Using Maps in Programming: A Comprehensive Guide
Aug 22, 2024
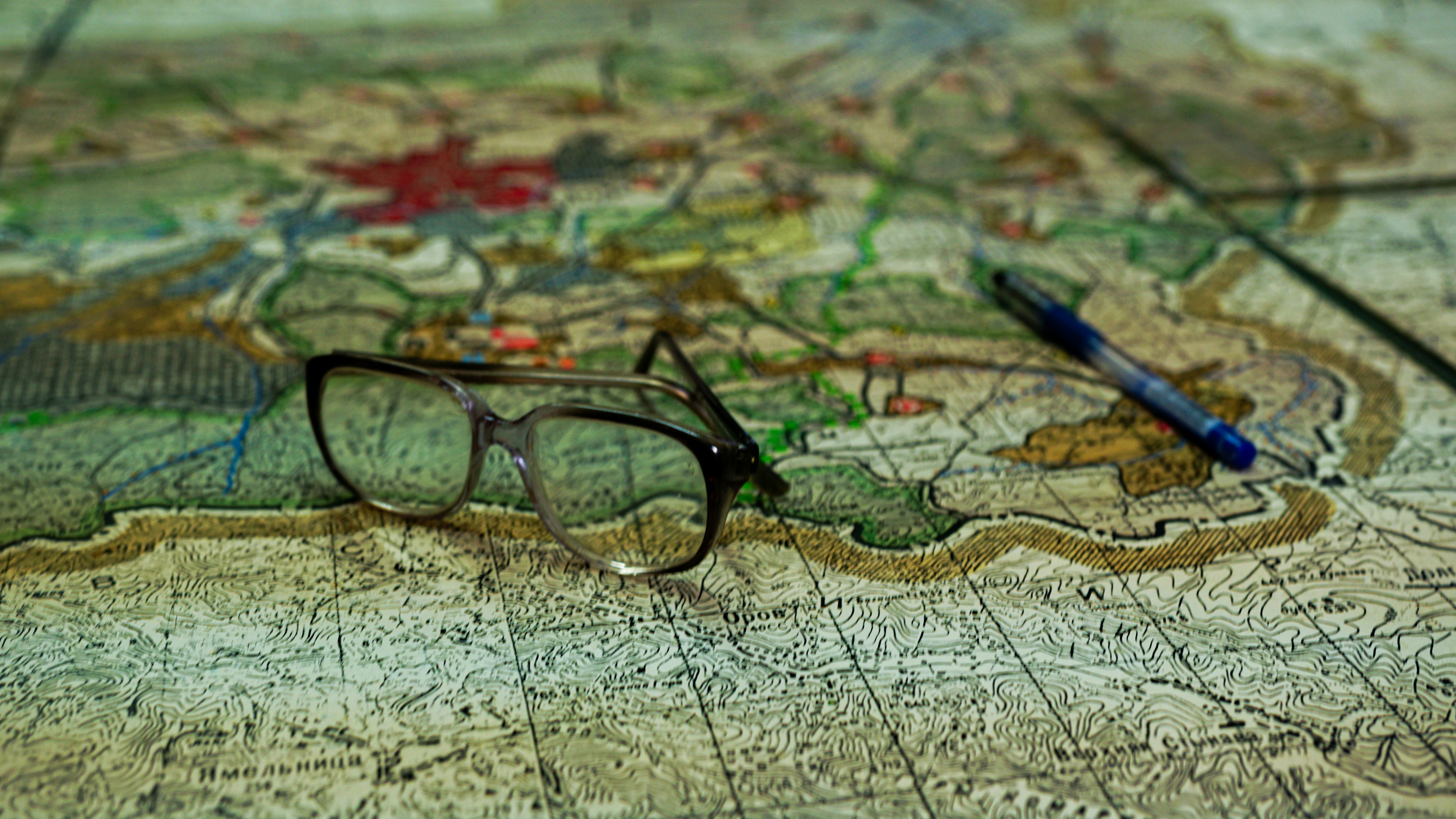
Maps are a fundamental data structure in programming that allows developers to store key-value pairs. They are widely used across various programming languages due to their efficiency and versatility. In this blog post, we will explore the concept of maps, their implementation in different programming languages, and practical use cases. We will also include code snippets to illustrate how to use maps effectively.
What is a Map?
A map is a collection of key-value pairs where each key is unique, and it is used to retrieve values based on their corresponding keys. Maps are also known as dictionaries in Python, hash tables in Java, and associative arrays in JavaScript.
Key Characteristics of Maps:
Unique Keys: Each key in a map must be unique. If a key is repeated, the new value will overwrite the existing one.
Dynamic Size: Maps can grow and shrink dynamically, allowing for flexible data management.
Fast Lookups: Maps provide efficient data retrieval, typically with average time complexity of O(1) for lookups.
Why Use Maps?
Maps are particularly useful when you need to associate values with unique keys. Here are some common scenarios where maps excel:
Counting Frequencies: Counting occurrences of items (e.g., words in a text).
Caching Data: Storing results of expensive function calls to avoid repeated computations.
Configuration Settings: Storing application settings where each setting can be accessed via a key.
Implementing Maps in Different Programming Languages
1. Python
In Python, maps are implemented using dictionaries. Here’s how to create and use a dictionary:
2. Java
In Java, theHashMap
class is commonly used to implement maps. Below is an example:
3. JavaScript
In JavaScript, maps can be created using theMap
object. Here’s an example:
Common Operations on Maps
Adding Key-Value Pairs
Adding a key-value pair is straightforward across languages. You typically use a method likeput
in Java,set
in JavaScript, and direct assignment in Python.
Removing Key-Value Pairs
You can remove entries from maps using methods likeremove
in Java,delete
in JavaScript, andpop
in Python.
Checking for Existence of a Key
To check if a key exists, you can use methods likecontainsKey
in Java,has
in JavaScript, and thein
keyword in Python.
Performance Considerations
When using maps, consider the following performance aspects:
Memory Usage: Maps can consume more memory than other data structures due to their dynamic nature and overhead for maintaining key-value pairs.
Collision Handling: In hash maps, collisions can occur when different keys hash to the same index. Most implementations handle this through chaining or open addressing.
Use Cases for Maps
Maps are versatile and can be used in various applications:
Configuration Management: Storing application settings that can be easily accessed and modified.
Data Caching: Implementing caching mechanisms to store frequently accessed data.
Counting and Grouping: Efficiently counting occurrences of items, such as words in a document or votes in an election.
Conclusion
Maps are an essential data structure in programming that facilitate efficient data retrieval and management. Understanding how to implement and utilize maps in different programming languages can greatly enhance your coding capabilities. Whether you're counting frequencies, caching data, or managing configurations, maps provide a robust solution.
For further reading on maps and their applications in programming, you can check out these resources:
By mastering maps, you will be better equipped to handle complex data management tasks in your programming projects.