Rotating Arrays in JavaScript: A Comprehensive Guide
Jul 29, 2024
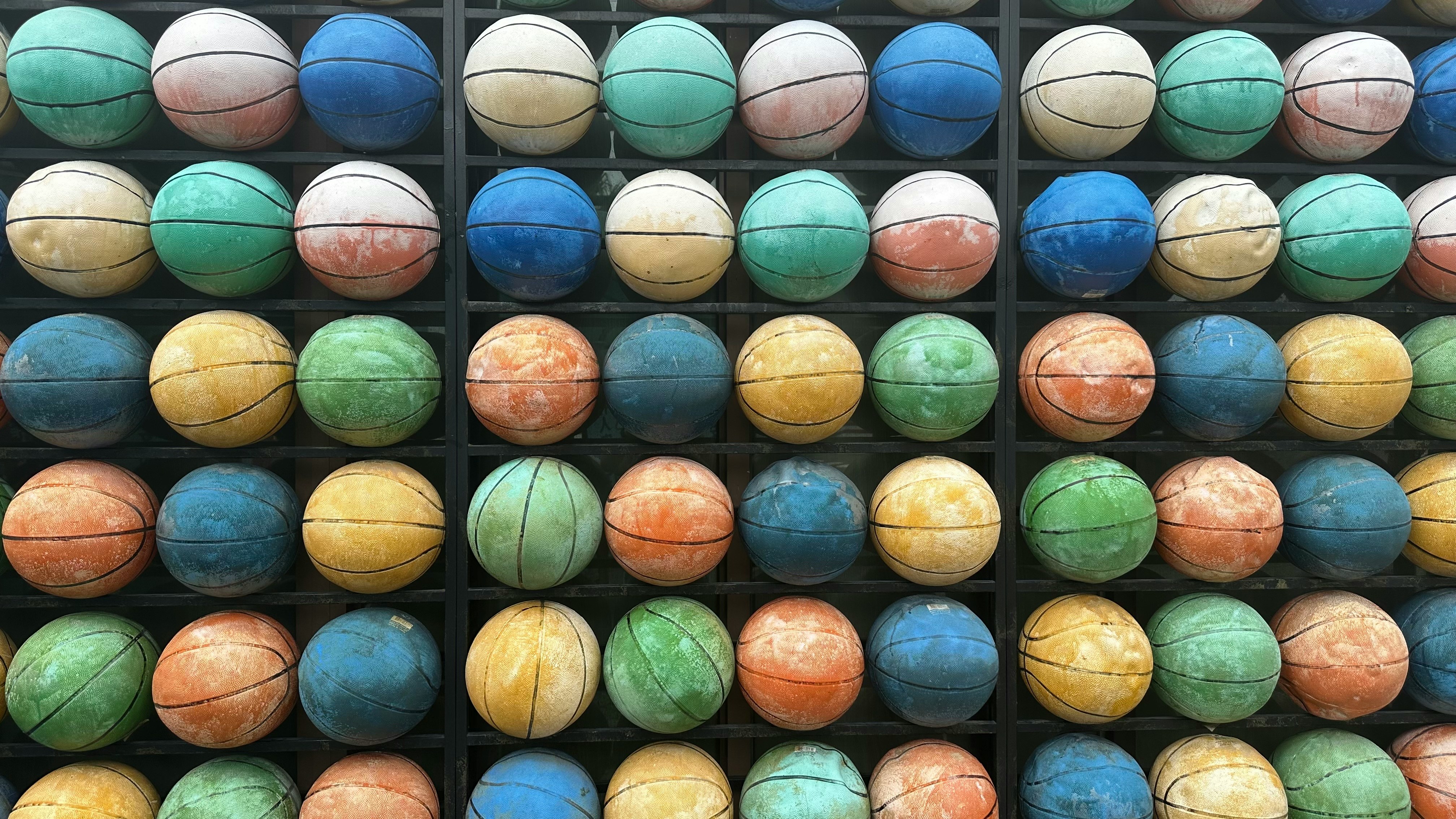
Rotating arrays
is a common task in programming, especially in JavaScript. Whether you're working on algorithms, data manipulation, or simply trying to enhance your coding skills, understanding how to rotate an array is essential. In this blog post, we will explore various methods to rotate an array in JS, providing code snippets and explanations for each approach.
What Does Rotating an Array Mean?
Rotating an array involves shifting its elements either to the left or to the right. For example, given an array [1, 2, 3, 4, 5]
, rotating it to the right by one position results in [5, 1, 2, 3, 4]
. Conversely, rotating it to the left by one position results in [2, 3, 4, 5, 1]
.
Why Rotate an Array?
Rotating arrays can be useful in various scenarios, including:
Implementing
circular queues
Solving problems in competitive programming
Manipulating data for visualizations
Methods to Rotate an Array in JavaScript
We will discuss several methods to rotate an array in JS, including using built-in array methods, slicing, and more. Each method will include a code snippet for better understanding.
Method 1: Using pop()
and unshift()
This method involves removing the last element of the array and adding it to the front.
Method 2: Using shift()
and push()
In this approach, we remove the first element and add it to the end of the array.
Method 3: Using slice()
and concat()
This method involves slicing the array into two parts and concatenating them in the desired order.
Method 4: Using splice()
Thesplice()
method can also be used to rotate an array by removing elements and adding them back in the desired order.
Method 5: Using Array Reversal
This is an efficient method that involves reversing parts of the array.
Performance Considerations
When choosing a method torotate an array in JS, consider the following:
Time Complexity: Most methods discussed have a time complexity of O(n), where n is the number of elements in the array.
Space Complexity: Methods using
slice()
andconcat()
create new arrays, which may not be optimal for large datasets.
Conclusion
Rotating arrays is a fundamental operation in JavaScript that can be implemented in various ways. Understanding these methods not only enhances your coding skills but also prepares you for technical interviews and real-world problem-solving.By practicing these techniques, you can become proficient in manipulating arrays, a crucial aspect of JavaScript programming. Whether you choose to use built-in methods or implement your own logic, the ability torotate an array in JSwill undoubtedly serve you well in your coding journey.