Simple Swipe in CSS
Jul 22, 2024
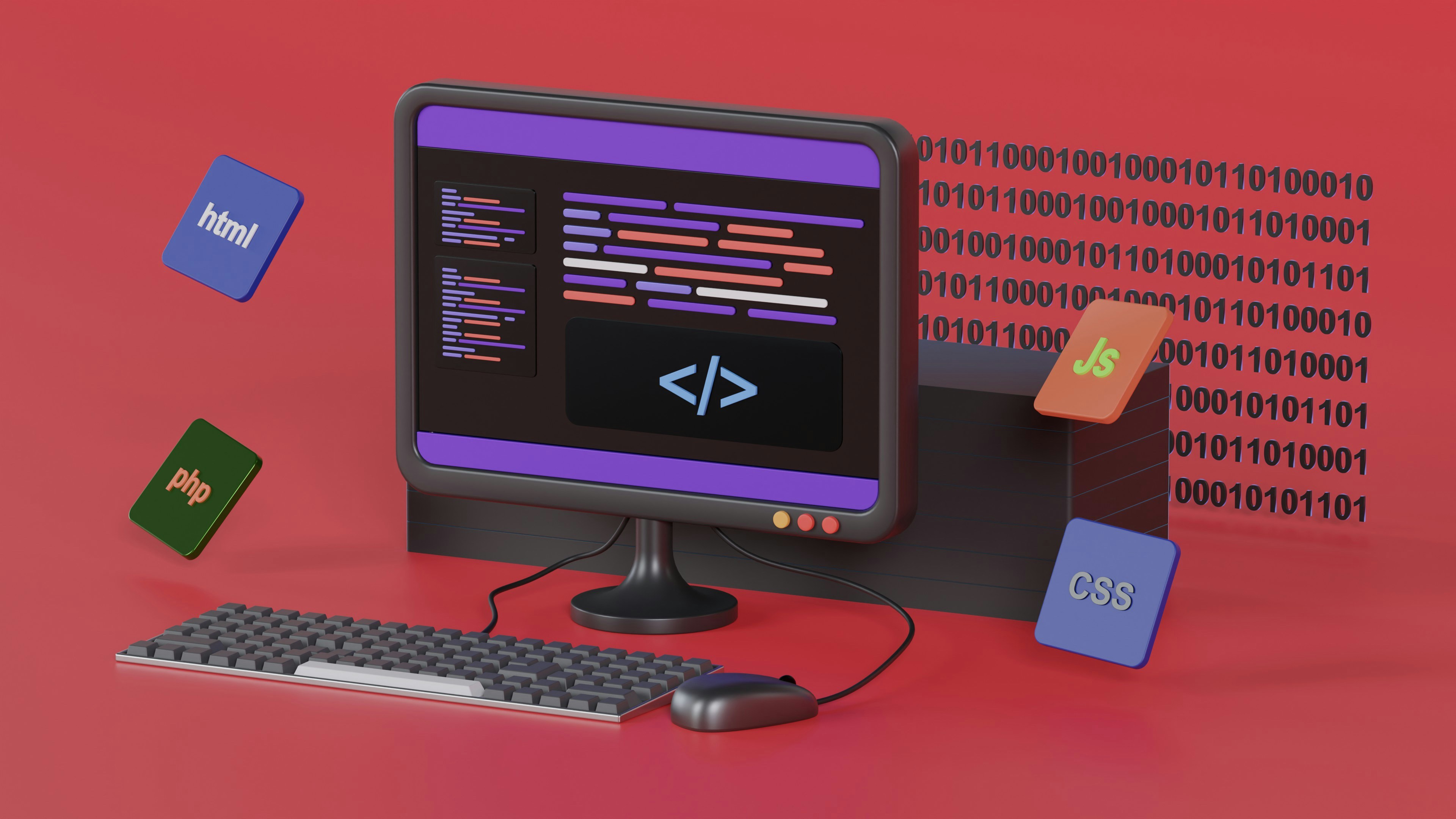
In today's mobile-centric world, creating intuitive and engaging user experiences is crucial for the success of any website or web application. One such feature that has gained popularity in recent years is the simple swipe interaction, which allows users to navigate content or perform actions with a simple swipe gesture. While many developers rely on JavaScript libraries or frameworks to implement swipe functionality, it is possible to achieve a similar effect using pure CSS. In this blog post, we will explore how to create a simple swipe interaction using CSS, along with examples and code snippets to help you get started.
