Understanding the else if Statement in JavaScript
Aug 20, 2024
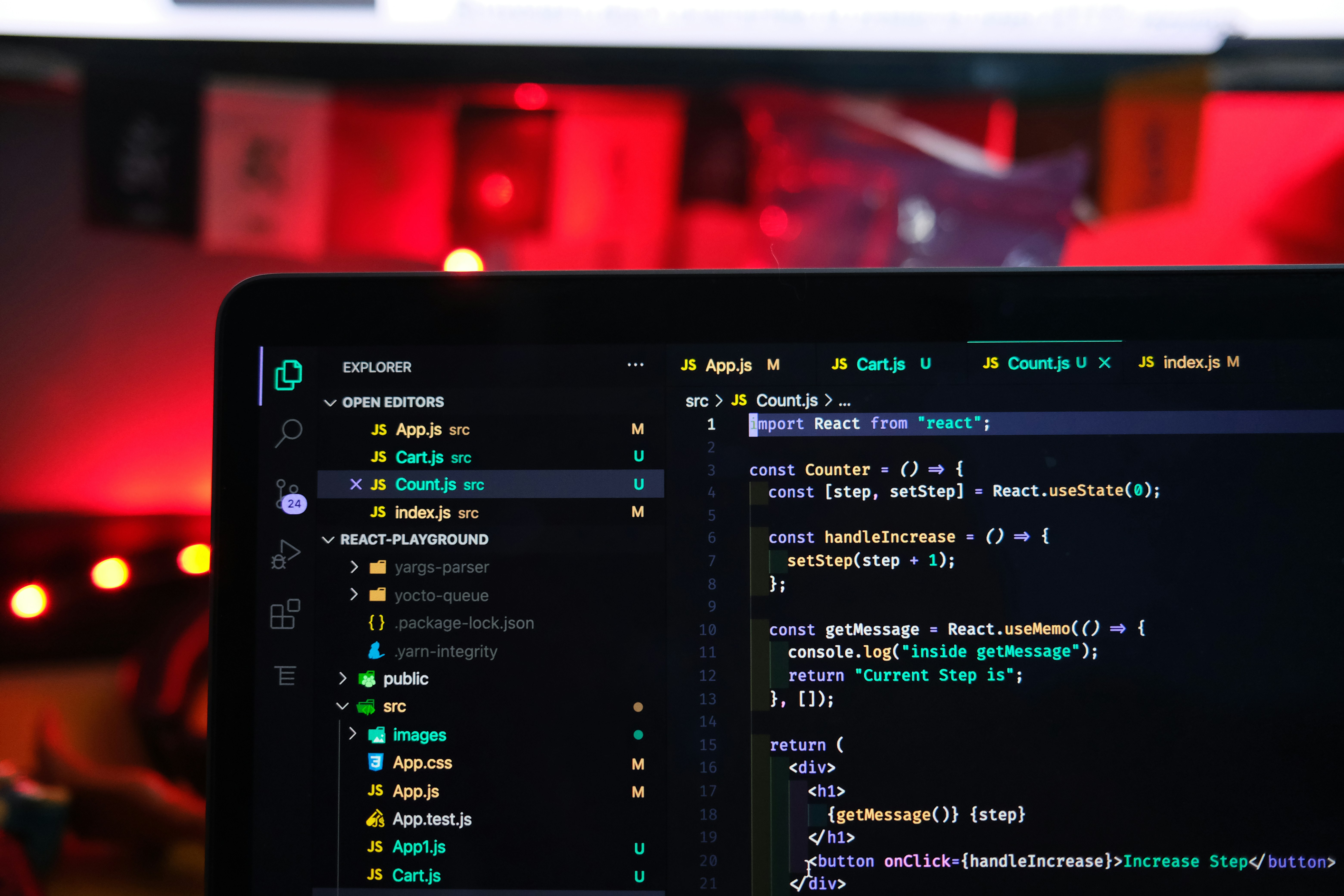
The else if
statement in JavaScript is a fundamental control structure that allows developers to execute different blocks of code based on multiple conditions. This feature is crucial for creating dynamic and responsive applications, enabling more complex decision-making processes than a simple if
or else
statement could provide.
What is else if
in JavaScript?
The else if
statement is used to specify a new condition to test if the previous if
condition was false. It allows for the evaluation of multiple expressions, providing a way to branch logic based on various criteria. Here’s the general syntax:
Example of Using else if
To illustrate howelse if
works, consider the following example that assesses a student's grade based on their score:
In this example, the program checks the score
variable against multiple conditions. If the score is 85, it will output "Grade: B" since it meets the second condition.
When to Use else if
You should use else if
when you have multiple conditions to evaluate that are mutually exclusive. This means that only one block of code will execute based on the first true condition. If you have several distinct paths that your code could take, else if
is the right choice.
Benefits of Using else if
Clarity: Using
else if
makes your code clearer and easier to read compared to multiple nestedif
statements.Efficiency: It stops evaluating conditions once a true condition is found, which can improve performance in complex decision trees.
Flexibility: You can handle multiple scenarios without duplicating code, making it easier to maintain.
Common Mistakes with else if
Omitting the
else
: If you forget to include anelse
at the end, you may not handle cases where none of the conditions are true, potentially leading to unexpected behavior.Overlapping Conditions: Ensure that your conditions do not overlap. For example, if you have
if (x > 10)
andelse if (x > 5)
, the second condition will never be reached if the first is true.Complex Conditions: While
else if
is powerful, overly complex conditions can make your code hard to read. Consider breaking them into smaller functions or using a switch statement for clarity.
Using else if
with Logical Operators
You can also combine else if
with logical operators like &&
(AND) and ||
(OR) to create more complex conditions. Here’s an example:
In this case, the second condition checks if the temperature is between 20 and 30 degrees, providing a more nuanced output based on the range of values.
Conclusion
The else if
statement is a vital part of JavaScript programming, allowing for more sophisticated decision-making in your code. By understanding how to use it effectively, you can create programs that respond intelligently to different conditions, enhancing user experience and application functionality.
For more in-depth information about JavaScript control structures, check out resources like MDN Web Docs and W3Schools.