Understanding the Problem: Why You Can't See Animated Scroll Bars
Aug 6, 2024
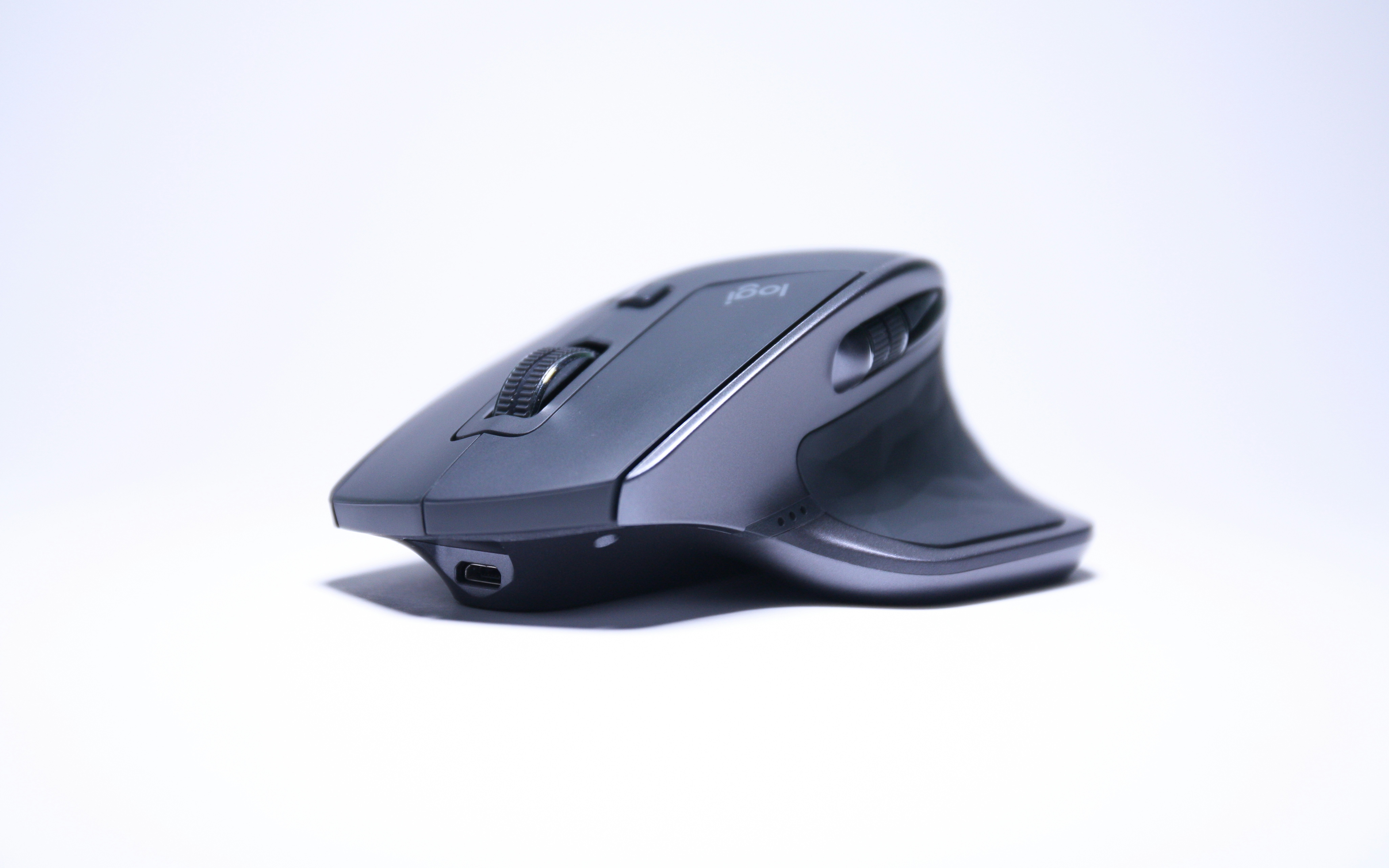
When developing web applications or websites, you might encounter a frustrating issue: the scrolling bar can't see animate. This phenomenon can disrupt user experience, especially when animations are involved. In this blog post, we will explore the reasons behind this issue and provide solutions to ensure your scrolling animations are smooth and visually appealing.
The Basics of Scroll Bars
Before diving into the specifics of animation, it's essential to understand what scroll bars are and how they function within web design. Scroll bars allow users to navigate through content that exceeds the visible area of a webpage or application. They can appear in various forms, including vertical and horizontal bars, and are crucial for user interaction.
Why Scroll Bars Might Not Animate
There are several reasons why a scrolling bar might not animate
as expected:
CSS Properties: Certain CSS properties cannot be animated. For instance, properties like
overflow
ordisplay
do not support transitions, which can lead to issues when trying to animate scroll bars.JavaScript Limitations: If you're using
JavaScript to control animations
, you might encounter limitations based on how the DOM is manipulated. For example, if the scroll bar is hidden or removed from the DOM during an animation, it won't be visible.Browser Compatibility: Different browsers may handle animations and scroll bars differently. It's crucial to test across multiple browsers to ensure consistency.
Solutions to Make Scroll Bars Animate Smoothly
To address the issue of the scrolling bar can't see animate, we can implement several techniques using CSS and JavaScript.
1. Using CSS Transitions
CSS transitions can help create smooth animations for scroll bars. Here’s a simple example:
In this example, the .scroll-content
will move up when the user hovers over the .scroll-container
, creating a smooth scrolling effect.
2. Hiding Scroll Bars During Animation
One common technique is to hide the scroll bars during an animation. This can be achieved using CSS and JavaScript. Here’s how you can do it:
In this code snippet, the scroll content is hidden initially. When the .scroll-container
is given the class open
, the content expands, and the scroll bars become visible only after the animation completes.
3. JavaScript for Scroll Control
Using JavaScript, you can control the visibility of scroll bars dynamically. Here’s an example:
This script toggles the open
class on the scroll container when a button is clicked, triggering the CSS animations defined earlier.
Advanced Techniques for Scroll Bar Animations
For more advanced animations, consider using libraries like GSAP (GreenSock Animation Platform) or Anime.js. These libraries provide robust tools for creating complex animations, including scroll bar effects.
Example with GSAP
Best Practices for Scroll Bar Animations
When implementing scroll bar animations, keep the following best practices in mind:
Performance: Ensure that animations do not hinder performance. Use hardware-accelerated CSS properties where possible.
Accessibility: Make sure that animations do not interfere with users who may have motion sensitivity. Provide options to disable animations if necessary.
Testing: Always test your animations across different devices and browsers to ensure compatibility and smooth performance.
Conclusion
The issue of thescrolling bar can't see animatecan be addressed through various techniques involving CSS and JavaScript. By understanding the underlying causes and implementing the suggested solutions, you can create a seamless and visually appealing user experience.Remember, the key to successful animations lies in balancing aesthetics with performance and accessibility. By following best practices and leveraging modern animation libraries, you can enhance your web applications and provide users with an engaging experience.