Recurrent Neural Networks: A Comprehensive Guide
Aug 14, 2024
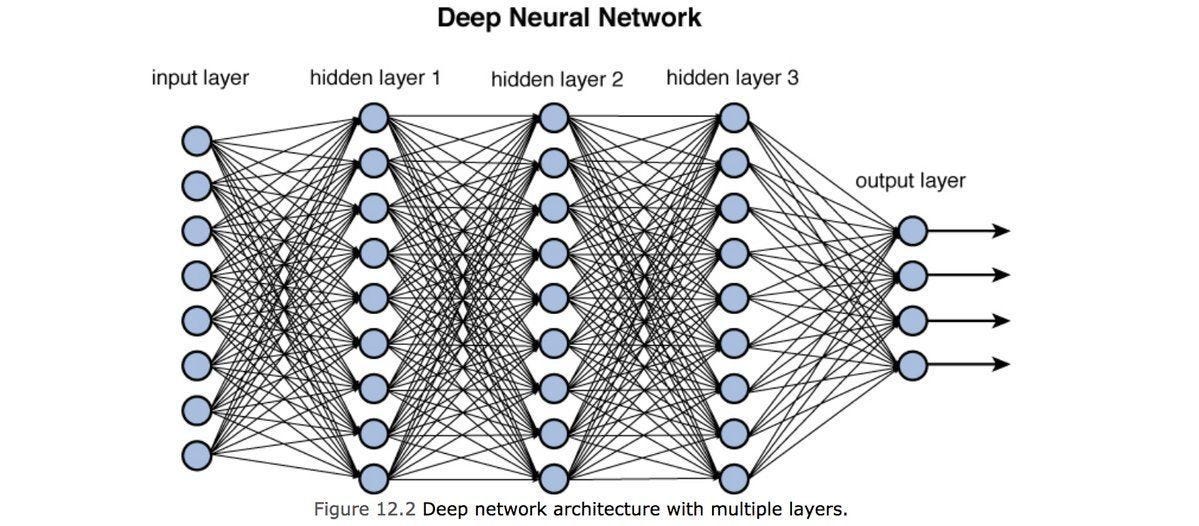
Recurrent Neural Networks (RNNs) are a powerful class of neural networks designed to process sequential data. They are particularly adept at tasks where the order of the data points is significant, such as time series analysis, natural language processing, and speech recognition. This blog post will explore the architecture, working principles, advantages, challenges, and applications of RNNs, along with code snippets to illustrate their implementation.
What is a Recurrent Neural Network?
A Recurrent Neural Network is a type of artificial neural network where connections between nodes can create cycles, allowing information to persist. Unlike traditional feedforward neural networks, RNNs can use their internal memory to process sequences of inputs. This unique feature makes RNNs suitable for tasks where context and order matter.
How Do Recurrent Neural Networks Work?
RNNs function by maintaining a hidden state that captures information about previous inputs. At each time step, the network receives an input and updates its hidden state based on the current input and the previous hidden state. The basic equations governing RNNs are:
ht=σ(Whht−1+Wxxt+b)
yt=Wyht+by
Where:
htht is the hidden state at time tt.
xtxt is the input at time tt.
WhWh, WxWx, and WyWy are weight matrices.
bb and byby are biases.
σσ is an activation function (often tanh or ReLU).
This architecture allows RNNs to learn from sequences by passing information from one time step to the next.
Types of Recurrent Neural Networks
RNNs can be categorized into several types based on the input-output relationship:
One-to-One: Standard neural network structure with one input and one output.
One-to-Many: One input leads to multiple outputs, useful in tasks like image captioning.
Many-to-One: Multiple inputs are summarized into a single output, commonly used in sentiment analysis.
Many-to-Many: Multiple inputs produce multiple outputs, such as in machine translation.
Challenges with RNNs
Despite their advantages, RNNs face significant challenges:
Vanishing Gradient Problem: During training, gradients can become very small, making it difficult for the network to learn long-range dependencies.
Exploding Gradient Problem: Conversely, gradients can also grow too large, leading to unstable training.
To address these issues, advanced architectures like Long Short-Term Memory (LSTM) networks and Gated Recurrent Units (GRUs) have been developed.
Long Short-Term Memory (LSTM) Networks
LSTMs are a type of RNN specifically designed to overcome the vanishing gradient problem. They introduce a memory cell that can maintain information over long periods. The key components of an LSTM cell include:
Forget Gate: Decides what information to discard from the cell state.
Input Gate: Determines what new information to store in the cell state.
Output Gate: Controls the output based on the cell state.
The equations governing an LSTM cell are:
ft=σ(Wf⋅[ht−1,xt]+bf)
it=σ(Wi⋅[ht−1,xt]+bi)
C~t=tanh(WC⋅[ht−1,xt]+bC)
Ct=ft∗Ct−1+it∗C~t
ot=σ(Wo⋅[ht−1,xt]+bo)
ht=ot∗tanh(Ct)
Where ftft, itit, otot are the forget, input, and output gates, respectively, and CtCt is the cell state.
Gated Recurrent Units (GRUs)
GRUs are a simpler alternative to LSTMs, combining the forget and input gates into a single update gate. This reduces the complexity while maintaining performance. The equations for a GRU are:
zt=σ(Wz⋅[ht−1,xt]+bz)
rt=σ(Wr⋅[ht−1,xt]+br)
h~t=tanh(Wh⋅[rt∗ht−1,xt]+bh)
ht=(1−zt)∗ht−1+zt∗h~t
Where ztzt is the update gate and rtrt is the reset gate.
Applications of Recurrent Neural Networks
RNNs have a wide range of applications, including:
Natural Language Processing: Tasks like text generation, sentiment analysis, and machine translation.
Speech Recognition: Converting spoken language into text.
Time Series Prediction: Forecasting stock prices, weather conditions, and other time-dependent phenomena.
Image Captioning: Generating textual descriptions for images.
Music Generation: Composing music by learning from existing pieces.
Implementing a Simple RNN in Python
Here’s a basic example of how to implement a simple RNN using Python and TensorFlow/Keras:
Conclusion
Recurrent Neural Networks are a vital tool in the field of machine learning, particularly for tasks involving sequential data. Their ability to maintain internal memory allows them to excel in applications ranging from natural language processing to time series prediction. Despite challenges like the vanishing gradient problem, advancements such as LSTMs and GRUs have made RNNs more robust and effective.
As the field of deep learning continues to evolve, RNNs will likely remain a fundamental component in tackling complex sequential tasks, paving the way for innovative applications in various domains.