Understanding the Need to Hide Slider Arrows
Aug 9, 2024
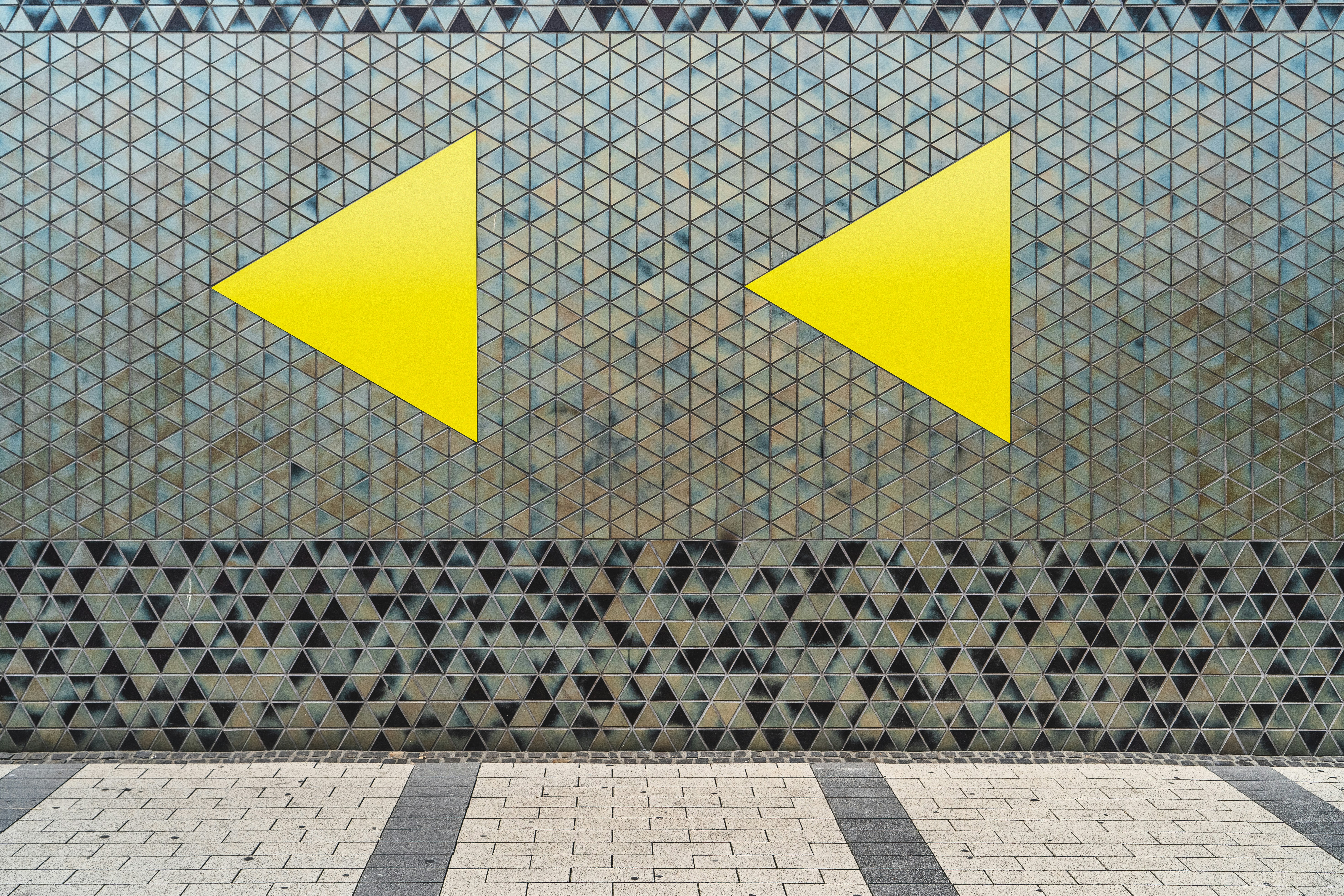
CSS Techniques to Hide Slider Arrows
Basic CSS for Hiding Scrollbars
Before diving into hiding slider arrows, it’s essential to understand how to manage scrollbars effectively. You can hide scrollbars without losing scrolling functionality
using the following CSS:
This CSS snippet will hide the scrollbar while allowing for scrolling within the designated element, thus maintaining usability while achieving a clean design.
Hiding Slider Arrows with CSS
To hide slider arrows when reaching the end of a scroll, you can utilize CSS along with JavaScript to dynamically add or remove classes based on the scroll position. Here’s a simple example:
In this CSS, we define a class that hides the arrows. We will use JavaScript to toggle this class based on the scroll position.
JavaScript for Dynamic Arrow Hiding
To achieve the functionality where arrows are hidden at the end of the scroll, we can use the following JavaScript code:
This script listens for the scroll event on the slider. It checks the current scroll position and hides the appropriate arrow based on whether the user is at the beginning or the end of the scrollable area.
How to Remove Scroll from Text Area
In some cases, you may want to remove the scroll from a text area while still allowing text input. You can achieve this by setting the overflow property to hidden:
However, this will also prevent any scrolling within the text area, which may not be desirable. If you want to keep scrolling functionality while hiding the scrollbar, you can use:
This allows users to scroll through the text area without displaying the scrollbar, maintaining a clean interface.
CSS Scrolling Bar Customization
Customizing the appearance of scrollbars can also enhance the aesthetics of your website. Here’s how you can style scrollbars using CSS:
This CSS allows you to create a visually appealing scrollbar that matches your website’s design.
Conclusion
Hiding slider arrows at the end of a scroll enhances user experience by decluttering the interface. By employing CSS and JavaScript, you can effectively manage the visibility of slider arrows and scrollbars.
Utilizing the techniques outlined in this post, you can create a seamless and visually appealing web experience. Remember to consider accessibility when hiding scrollbars and arrows, ensuring that users can still navigate your content effectively.