Understanding Vital Flex Core
Aug 19, 2024
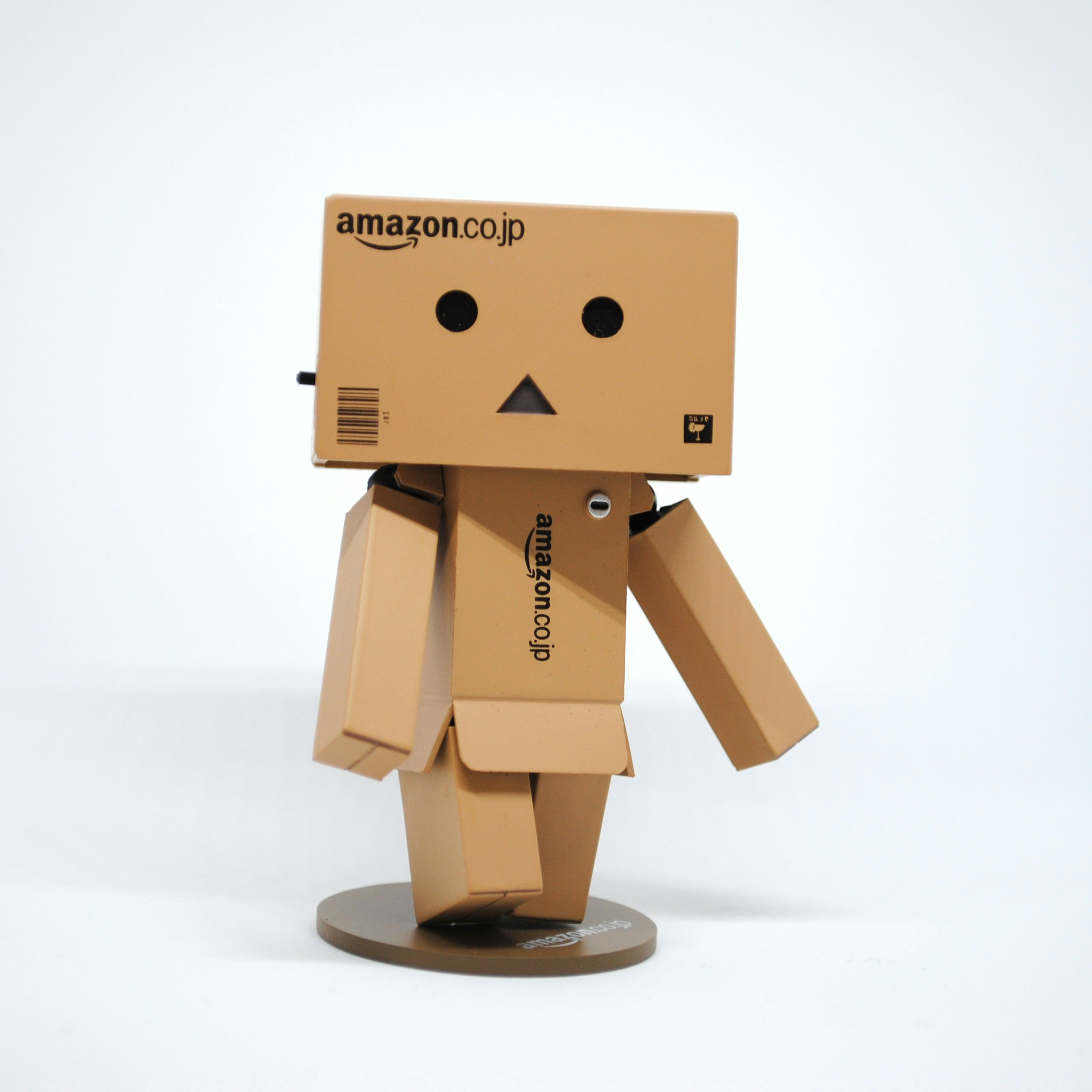
What is Vital Flex Core?
Vital Flex Core is a versatile framework designed to facilitate the development of applications across various platforms. It provides a comprehensive set of tools and libraries that streamline the coding process, allowing developers to focus on building features rather than dealing with underlying complexities.
Key Features of Vital Flex Core
Modular Architecture: Vital Flex Core is built on a modular architecture, enabling developers to utilize only the components they need. This reduces bloat and enhances application performance.
Cross-Platform Compatibility: One of the standout features of Vital Flex Core is its ability to support multiple platforms, including web, mobile, and desktop applications. This flexibility allows developers to write code once and deploy it across various environments.
Robust Security Protocols: Security is paramount in today’s digital landscape. Vital Flex Core incorporates advanced security features, including encryption and authentication mechanisms, to safeguard applications against vulnerabilities.
Rich Ecosystem: The framework comes with a rich ecosystem of plugins and extensions that enhance its functionality. Developers can easily integrate third-party tools and libraries to extend the capabilities of their applications.
Comprehensive Documentation: Vital Flex Core is supported by extensive documentation, making it easier for developers to understand its features and functionalities. This resource is invaluable for both beginners and experienced developers.
Getting Started with Vital Flex Core
To begin using Vital Flex Core, developers need to install the framework and set up their development environment. Below are the steps to get started:
Installation
Prerequisites:
Ensure you have Node.js installed on your machine.
Install a code editor (e.g., Visual Studio Code, Atom).
Install Vital Flex Core:
Open your terminal and run the following command:
Create a New Project:
After installation, create a new project by running:
Navigate to Your Project Directory:
Change into your project directory:
Start the Development Server:
Launch the development server to see your application in action:
Building Your First Application
Now that you have set up your environment, let’s build a simple application using Vital Flex Core. This example will demonstrate how to create a basic to-do list application.
Code Snippet: Creating a To-Do List
In your project directory, locate thesrc
folder and create a new file namedTodoApp.js
. Add the following code:
Explanation of the Code
Imports: The code imports React and Vital from the Vital Flex Core framework. React is used for building the user interface, while Vital provides the core functionalities.
State Management: The
useState
hook is used to manage the state of the to-do list and the input field.Adding Tasks: The
addTodo
function adds a new task to the list if the input is not empty.Rendering the Component: The component renders an input field, a button to add tasks, and a list of current tasks.
Enhancing Your Application
To further enhance the to-do list application, consider adding features such as:
Task Deletion: Allow users to remove tasks from the list.
Task Completion: Implement a feature to mark tasks as completed.
Persistent Storage: Use local storage or a database to save tasks between sessions.
Code Snippet: Adding Task Deletion
To add the ability to delete tasks, modify theTodoApp.js
file as follows:
Conclusion
Vital Flex Core is a powerful framework that simplifies the development of cross-platform applications. By leveraging its modular architecture and rich ecosystem, developers can create robust applications efficiently. This blog post provided an overview of Vital Flex Core, its features, and a practical coding example to help you get started.
As you continue to explore Vital Flex Core, consider experimenting with its various features and capabilities. The more you practice, the more proficient you will become in utilizing this framework to build exceptional applications.