Understanding the Concept
The basic idea behind a simple swipe interaction in CSS is to create a container element that holds the content and a separate element that represents the swipeable content. By using CSS transitions and transforms, we can animate the swipeable content to move horizontally when the user interacts with it.Here's a high-level overview of the steps involved:
Create a container element that holds the swipeable content.
Create a separate element inside the container that represents the swipeable content.
Apply CSS transitions to the swipeable content element to enable smooth animations.
Use CSS transforms to move the swipeable content horizontally when the user interacts with it.
Optionally, add event listeners to the container element to detect user interactions and update the CSS accordingly.
Creating the HTML Structure
Let's start by creating the HTML structure for our simple swipe interaction. We'll have a container element with a class of swipe-container
and a child element with a class of swipe-content
that represents the swipeable content:
<div class="swipe-container"> <div class="swipe-content"> <!-- Content to be swiped goes here --> <h2>Swipeable Content</h2> <p>Swipe left or right to navigate through the content.</p> <!-- Add more content as needed --> </div> </div>
Styling with CSS
Now, let's add some CSS styles to create the simple swipe interaction:
In this CSS code:
The
.swipe-container
class sets the width to 100% and the height to 300 pixels. It also setsoverflow
tohidden
to hide any content that exceeds the container's dimensions.The
.swipe-content
class sets the display toflex
and the width to300%
(three times the container's width). This allows us to have multiple sections of content that can be swiped horizontally. Theheight
is set to100%
to match the container's height. Thetransition
property is added to enable smooth animations when the content is swiped.Inside the
.swipe-content
class, each child element (representing a section of content) is given aflex
value of1
, which means they will share the available space equally. Thepadding
andbox-sizing
properties are used for styling purposes.
Handling User Interactions
To enable the swipe interaction, we need to detect user interactions (such as touch events or mouse events) and update the CSS accordingly. Here's an example of how you can use JavaScript to handle user interactions and update the CSS:
In this JavaScript code:
We select the
.swipe-container
and.swipe-content
elements usingdocument.querySelector
.We define three variables:
isDown
(to track if the mouse button is down),startX
(to store the initial X coordinate of the mouse pointer), andscrollLeft
(to store the initial scroll position of the content).We add event listeners to the
.swipe-container
element formousedown
,mouseleave
,mouseup
, andmousemove
events.When the user presses down the mouse button (
mousedown
), we setisDown
totrue
, store the initial X coordinate and scroll position, and add event listeners formousemove
andmouseup
.During
mousemove
, we calculate the distance the mouse has moved and update thescrollLeft
property of the.swipe-content
element accordingly.When the user releases the mouse button (
mouseup
) or moves the mouse outside the container (mouseleave
), we setisDown
tofalse
to stop the swipe interaction.We also add similar event listeners for touch events (
touchstart
,touchend
, andtouchmove
) to support swipe interactions on mobile devices.
Conclusion
Creating a simple swipe interaction using CSS is a great way to enhance the user experience of your website or web application. By leveraging CSS transitions, transforms, and JavaScript event handling, you can achieve a smooth and responsive swipe interaction without relying on external libraries or frameworks.Remember to test your implementation thoroughly on various devices and browsers to ensure cross-compatibility and a consistent user experience. With a little creativity and attention to detail, you can create engaging and intuitive swipe interactions that keep your users engaged and satisfied.
Happy coding!