Understanding Vectors in Machine Learning
Aug 16, 2024
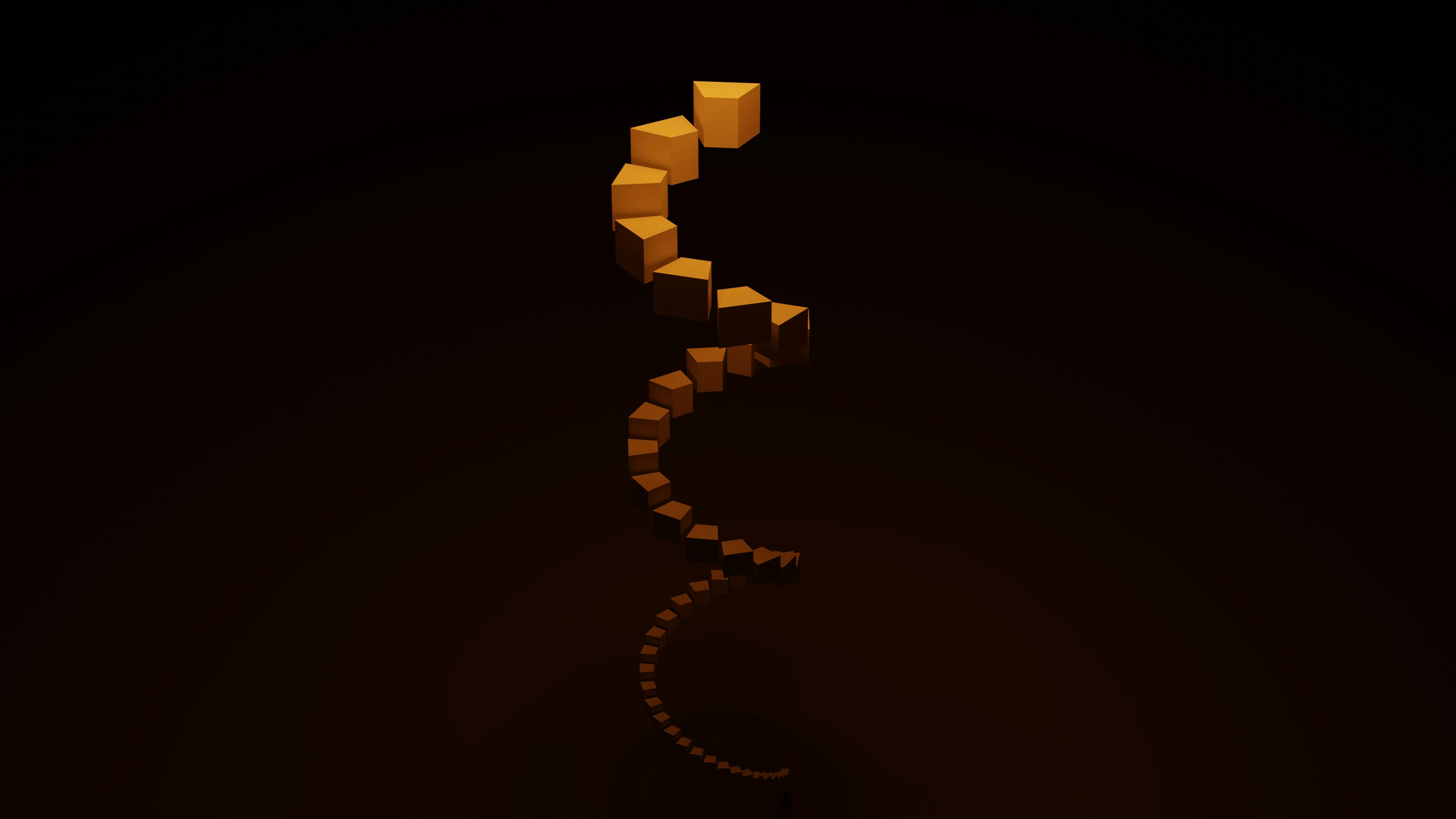
Vectors play a crucial role in machine learning, serving as fundamental components for data representation, model training, and algorithm implementation. This blog post will explore the concept of vectors in machine learning, their applications, and the mathematical principles behind them. By the end, you will have a comprehensive understanding of how vectors function in the realm of machine learning, along with practical coding examples.
