Understanding Long Running Activities in TypeScript
Aug 19, 2024
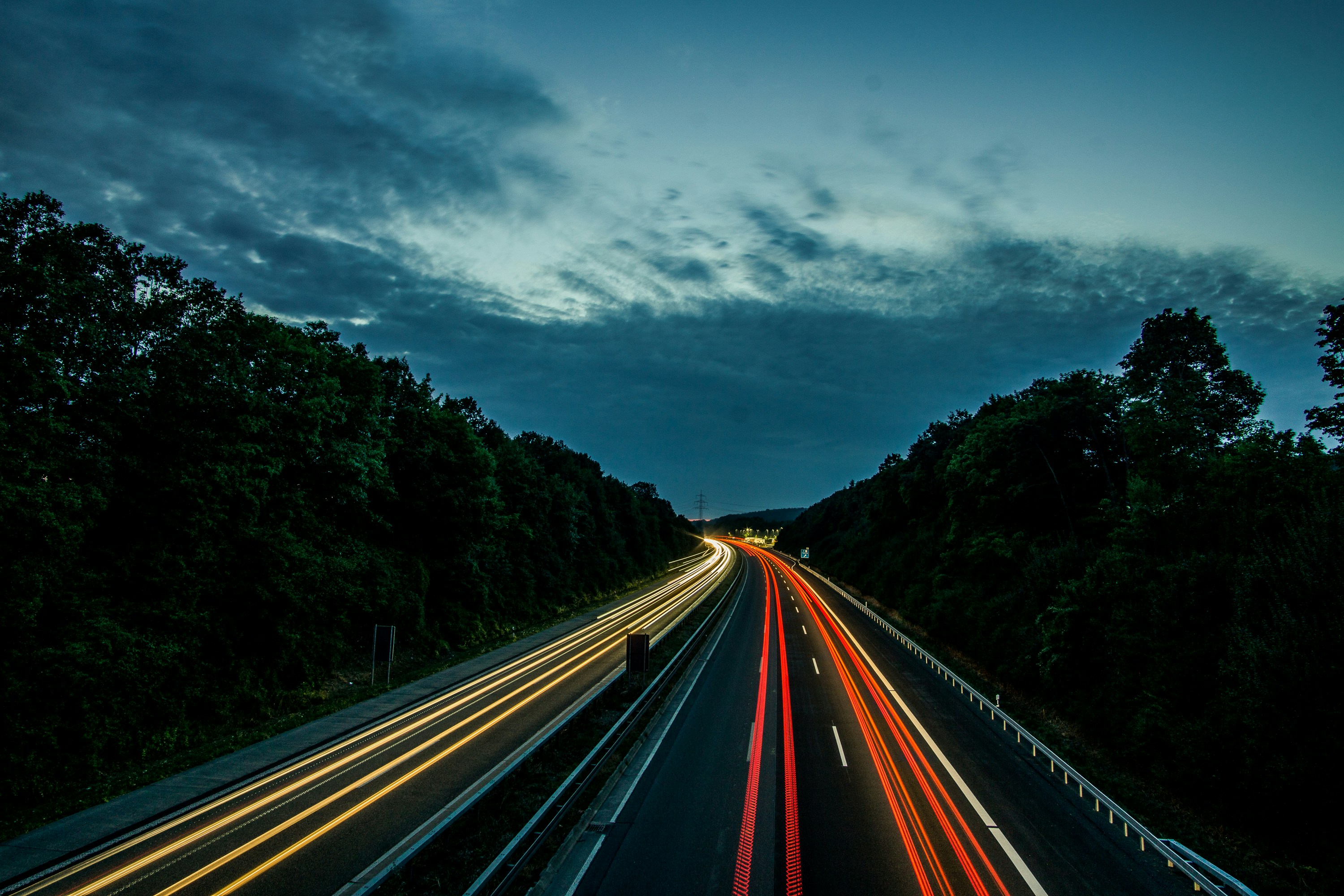
In modern web applications, managing long-running activities is crucial for maintaining a responsive user experience. This blog post will explore the concept of long-running activities in TypeScript, providing examples and best practices to help developers implement them effectively. We will cover various scenarios where long-running tasks are necessary, the challenges they present, and how to handle them using TypeScript.
What Are Long Running Activities?
Long-running activities refer to tasks that take a significant amount of time to complete, such as data processing, file uploads, or network requests. These activities can lead to a poor user experience if not handled properly, as they can block the main thread, causing the application to become unresponsive.
Why Use TypeScript for Long Running Activities?
TypeScript is a superset of JavaScript that adds static typing and other features, making it an excellent choice for managing complex applications. Here are some reasons to use TypeScript for long-running activities:
Type Safety: TypeScript helps catch errors at compile time, reducing runtime errors and improving code quality.
Enhanced Readability: With TypeScript's type annotations, code becomes more self-documenting, making it easier for developers to understand the flow of long-running tasks.
Better Tooling: TypeScript's integration with IDEs provides features like autocompletion and refactoring tools, which can speed up development.
Common Scenarios for Long Running Activities
Data Fetching: Making API calls to retrieve large datasets.
File Uploads: Uploading large files to a server.
Image Processing: Manipulating images on the client side before sending them to a server.
Complex Calculations: Performing intensive calculations that may take time to complete.
Implementing Long Running Activities in TypeScript
To effectively manage long-running activities in TypeScript, we can use asynchronous programming patterns such as Promises and async/await. Below, we provide a basic example of how to implement a long-running activity using TypeScript.
Example: Fetching Data from an API
Handling Long Running Activities with Web Workers
For tasks that are computationally intensive, using Web Workers is a great way to offload processing to a separate thread. This prevents blocking the main thread and keeps the UI responsive.
Example: Using Web Workers in TypeScript
Create a Web Worker File (worker.ts):
Main Application File (app.ts):
Best Practices for Managing Long Running Activities
Use
Asynchronous Patterns
: Always prefer asynchronous programming to keep the UI responsive.Implement Loading Indicators: Show users that a task is in progress to improve user experience.
Error Handling
: Implement robust error handling to manage failures gracefully.Optimize Performance: Break tasks into smaller chunks if possible, and use techniques like throttling or debouncing.
Utilize Web Workers
: For CPU-intensive tasks, consider using Web Workers to run processes in the background.
Conclusion
Managing long-running activities in TypeScript is essential for creating responsive web applications. By leveraging asynchronous programming and Web Workers, developers can ensure that their applications remain performant and user-friendly. This blog post provided a comprehensive overview of long-running activities, practical examples, and best practices to help you implement them effectively in your TypeScript projects.