Troubleshooting Flutter Asset Not Working Issues
Aug 19, 2024
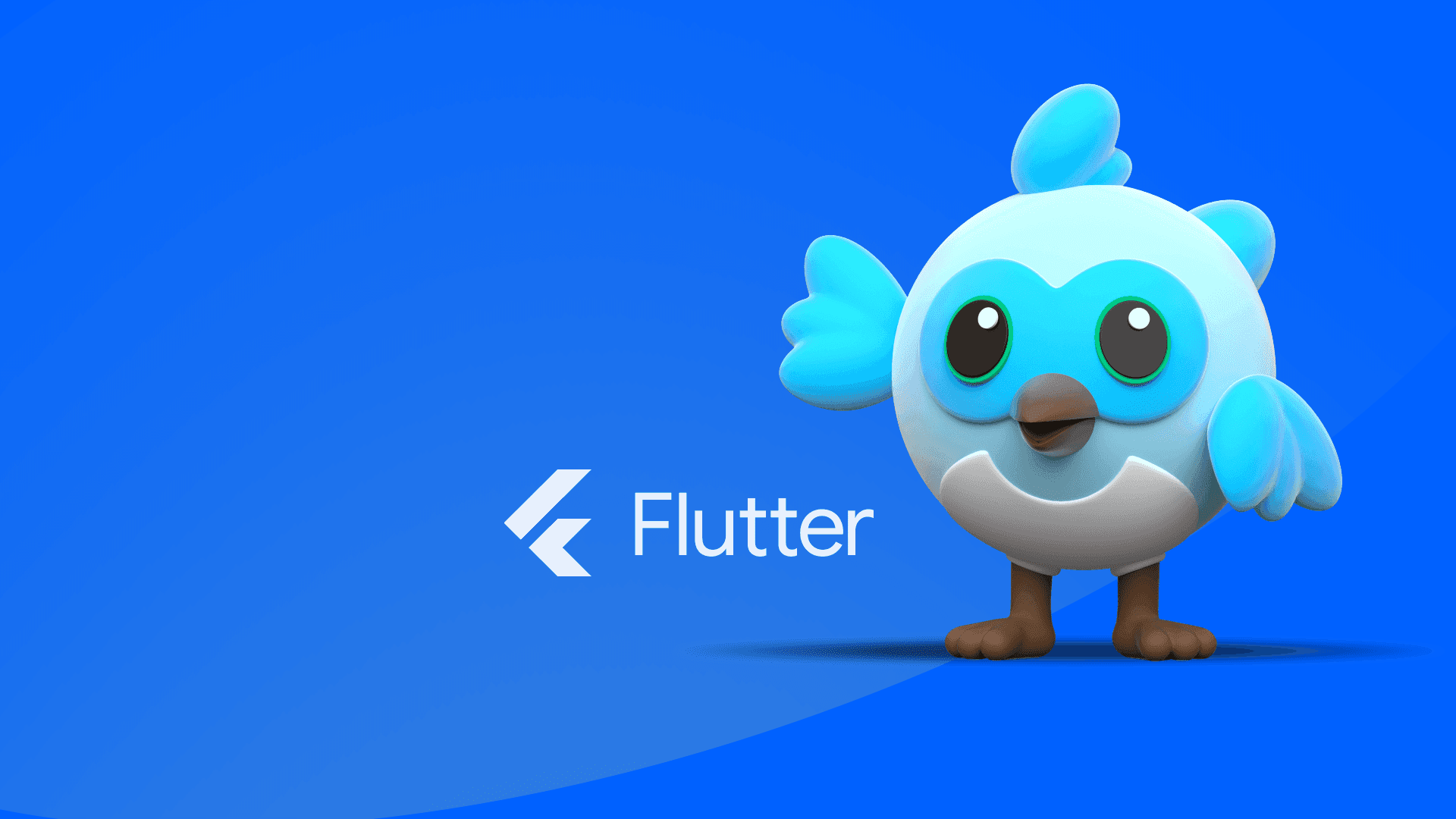
When working with Flutter, managing assets like images, fonts, and other files is crucial for building visually appealing and functional applications. However, sometimes you may encounter issues where your assets are not loading or working as expected. In this comprehensive guide, we'll dive into the common causes of the "Flutter asset not working" problem and provide step-by-step solutions to help you resolve the issue.
Understanding Flutter Assets
In Flutter, assets are any files that are bundled and deployed with your application. These can include images, fonts, JSON files, and other resources that your app needs to function correctly. Flutter supports various types of assets, including:
Images: JPG, PNG, GIF, BMP, WBMP, and WEBP formats
Fonts: TTF and OTF formats
JSON files
Text files
Other binary files
To use an asset in your Flutter app, you need to declare it in the pubspec.yaml
file and reference it in your Dart code.
