Simple Promise JS
Aug 20, 2024
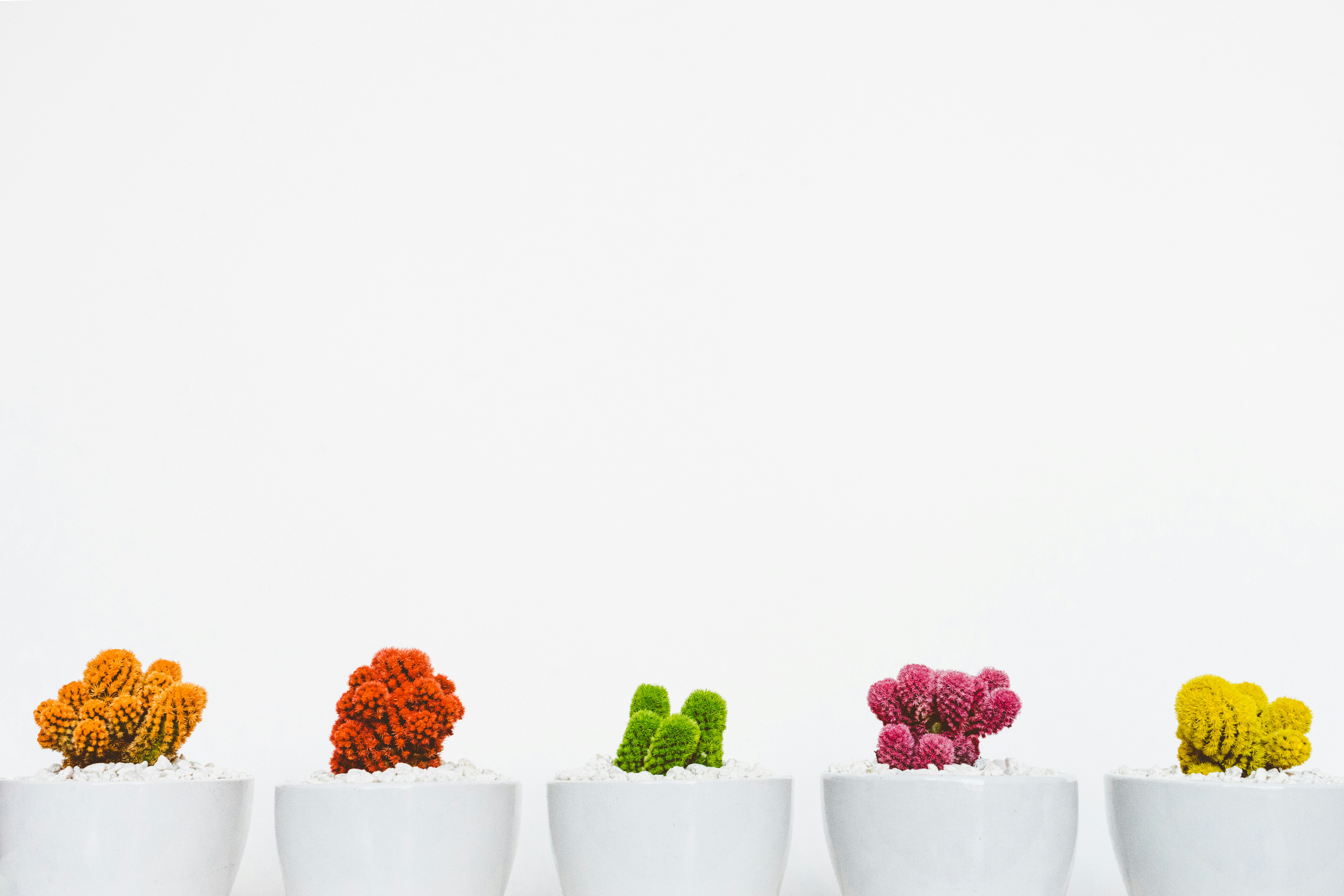
JavaScript is known for its asynchronous capabilities, which allow developers to handle tasks like API calls without blocking the main thread. One of the most effective ways to manage asynchronous operations is by using Promises. A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
What is a Promise?
A Promise can be in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully.
Rejected: The operation failed.
This mechanism allows developers to write cleaner, more manageable asynchronous code.
Creating a Simple Promise
To create a simple Promise in JavaScript, you can use the following syntax:
In this example, we create a new Promise that simulates an asynchronous operation using setTimeout
. After one second, it either resolves with a success message or rejects with a failure message.
Using Promises
Once you have a Promise, you can handle its result using the .then()
and .catch()
methods. Here's how you can do that:
The .then()
method is called when the Promise is fulfilled, while the .catch()
method is called when it is rejected. This structure helps in managing errors effectively.
Chaining Promises
One of the powerful features of Promises is the ability to chain them. This allows you to perform a series of asynchronous operations in sequence. Here’s an example:
In this example, fetchData
returns a Promise that simulates fetching data, and processData
processes that data. The results are chained together, allowing for a clear flow of asynchronous operations.
Error Handling in Promises
Handling errors in Promises is crucial for building robust applications. You can catch errors at any point in the Promise chain:
In this case, an error is thrown deliberately to demonstrate how to catch it in the chain.
Using async
and await
With the introduction of ES2017, JavaScript introduced async
and await
, which provide a more intuitive way to work with Promises. An async
function always returns a Promise, and await
can be used to pause the execution of the function until the Promise is resolved.Here’s how you can rewrite the previous example using async
and await
:
This syntax simplifies the code and makes it easier to read and maintain.
Conclusion
Understanding and using Promises in JavaScript is essential for modern web development. They provide a powerful way to handle asynchronous operations, making your code cleaner and more manageable. By using async
and await
, you can write asynchronous code that looks synchronous, further enhancing readability.
For more information on JavaScript Promises, you can check out resources like MDN Web Docs or Simply Scripts.By focusing on the principles outlined in this post, you can effectively utilize simple promise js in your projects, leading to better performance and user experience.