What is a Vector?
A vector is a mathematical object that has both magnitude and direction. In the context of machine learning, vectors are used to represent data points in a multi-dimensional space. Each vector can be thought of as a tuple of numerical values, which can represent features of the data or parameters of a model.
Key Characteristics of Vectors
Magnitude: This refers to the length of the vector, which can be calculated using the Euclidean distance formula.
Direction: This indicates the orientation of the vector in space, which is essential for understanding relationships between different data points.
In machine learning, vectors are often used to represent feature sets of data points, where each feature corresponds to a dimension in the vector space.
The Role of Vectors in Machine Learning
Vectors are integral to various machine learning algorithms and processes. Here are some key applications:
Data Representation: Vectors allow for the representation of complex data in a structured format. For example, an image can be represented as a vector of pixel values.
Feature Vectors: A feature vector is an array of numerical values that represent the features of a single data point. For instance, a feature vector for a house might include its size, number of bedrooms, and location.
Target Variables: In supervised learning, the target variable (often denoted as yy) can also be represented as a vector, especially in multi-class classification problems.
Support Vector Machines (SVM): This is a popular algorithm that uses vectors to classify data points by finding the optimal hyperplane that separates different classes.
Mathematical Operations on Vectors
Understanding how to perform operations on vectors is essential for implementing machine learning algorithms. Here are some common vector operations:
1. Vector Addition
Vector addition involves combining two vectors to form a new vector. If we have two vectors aa and bb:
c=a+b
In Python, using NumPy, vector addition can be performed as follows:
2. Vector Subtraction
Vector subtraction is similar to addition but involves taking the difference between two vectors:
c=a−b
Example in Python:
3. Dot Product
The dot product of two vectors is a scalar value that represents the cosine of the angle between them, multiplied by their magnitudes:
a⋅b=∑i=1naibi
4. Cross Product
The cross product is only defined for three-dimensional vectors and results in a new vector that is perpendicular to the plane formed by the two input vectors:
c=a×b
Example in Python:
Vectors in Machine Learning Algorithms
Support Vector Machines (SVM)
Support Vector Machines are a powerful class of supervised learning algorithms used for classification and regression tasks. The main idea behind SVM is to find the hyperplane that best separates the classes in the feature space.How SVM Works:
Hyperplane: In a two-dimensional space, a hyperplane is a line that separates the data points of different classes. In higher dimensions, it becomes a plane or a hyperplane.
Support Vectors: These are the data points that are closest to the hyperplane. They are critical in defining the position of the hyperplane.
Maximizing the Margin: The goal of SVM is to maximize the margin between the hyperplane and the support vectors, ensuring the best separation of classes.
Here’s a simple implementation of SVM using thescikit-learn
library in Python:
Vectorization in Machine Learning
Vectorization is the process of converting data into a vector format suitable for machine learning algorithms. This is crucial because most machine learning models require numerical input.
Example: One-Hot Encoding
One common method of vectorization isone-hot encoding, which transforms categorical variables into a binary vector representation. For instance, if we have a categorical feature "Color" with three possible values (Red, Green, Blue), one-hot encoding would represent them as follows:
Red: [1, 0, 0]
Green: [0, 1, 0]
Blue: [0, 0, 1]
In Python, this can be done usingpandas
:
Challenges with Vectors in Machine Learning
While vectors are powerful, they also come with challenges:
High Dimensionality: As the number of features increases, the dimensionality of the vector space grows, which can lead to the curse of dimensionality, making algorithms less effective.
Computational Complexity: Operations on large vectors can be computationally intensive, requiring optimized algorithms and hardware.
Data Sparsity: In many applications, especially with high-dimensional data, vectors can be sparse, meaning that most of their elements are zero. This can complicate the learning process.
Conclusion
Vectors are fundamental to the field of machine learning, providing a means to represent data and enable various algorithms to function effectively. Understanding how to manipulate and utilize vectors is essential for anyone looking to delve into machine learning. By mastering vector operations and their applications, you can enhance your ability to develop robust machine learning models.