Declaring Assets in pubspec.yaml
In your pubspec.yaml
file, you need to specify the location of your assets using the assets
key. Here's an example:
In this example, we have three assets declared:
assets/images/logo.png
: An image fileassets/fonts/Roboto-Regular.ttf
: A font fileassets/data/countries.json
: A JSON data file
Make sure that the paths specified in the assets
key match the actual location of your asset files in your project directory.
Referencing Assets in Dart Code
Once you've declared your assets in the pubspec.yaml
file, you can reference them in your Dart code using the AssetImage
or FontLoader
classes. Here's an example of how to use an image asset:
And here's an example of how to use a font asset:
Common Causes of "Flutter Asset Not Working" Issues
Now that you understand the basics of working with assets in Flutter, let's dive into the common causes of the "Flutter asset not working" problem:
Incorrect asset declaration in
pubspec.yaml
Incorrect asset path in Dart code
Missing asset files in the project directory
Asset files not being included in the build
Asset files being ignored by version control systems
Let's explore each of these causes in detail and provide solutions to help you resolve the issue.
Incorrect Asset Declaration in pubspec.yaml
One of the most common causes of the "Flutter asset not working" problem is incorrect asset declaration in thepubspec.yaml
file. Make sure that you've followed the correct syntax and that the paths specified in theassets
key match the actual location of your asset files in your project directory.Here are a few things to check:
Ensure that the indentation is correct: YAML files are sensitive to indentation, so make sure that the
assets
key is properly indented under theflutter
key.Check for typos in file paths: Double-check the spelling and capitalization of your file paths to ensure that they match the actual file names and locations.
Use relative paths: Always use relative paths when specifying asset locations, starting from the
assets
directory in your project.Include the file extension: Make sure to include the file extension (e.g.,
.png
,.ttf
,.json
) in the asset path.
If you've made changes to thepubspec.yaml
file, make sure to runflutter pub get
in your terminal to update the project dependencies.
Incorrect Asset Path in Dart Code
Another common cause of the "Flutter asset not working" problem is incorrect asset path in your Dart code. When referencing an asset in your Dart code usingAssetImage
orFontLoader
, make sure that the path matches the one specified in thepubspec.yaml
file.Here are a few things to check:
Double-check the asset path: Ensure that the path specified in your Dart code matches the one declared in the
pubspec.yaml
file, including the file extension.Use relative paths: Just like in the
pubspec.yaml
file, use relative paths starting from theassets
directory in your project.Avoid hardcoding paths: Consider using constants or variables for asset paths to make them easier to manage and update.
Here's an example of how to correctly reference an image asset in your Dart code:
Missing Asset Files in the Project Directory
If your asset files are not present in the project directory, Flutter won't be able to locate and load them. Make sure that you've placed your asset files in the correct location in your project directory, and that the paths specified in thepubspec.yaml
file match the actual file locations.Here are a few things to check:
Ensure that the asset files exist: Double-check that the asset files you've specified in the
pubspec.yaml
file are present in your project directory.Check the file locations: Verify that the asset files are placed in the correct directories relative to the
assets
directory.Use the correct file names: Make sure that the file names specified in the
pubspec.yaml
file match the actual file names, including the file extension.
If you've added new asset files to your project, make sure to runflutter pub get
in your terminal to update the project dependencies.
Asset Files Not Being Included in the Build
Even if your asset files are present in the project directory and correctly declared in thepubspec.yaml
file, they may not be included in the build process. This can happen if you're using a build configuration that excludes certain files or directories.Here are a few things to check:
Verify the build configuration: Ensure that your build configuration (e.g.,
flutter build apk
,flutter build ios
) includes theassets
directory and the specified asset files.Check for build exclusions: If you're using a build configuration that excludes certain files or directories, make sure that the
assets
directory and the specified asset files are not being excluded.Clean and rebuild the project: Try cleaning and rebuilding your project by running
flutter clean
followed byflutter build apk
(for Android) orflutter build ios
(for iOS) in your terminal.
Asset Files Being Ignored by Version Control Systems
If you're using a version control system like Git, your asset files may be ignored by default. This can prevent the asset files from being included in the build process and cause the "Flutter asset not working" problem.Here are a few things to check:
Verify the
.gitignore
file: If you're using Git, check the.gitignore
file in your project directory to ensure that theassets
directory and the specified asset files are not being ignored.Remove asset files from the ignore list: If the
assets
directory or the specified asset files are being ignored, remove them from the.gitignore
file.Add asset files to the version control system: If the asset files are not being tracked by the version control system, add them using the appropriate commands (e.g.,
git add assets/images/logo.png
).
By following these steps and troubleshooting the common causes of the "Flutter asset not working" problem, you should be able to resolve the issue and ensure that your assets are loading correctly in your Flutter app.
Conclusion
In this comprehensive guide, we've covered the common causes of the "Flutter asset not working" problem and provided step-by-step solutions to help you resolve the issue. By understanding how Flutter assets work, correctly declaring them in thepubspec.yaml
file, and referencing them in your Dart code, you can ensure that your assets are loading correctly in your Flutter app.