Understanding Headless Code: A Comprehensive Guide
Aug 6, 2024
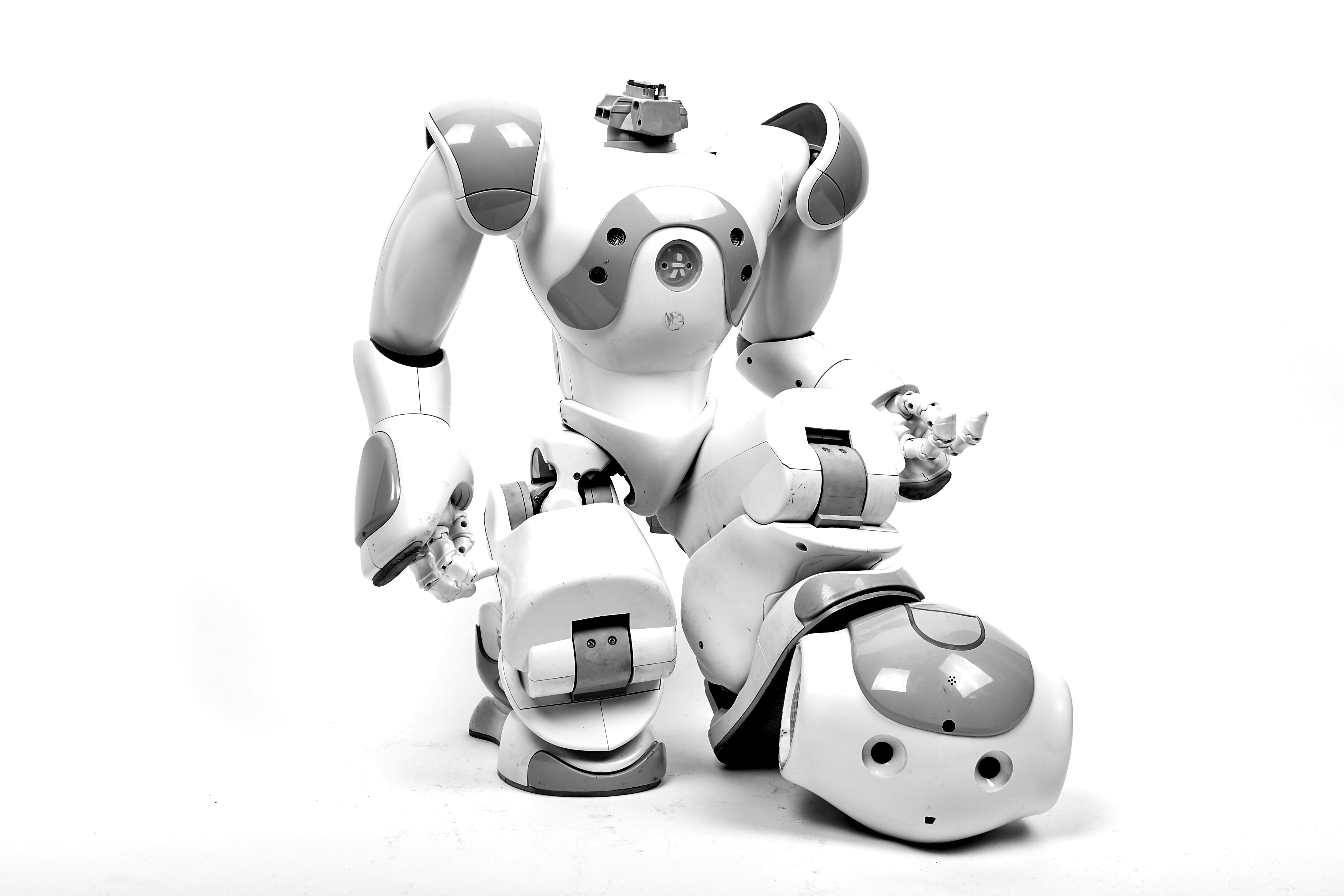
In the evolving landscape of web development and software architecture, the term headless code has gained significant traction. This concept refers to a design approach where the backend of an application operates independently from the frontend. In this blog post, we will explore the intricacies of headless code, its benefits, implementation strategies, and practical examples, complete with coding snippets to enhance your understanding.
What is Headless Code?
Headless code refers to applications or systems where the frontend (the user interface) is decoupled from the backend (the server-side logic and database). This architecture allows developers to build flexible, scalable applications that can serve content across multiple platforms without being tied to a specific presentation layer.
Key Components of Headless Architecture
Backend: The server-side logic, APIs, and databases that handle data storage and processing.
Frontend: The presentation layer that users interact with, which can be built using various frameworks or technologies.
API: The communication layer that allows the frontend to interact with the backend, typically using RESTful or GraphQL APIs.
The Benefits of Using Headless Code
Adopting headless code architecture offers numerous advantages, including:
Flexibility: Developers can choose the best technologies for both frontend and backend, allowing for tailored solutions that meet specific project needs.
Scalability: Headless systems can be scaled independently, making it easier to manage traffic and performance.
Faster Development: With the separation of concerns, teams can work on the frontend and backend simultaneously, speeding up the development process.
Omnichannel Capabilities: Content can be delivered across various platforms (web, mobile, IoT) seamlessly, enhancing user experience.
Improved Performance: Headless applications often load faster, as they can be optimized independently from the backend.
Implementing Headless Code: A Step-by-Step Guide
To illustrate how to implement headless code, let’s consider a simple example of a headless content management system (CMS) using Node.js for the backend and React for the frontend.
Step 1: Setting Up the Backend
First, we need to create a basic Node.js server that serves
content through an API
.
This code sets up a simple Express server with a single API endpoint that returns a list of posts.
Step 2: Creating the Frontend
Next, we will create a React application that fetches and displays the posts from our backend.
In this React component, we use the useEffect
hook to fetch data from our backend API when the component mounts. The fetched posts are stored in the state and displayed in a list.
Real-World Applications of Headless Code
Headless code is not just a theoretical concept; it has practical applications across various industries. Here are some notable examples:
E-commerce: Many online retailers use headless commerce platforms to manage their product catalogs and customer data while offering a customized shopping experience across multiple channels.
Content Management:
Headless CMS platforms
like Contentful and Strapi allow organizations to manage content centrally and distribute it to various frontends, such as websites, mobile apps, and digital signage.IoT Applications: Headless architecture is ideal for Internet of Things (IoT) applications, where devices need to communicate with a backend without a traditional user interface.
Challenges and Considerations
While headless code offers many benefits, there are also challenges to consider:
Complexity: Managing multiple systems (frontend and backend) can introduce complexity, requiring robust API management and monitoring.
Development Skills: Teams may need to possess a diverse skill set to work effectively with different technologies for the frontend and backend.
SEO Considerations: Ensuring that headless applications are optimized for search engines can be more challenging, as traditional SEO practices may not apply directly.
Conclusion
In conclusion, headless code represents a modern approach to software development that decouples the frontend from the backend, offering flexibility, scalability, and improved performance. By leveraging APIs, developers can create tailored solutions that meet the demands of today’s digital landscape.As more businesses adopt headless architectures, understanding and implementing headless code will become increasingly important for developers and organizations alike. Whether you are building a simple blog or a complex e-commerce platform, embracing headless principles can lead to a more agile and responsive development process.