What is the SVM Algorithm in Machine Learning?
Aug 16, 2024
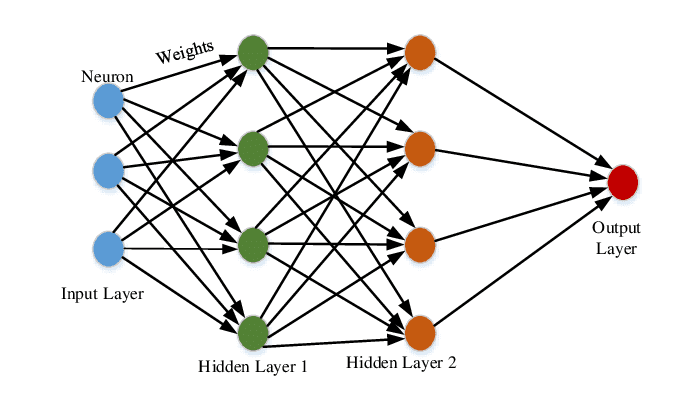
The SVM algorithm in machine learning is a supervised learning model that aims to find the optimal hyperplane that separates data points of different classes in an N-dimensional space. The hyperplane is chosen such that it maximizes the margin between the closest points of the classes, known as support vectors. This characteristic makes SVM particularly effective for high-dimensional data and complex classification tasks.
Key Concepts
Hyperplane
: In the context of SVM, a hyperplane is a decision boundary that separates different classes. For two-dimensional data, it is a line; for three-dimensional data, it is a plane; and for higher dimensions, it becomes increasingly complex.Support Vectors
: These are the data points that are closest to the hyperplane. They are critical in defining the position and orientation of the hyperplane. If the support vectors are removed, the position of the hyperplane can change.Margin: This refers to the distance between the hyperplane and the nearest data point from either class. The SVM algorithm aims to maximize this margin, leading to better generalization on unseen data.
How Does the SVM Algorithm Work?
The SVM algorithm operates through the following steps:
Data Preparation: The first step is to gather and preprocess the data. This may involve normalizing the data, handling missing values, and encoding categorical variables.
Choosing the Kernel: SVM uses kernel functions to transform the data into a higher-dimensional space where it is easier to separate the classes. Common kernels include linear, polynomial, and radial basis function (RBF).
Training the Model: The SVM algorithm is trained using the training dataset. During training, it identifies the support vectors and computes the optimal hyperplane.
Making Predictions: After training, the SVM model can classify new data points based on their position relative to the hyperplane.
Mathematical Formulation
To better understand the SVM algorithm in machine learning, let's look at its mathematical formulation. The goal is to find a hyperplane defined by the equation:
where ww is the weight vector, xx is the input feature vector, and bb is the bias term. The optimization problem can be formulated as:
\minimize \frac{1}{2}||w||^2
subject to the constraints:
y_i(w^Tx_i+b)\geq 1\quad \forall i
Here, y_i is the class label of the data point x_i. The objective is to minimize the norm of the weight vector while ensuring that all data points are classified correctly with a margin of at least 1.
Implementing SVM in Python
To implement the SVM algorithm in machine learning, we can use the popular scikit-learn
library in Python. Below is a step-by-step guide along with code snippets.
Step 1: Import Libraries
Step 2: Load the Dataset
For this example, we will use the Iris dataset, which is commonly used for classification tasks.
Step 3: Split the Data
We will split the dataset into training and testing sets.
Step 4: Train the SVM Model
Now we will create an SVM classifier and fit it to the training data.
Step 5: Make Predictions
After training the model, we can make predictions on the test set.
Step 6: Evaluate the Model
We can evaluate the model's performance using accuracy.
Visualizing the Results
To better understand how the SVM algorithm works, we can visualize the decision boundary created by the SVM model.
Applications of SVM
The SVM algorithm in machine learning is widely used across various fields due to its effectiveness in handling complex datasets. Some notable applications include:
Text Classification: SVMs are used for spam detection, sentiment analysis, and categorizing documents into predefined categories.
Image Recognition: SVMs can classify images based on pixel intensity, making them useful in facial recognition and object detection.
Bioinformatics: SVMs are applied in gene classification and protein structure prediction.
Financial Forecasting: SVMs are used to predict stock prices and assess credit risk.
Advantages of SVM
Effective in High Dimensions: SVMs perform well in high-dimensional spaces, making them suitable for complex datasets.
Robust to Overfitting: With the right choice of kernel and regularization, SVMs are less prone to overfitting, especially in high-dimensional spaces.
Versatile: SVMs can be adapted for both linear and nonlinear classification tasks through the use of different kernels.
Limitations of SVM
Computationally Intensive: Training SVMs can be time-consuming, especially with large datasets.
Choice of Kernel: The performance of SVMs heavily depends on the choice of the kernel and its parameters.
Not Suitable for Noisy Data: SVMs can struggle with datasets that contain a lot of noise or overlapping classes.
Conclusion
The SVM algorithm in machine learning is a powerful tool for classification and regression tasks. Its ability to find the optimal hyperplane that separates classes makes it a popular choice for various applications, from text classification to image recognition. By understanding its workings and implementation, you can leverage SVMs to solve complex problems in your projects.