routes byadding JavaScript filesin thepages/api
directory. For example, to create anAPI route forfetching userdata:
8. What is the purpose of the Link
component in Next.js?
Answer:TheLink
componentis used for client-side navigationbetween pagesin a Next.js application. It prefetches thelinked page forfaster loading.
9. How do you handle dynamic routing in Next.js?
Answer: Dynamicrouting in Next.js can be handledby using squarebrackets in thefile name. Forexample, to createa dynamic routefor user profiles:
10. What are CSS Modules in Next.js?
Answer: CSSModules allowyou to writescoped CSS thatapplies onlyto the componentwhere it is imported, preventing globalnamespace conflicts.
css
Intermediate Next.js Interview Questions
11. How do you implement global styles in Next.js?
Answer: Toimplement globalstyles, createastyles/globals.css
fileand import itin the_app.js
file.
12. Explain the concept of automatic code splitting in Next.js.
Answer:Automatic codesplitting allowsNext.js to loadonly the JavaScript necessaryfor the pagebeing rendered, improving loadtimes and performance.
13. What is the purpose of the _document.js
file in Next.js?
Answer:The_document.js
fileis used to customizethe HTML documentstructure. Itallows you tomodify the<head>
and<body>
tags.
14. How do you handle authentication in Next.js?
Answer:Authenticationcan be handledusing librarieslike NextAuth.js orby implementingcustom authenticationlogic with APIroutes and cookies.
15. What is the next/image
component used for?
Answer: Thenext/image
componentprovides an optimizedway to serveimages with featureslike lazy loadingand automaticresizing.
16. How do you enable TypeScript in a Next.js project?
Answer: Toenable TypeScript, createatsconfig.json
filein your projectroot and installTypeScript alongwith the necessarytypes:
17. Explain the concept of middleware in Next.js.
Answer:Middleware inNext.js allowsyou to run codebefore a requestis completed. It can be usedfor authentication, logging, andmodifying requests.
18. How do you deploy a Next.js application?
Answer: Youcan deploy aNext.js applicationusing platformslike Vercel, Netlify, or AWS. Thebasic steps includebuilding theapplication anduploading theoutput to thehosting provider.
19. What is the difference between getStaticPaths
and getStaticProps
?
Answer:getStaticPaths
isused to specifydynamic routesthat should bepre-rendered atbuild time, whilegetStaticProps
fetches data for thoseroutes.
20. How can you optimize performance in a Next.js application?
Answer: Performancecan be optimizedby:
Using static site generation (SSG) where possible.
Implementing lazy loading for images and components.
Utilizing caching strategies.
Minimizing bundle size with code splitting.
Advanced Next.js Interview Questions
21. What is the purpose of next.config.js
?
Answer: Thenext.config.js
fileis used to customizethe Next.js configuration, such as enablingexperimentalfeatures, settingenvironment variables, and modifyingwebpack settings.
22. How do you implement internationalization (i18n) in Next.js?
Answer: Next.js supports internationalization throughthei18n
configurationinnext.config.js
, allowingyou to definelocales and defaultlanguage settings.
23. Explain the use of getInitialProps
.
Answer:getInitialProps
is a methodused for datafetching in Next.js pages. Itruns on the serverduring SSR andon the clientduring navigation.
24. How do you handle errors in Next.js?
Answer: Youcan handle errorsin Next.js bycreating a custom_error.js
pageto display errormessages or usingerror boundariesin React.
25. What is the role of next/head
?
Answer: Thenext/head
componentallows you tomodify the<head>
sectionof your HTMLdocument, enablingyou to set metatags, titles, and links.
26. How do you implement A/B testing in Next.js?
Answer:A/B testing canbe implementedusing featureflags or third-party tools likeGoogle Optimizeto serve differentversions of apage to users.
27. What are the different methods for data fetching in Next.js?
Answer: Themain methodsfor data fetchingin Next.js include:
getStaticProps
: For static generation.getServerSideProps
: For server-side rendering.Client-side fetching with libraries like Axios or Fetch API.
28. How do you use environment variables in Next.js?
Answer: Environmentvariables canbe defined ina.env.local
fileand accessedin your applicationusingprocess.env.VARIABLE_NAME
.
29. What is the purpose of the next/head
component?
Answer: Thenext/head
componentis used to managethe documenthead, allowingyou to set titles, meta tags, andother elementsdynamically.
30. How do you implement custom 404 pages in Next.js?
Answer: Youcan create acustom 404 pageby adding a404.js
filein thepages
directory. This page willbe rendered whena user navigates to a non-existent route.
31. Explain the concept of static site generation (SSG).
Answer: Staticsite generation(SSG) is a methodof pre-rendering pagesat build time, resulting infaster load timesand improvedSEO.
32. How do you handle real-time data in Next.js?
Answer:Real-time datacan be handledusing WebSockets orlibraries likeSocket.io toestablish a persistentconnection betweenthe client andserver.
33. What is the difference between SSR and CSR?
Answer: Server-side rendering(SSR) generatesHTML on the serverfor each request, while client-side rendering(CSR) generatesHTML in the browserusing JavaScript.
34. How do you implement pagination in Next.js?
Answer:Pagination canbe implementedby fetching asubset of databased on thecurrent pagenumber and renderingthe appropriateitems.
35. What are some best practices for optimizing Next.js applications?
Answer: Bestpractices include:
Using static generation where possible.
Minimizing bundle size.
Leveraging caching strategies.
Implementing lazy loading for images and components.
36. How do you use Redux with Next.js?
Answer: Reduxcan be integratedwith Next.js bycreating a Reduxstore and usingtheProvider
componentto wrap yourapplication, allowingaccess to thestore in allcomponents.
37. What is the purpose of the next/script
component?
Answer: Thenext/script
componentis used to loadexternal scriptsin a way thatoptimizes performanceand ensures thatscripts are loadedin the correctorder.
38. How do you implement a custom server in Next.js?
Answer: Youcan create acustom serverusing Node.js andExpress, allowingyou to definecustom routesand middleware.
Happy Coding!